Build two classes (Fraction and FractionCounter) and a Driver for use in counting the number of unique fractions read from a text file. We’ll also reuse the ObjectList class we built in lab to store our list of unique FractionCounters, instead of directly using arrays or the ArrayList. Remember NO DECIMALS! Handle input of any length Introduction Your project is to read in a series of fractions from a text file, which will have each line formatted as follows: “A/B”. A sample text file is listed below, and the purpose of your program is to read in one line at a time and build a Fraction object from A and B. For each unique Fraction seen, your program will create a FractionCounter object used to track the number of occurrences of that specific fraction. When all the input is consumed, your program will print out its ObjectList of unique FractionCounters, which should report the fraction and its count – see output below. You can assume no blank lines or misleading characters; see the text file link above for the some of the input I’ll use when testing your submission. Your program must reduce fractions, as demonstrated in the output below. Sample text file input: 6/3 7/3 6/3 2/1 Sample output: 2/1 has a count of 3 7/3 has a count of 1 Building Multiple Classes Class Fraction This class should be a simple abstraction (i.e., a small class) that represents the ratio of two numbers. There will be only two data elements, and only a few methods. Note that it is required for your Fraction class to store your ratio in reduced form, so this is feature to implement in your software. Data Members The numerator – what primitive type makes the most sense here? The denominator – same type as the numerator. Method Members Overloading Fraction() – default “no-arg” constructor Fraction(int,int) – constructor which initializes your data Overriding boolean equals(Fraction other) – compares “this” to “other” String toString() – replace this inherited method with one appropriate to the class Getters & Setters getNumerator() setNumerator(…) getDenominator() setDenominator(…) Class FractionCounter This class should also be small, and contain only a few state variables. The purpose of this class is to store a reference to a Fraction object and a count of how many times this fraction object has been seen in the input file. Data Members The Fraction – declare this class variable to be of type Fraction from above The counter – the integer value used to count the number of these fractions seen Method Members FractionCounter( Fraction theFraction ) - constructor bool compareAndIncrement( Fraction newFraction ) – used to see if the newFraction passed into this function is the same as the Fraction we’re storing, and if so increments the counter by one and returns true (otherwise, returns false). String toString() – replace this inherited method with one that prints out both the Fraction and its count Error handling When encountering illegal data, print an error message, skip the data, and continue processing Testing and Boundary Cases Before submitting your assignment, be sure to thoroughly test it – for example, if your software fails to compile, the maximum grade falls to a 75%. Does your software reduce fractions? Test this common case. What about boundary cases or unusual situations? For example, does your software read in the fraction “4/0” and crash with a DivideByZeroException? Can your software handle 0 fractions? 1 fraction? 1,000 fractions? What does your software do with the fraction “-1/-4”? What if the numerator or denominator is very large, as in “1/9999”? Does your program report the fraction “1/1 occurs 4 times” only once (as it should, for the fractions.txt file linked in Fractions V1), or does it report “1/1 occurs 4 times” multiple times (which it should not)? If you store the fraction using doubles, does the equals() function suffer from roundoff errors? Test your code for as many cases as you can think of by varying the “fractions.txt” input file. Note that I will try multiple “fractions.txt” input files when grading your assignment. NB: You need to code your program it so it can run with input files besides the example one. In particular, it has to work with input files that are of any length. You must enable the appropriate lists to dynamically resize. OBJECT LIST // pseudo code: when adding element if new count exceeds size { create new larger array copy elements from original array into new array assign new array to array instance variable update maxSize } // Minimal interface for this assignment *** pubic ObjectList() public void add(Object obj) public String toString() // Complete Interface public String toString() public int indexOf(Object obj) public int getNumElements() public Object getAt(int i)
Build two classes (Fraction and FractionCounter) and a Driver for use in counting the number of unique fractions read from a text file. We’ll also reuse the ObjectList class we built in lab to store our list of unique FractionCounters, instead of directly using arrays or the ArrayList.
Remember
- NO DECIMALS!
- Handle input of any length
Introduction
Your project is to read in a series of fractions from a text file, which will have each line formatted as follows: “A/B”. A sample text file is listed below, and the purpose of your program is to read in one line at a time and build a Fraction object from A and B. For each unique Fraction seen, your program will create a FractionCounter object used to track the number of occurrences of that specific fraction. When all the input is consumed, your program will print out its ObjectList of unique FractionCounters, which should report the fraction and its count – see output below. You can assume no blank lines or misleading characters; see the text file link above for the some of the input I’ll use when testing your submission. Your program must reduce fractions, as demonstrated in the output below.
Sample text file input:
6/3
7/3
6/3
2/1
Sample output:
2/1 has a count of 3
7/3 has a count of 1
Building Multiple Classes
Class Fraction
This class should be a simple abstraction (i.e., a small class) that represents the ratio of two numbers. There will be only two data elements, and only a few methods. Note that it is required for your Fraction class to store your ratio in reduced form, so this is feature to implement in your software.
Data Members
- The numerator – what primitive type makes the most sense here?
- The denominator – same type as the numerator.
Method Members
- Overloading
- Fraction() – default “no-arg” constructor
- Fraction(int,int) – constructor which initializes your data
- Overriding
- boolean equals(Fraction other) – compares “this” to “other”
- String toString() – replace this inherited method with one appropriate to the class
- Getters & Setters
- getNumerator()
- setNumerator(…)
- getDenominator()
- setDenominator(…)
Class FractionCounter
This class should also be small, and contain only a few state variables. The purpose of this class is to store a reference to a Fraction object and a count of how many times this fraction object has been seen in the input file.
Data Members
- The Fraction – declare this class variable to be of type Fraction from above
- The counter – the integer value used to count the number of these fractions seen
Method Members
- FractionCounter( Fraction theFraction ) - constructor
- bool compareAndIncrement( Fraction newFraction ) – used to see if the newFraction passed into this function is the same as the Fraction we’re storing, and if so increments the counter by one and returns true (otherwise, returns false).
- String toString() – replace this inherited method with one that prints out both the Fraction and its count
Error handling
- When encountering illegal data, print an error message, skip the data, and continue processing
Testing and Boundary Cases
Before submitting your assignment, be sure to thoroughly test it – for example, if your software fails to compile, the maximum grade falls to a 75%. Does your software reduce fractions? Test this common case. What about boundary cases or unusual situations? For example, does your software read in the fraction “4/0” and crash with a DivideByZeroException? Can your software handle 0 fractions? 1 fraction? 1,000 fractions? What does your software do with the fraction “-1/-4”? What if the numerator or denominator is very large, as in “1/9999”? Does your program report the fraction “1/1 occurs 4 times” only once (as it should, for the fractions.txt file linked in Fractions V1), or does it report “1/1 occurs 4 times” multiple times (which it should not)? If you store the fraction using doubles, does the equals() function suffer from roundoff errors? Test your code for as many cases as you can think of by varying the “fractions.txt” input file. Note that I will try multiple “fractions.txt” input files when grading your assignment.
NB: You need to code your program it so it can run with input files besides the example one. In particular, it has to work with input files that are of any length. You must enable the appropriate lists to dynamically resize.
OBJECT LIST
// pseudo code: when adding element
if new count exceeds size
{
create new larger array
copy elements from original array into new array
assign new array to array instance variable
update maxSize
}
// Minimal interface for this assignment ***
pubic ObjectList()
public void add(Object obj)
public String toString()
// Complete Interface
public String toString()
public int indexOf(Object obj)
public int getNumElements()
public Object getAt(int i)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

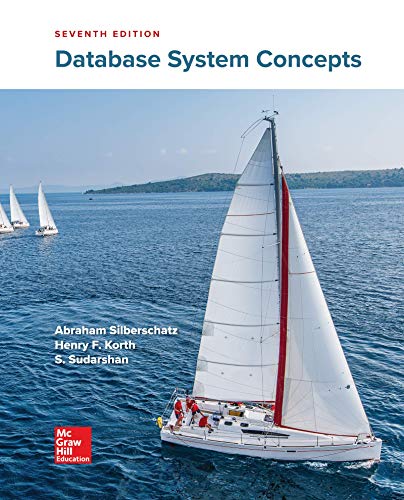
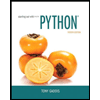
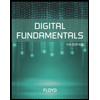
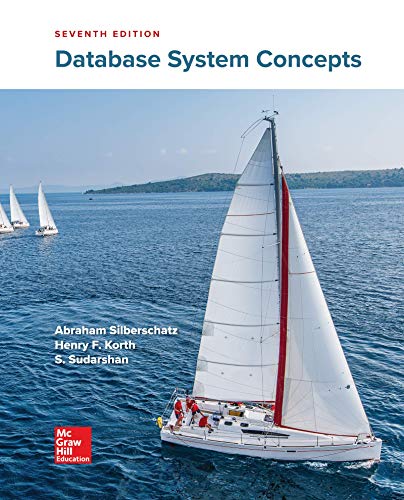
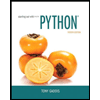
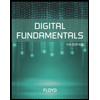
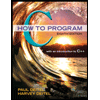
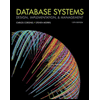
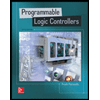