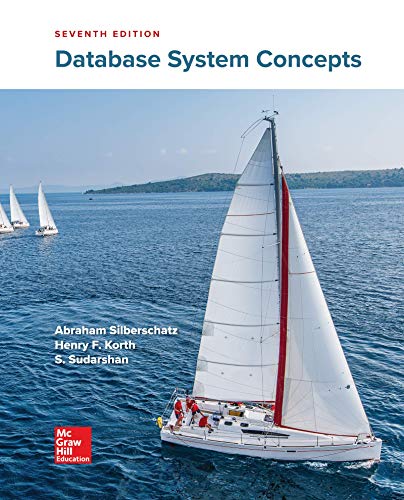
Description: The program prompts the user to enter three numbers and invokes the method: public static void displayIncreasingNumbers(int num1, double num2, long num3) to display them in increasing order.
Below is my code:
package threeincreasingnumbers;
import java.util.Scanner;
public class Threeincreasingnumbers {
public static void main(String[] args) {
// Create Scanner
Scanner scan = new Scanner(System.in);
// Prompt the user to enter three numbers
System.out.print("Enter three numbers: ");
int num1 = scan.nextInt();
double num2 = scan.nextDouble();
long num3 = scan.nextLong();
// Display numbers in increasing order
displayIncreasingNumbers(num1, num2, num3);
}
public static void displayIncreasingNumbers(int num1, double num2, long num3) {
double temp;
if (num2 < num1 && num2 < num3){
temp = num1;
num1 = (int) (double) num2;
num2 = temp;
}
else if (num3 < num1 && num3 < num2) {
temp = num1;
num1 = (int) num3;
num3 = (long) temp;
}
if (num3 < num2) {
temp = num2;
num2 = num3;
num3 = (long) temp;
}
// Display result
System.out.println(num1 + " " + num2 + " " + num3);
}
}
But I get the error when run: Exception in thread "main" java.util.InputMismatchException
at java.util.Scanner.throwFor(Scanner.java:864)
at java.util.Scanner.next(Scanner.java:1485)
at java.util.Scanner.nextInt(Scanner.java:2117)
at java.util.Scanner.nextInt(Scanner.java:2076)
at threeincreasingnumbers.Threeincreasingnumbers.main(Threeincreasingnumbers.java:21)
I assume there is a problem comparing int, double and long numbers. How can I solve this problem?

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- PROBLEM STATEMENT: An anagram is a word that has been rearranged from another word, check tosee if the second word is a rearrangement of the first word. public class AnagramComputation{public static boolean solution(String word1, String word2){// ↓↓↓↓ your code goes here ↓↓↓↓return true;}} Can you help me with this java question the language is java please use the code I gavearrow_forwardMethod Overloading is a feature that allows a class to have two or more methods having same name, if their argument lists are different. Argument lists could differ in: 1. Number of parameters. 2. Data type of parameters. 3. Sequence of Data type of parameters. Write different version of method sum() that display the sum of the values received as parameter according to the following main method content: public static void main(String[] args) { sum ( 10, 10 ); sum ( 10, 10, 10 ); sum ( 10.0, 10.0 ); sum ( 10, 10.0 ); sum ( 10.0, 10); } You have to define five functions with the specified types. Then, demonstrate the Argument Promotion concept by reducing the number of method to two.arrow_forwardExercise 3: The following code asks a user to enter her/his age then determines if a user is an adult or not. Trace the code, to find and solve the syntax errors. You need to identify the errors and rewrite the correct code. import java.util.Scanner; public class Ex3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println(("Enter your age:"); int age = input.nextInt(); double flag = isAdult(double age); if(flag) { System.out.println("You are an adult."); } else { System.out.println("You are not an adult."); } } public boolean isAdult(int age) { if(age > 18) { return true; } else { return false;arrow_forward
- preLabC.java 1 import java.util.Random; 2 import java.util.StringJoiner; 3 4 public class preLabC { 5 6- 7 8 9 10 11 12 13 ~45608192023 Y 17 23 } public static int myMethod(MathVector inputVector) { int valueAt5th Position -100000; // default value for integer System.out.println("here is the contents object, inputVector: + inputVector); // extract the value at the fifth position of the array in the object // Remember: the count starts at 0. So the 5th position has an in } System.out.println("This is the value at position 5: // return the integer value found at position 5 of the return valueAt5thPosition; of 4. + valueAt5thPosition); array.. Description A MathVector object will be passed to your method. Return the 5th element inside of that object. If you look in the file MathVector.java you'll see there is a way to extract the value of one element in the array contained inside a MathVector object: /** * Returns the element at the specified index. * * @param index the index of the…arrow_forwardWhat is causing this error?arrow_forwardHow to fix this error: Error: Main method not found in class ExpatConsultant, please define the main method as: public static void main(String[] args) or a JavaFX application class must extend javafx.application.Application public ExpatConsultant(String dob, String sector, double skillPrice, double taxRate, double airfare) { super(); String[] parts = dob.split("/"); super.setDob(Integer.parseInt(parts[1]), Integer.parseInt(parts[0]), Integer.parseInt(parts[2])); this.skillPrice = skillPrice; this.permitTax = skillPrice * taxRate; this.airfare = airfare; this.sector = sector; this.id = getNextId(); } public String getSector() { return sector; } public String getContact() { return "Reg. Expatriate#" + id; } public int getNextId() { id = nextId; nextId++; return id; } public int getId() { return id; } public double getPay() { double earnings =…arrow_forward
- How do I fix the Java code errors? Code: //import java.util.Arrays;//Movie.java package movie; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; public Movie() { movieName = "Flick"; numMinutes = 0; isKidFriendly = false; numCastMembers = 0; castMembers = new String[10]; } public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = castMembers; } public String getMovieName() { return this.movieName; } public int getNumMinutes() { return this.numMinutes; } public boolean getIsKidFriendly() { return this.isKidFriendly; } public boolean isKidFriendly() {…arrow_forward1. Write a program that takes three numbers (a,b,c) from the user as input and finds the median of the three. The program has a method called median that takes 3 numbers as the parameter and returns the median. Use if-then-else statements to find the median. You can assume the three numbers are not the same. Sample Example: Enter 3 numbers 10 13 The median is 10 public class Median{ public static void main(String[] args){ Scanner input = new int a = int b = int c =arrow_forwardFix all logic and syntax errors. Results Circle statistics area is 498.75924968391547 diameter is 25.2 // Some circle statistics public class DebugFour2 { public static void main(String args[]) { double radius = 12.6; System.out.println("Circle statistics"); double area = java.Math.PI * radius * radius; System.out.println("area is " + area); double diameter = 2 - radius; System.out.println("diameter is + diameter); } }arrow_forward
- Fix all errors to make the code compile and complete. //MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String requiredString =…arrow_forwardComplete the convert() method that casts the parameter from a double to an integer and returns the result.Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 code: public class LabProgram { public static int convert(double d){ /* Type your code here */ } public static void main(String[] args) { System.out.println(convert(19.9)); System.out.println(convert(3.1)); }}arrow_forwardclass Lab4Q1P3 { public static void main(String args[]) { int max = 10;while (max < 10) {System.out.println("count down: " + max);max--;}}} Do you know what is wrong with the program? I know that there is error on the 7th line but I don't know what to fix.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
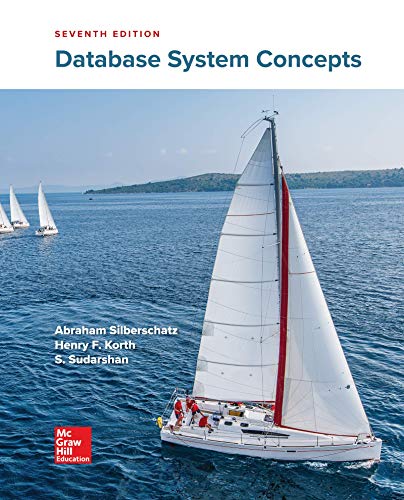
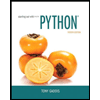
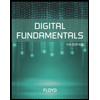
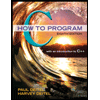
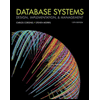
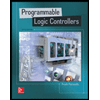