C code Blocks Write the complete function that receives a pointer to the array's first element as an argument and multiplies the third element of the array by 10. Define your function in the same way as the given function prototype. Take a look at the "For example" below to see how the function is used in the main function and the expected result. #include #include void multiplyElement(int *ptr);int main(){ int array[5] = {1,2,3,4,5}; int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){ printf("%d ", array[i]); } return 0; } //Your answer starts here For example: Test Result int array[5] = {1,2,3,4,5}; int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){ printf("%d ", array[i]); } 1 2 30 4
C code Blocks Write the complete function that receives a pointer to the array's first element as an argument and multiplies the third element of the array by 10. Define your function in the same way as the given function prototype. Take a look at the "For example" below to see how the function is used in the main function and the expected result. #include #include void multiplyElement(int *ptr);int main(){ int array[5] = {1,2,3,4,5}; int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){ printf("%d ", array[i]); } return 0; } //Your answer starts here For example: Test Result int array[5] = {1,2,3,4,5}; int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){ printf("%d ", array[i]); } 1 2 30 4
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C code Blocks
Write the complete function that receives a pointer to the array's first element as an argument and multiplies the third element of the array by 10.
Define your function in the same way as the given function prototype.
Take a look at the "For example" below to see how the function is used in the main function and the expected result.
#include <stdio.h>
#include <stdlib.h>void multiplyElement(int *ptr);int main(){ int array[5] = {1,2,3,4,5};
int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){
printf("%d ", array[i]);
}
return 0;
}
//Your answer starts here
#include <stdlib.h>void multiplyElement(int *ptr);int main(){ int array[5] = {1,2,3,4,5};
int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){
printf("%d ", array[i]);
}
return 0;
}
//Your answer starts here
For example:
Test | Result |
---|---|
int array[5] = {1,2,3,4,5}; int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){ printf("%d ", array[i]); } | 1 2 30 4 |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
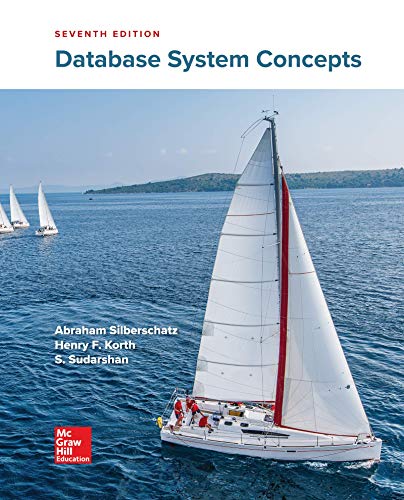
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
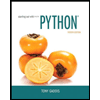
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
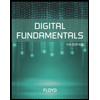
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
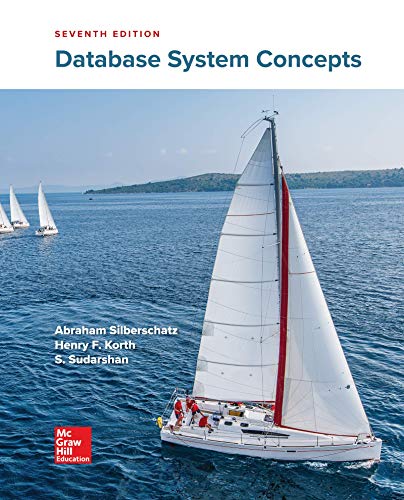
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
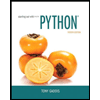
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
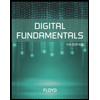
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
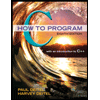
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
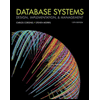
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
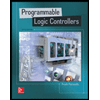
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education