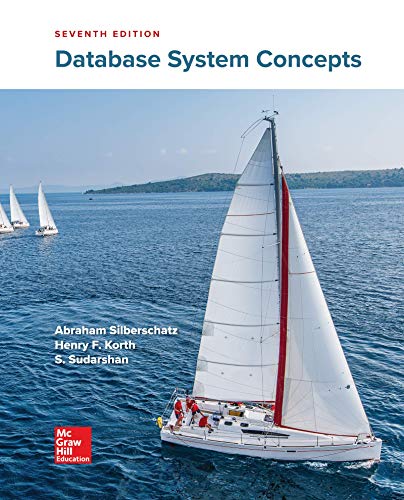
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
23.6 LAB: BankAccount class (Use Python)
Build a class called BankAccount that manages checking and savings accounts. The class has three attributes: a customer name, the customer's savings account balance, and the customer's checking account balance.
Implement the following constructor and instance methods as listed below:
- Constructor with parameters (self, new_name, checking_balance, savings_balance) - set the customer name to parameter new_name, set the checking account balance to parameter checking_balance, and set the savings account balance to parameter savings_balance.
- deposit_checking(self, amount) - add parameter amount to the checking account balance (only if positive)
- deposit_savings(self, amount) - add parameter amount to the savings account balance (only if positive)
- withdraw_checking(self, amount) - subtract parameter amount from the checking account balance (only if positive)
- withdraw_savings(self, amount) - subtract parameter amount from the savings account balance (only if positive)
- transfer_to_savings(self, amount) - subtract parameter amount from the checking account balance and add to the savings account balance (only if positive)
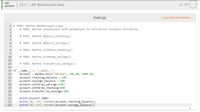
Transcribed Image Text:LAB
23.6.1: LAB: BankAccount class
0/ 10
АCTIVITY
main.py
Load default template...
1 # TODO: Define BankAccount class
2
# TODO: Define constructor with parameters to initialize instance attributes
3
4
# TODO: Define deposit_checking()
5
6
# TODO: Define deposit_savings ()
8
# TODO: Define withdraw_checking()
10
# TODO: Define withdraw_savings ()
11
12
# TODO: Define transfer_to_savings ()
13
14 if
== " main ":
name_
account = BankAccount ("Mickey", 500.00, 1000.00)
account.checking_balance = 500
account.savings_balance = 500
account.withdraw_savings (100)
account.withdraw_checking (10e)
account.transfer_to_savings (300)
15
16
17
18
19
20
21
22
print(account.name)
print('${:.2f}'.format(account.checking_balance))
print("${:.2f}'.format(account.savings_balance))
23
24
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 9.19 LAB: BankAccount class (EO) Build a class called BankAccount that manages checking and savings accounts. The class has three private member fields: a customer name (String), the customer's savings account balance (double), and the customer's checking account balance (double). Implement the following Constructor and instance methods: public BankAccount(String newName, double amt1, double amt2) - set the customer name to parameter newName, set the checking account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount) public void setName(String newName) - set the customer name public String getName() - return the customer name public void setChecking(double amt) - set the checking account balance to parameter amt public double getChecking() - return the checking account balance public void setSavings(double amt) - set the savings account balance to parameter amt public double getSavings() - return the savings account balance…arrow_forwardOnly Number 21arrow_forward7.13 LAB: Vending machine Given two integers as user inputs that represent the number of drinks to buy and the number of bottles to restock, create a VendingMachine object that performs the following operations: Purchases input number of drinks Restocks input number of bottles Reports inventory A VendingMachine's initial inventory is 20 drinks. Ex: If the input is: 5 2 the output is: Inventory: 17 bottles GIVEN: class VendingMachine: def __init__(self): self.bottles = 20 def purchase(self, amount): self.bottles = self.bottles - amount def restock(self, amount): self.bottles = self.bottles + amount def get_inventory(self): return self.bottles def report(self): print('Inventory: {} bottles'.format(self.bottles)) if __name__ == "__main__": # TODO: Create VendingMachine object # TODO: Purchase input number of drinks # TODO: Restock input number of bottles # TODO: Report inventoryarrow_forward
- 5.9 LAB - Database programming with Java (SQLite) Complete the Java program to create a Horse table, insert one row, and display the row. The main program calls four methods: createConnection() creates a connection to the database. createTable() creates the Horse table. insertHorse() inserts one row into Horse. selectAllHorses() outputs all Horse rows. Complete all four methods. Method parameters are described in the template. Do not modify the main program. The Horse table should have five columns, with the following names, data types, constraints, and values: Name Data type Constraints Value Id integer primary key, not null 1 Name text 'Babe' Breed text 'Quarter horse' Height double 15.3 BirthDate text '2015-02-10' The program output should be: All horses: (1, 'Babe', 'Quarter Horse', 15.3, '2015-02-10') This lab uses the SQLite database rather than MySQL. Both SQLite and MySQL Connector/J implement the JDBC API. Consequently, the API is as…arrow_forward1.12 Lab: Check Login Credentials (classes) Complete the Login class implementation. For the class method check credentials(), you will need to accept two arguments - login and passwd. Do not forget about the self argument. This class method will set two local variables simlogin = "Test' and simpass = "test1234". These variables will simulate the correct login and password for the credential check process. The method will receive the login and password from the user and check it with Test and test1234. If the user sent the correct credentials output: Successful login! If the user sent the correct login name but the wrong password output: Login name is correct, incorrect password! If the user sent an incorrect login name but the correct password output: Login name is incorrect, password accepted! If the user sent both incorrect login name and password output: Unsuccessful login attempt! The class method will return a boolean success variable as True if the login was successful. Using if…arrow_forward18.16 Define a method named getWordFrequency that takes an array of strings, the size of the array, and a search word as parameters. Method getWordFrequency() then returns the number of occurrences of the search word in the array parameter (case insensitive). Then, write a main program that reads a list of words into an array, calls method getWordFrequency() repeatedly, and outputs the words in the arrays with their frequencies. The input begins with an integer indicating the number of words that follow. Assume that the list will always contain less than 20 words. Ex: If the input is: 5 hey Hi Mark hi mark the output is: hey 1 Hi 2 Mark 2 hi 2 mark 2 Hint: Use the equalsIgnoreCase() method for comparing strings, ignoring case. The program must define and call a method:public static int getWordFrequency(String[] wordsList, int listSize, String currWord) code: import java.util.Scanner; public class LabProgram { /* Define your method here */ public static void main(String[] args) {…arrow_forward
- 23.4 LAB: Student class (Use Python) In main.py define the Student class that has two attributes: name and gpa Implement the following instance methods: A constructor that sets name to "Louie" and gpa to 1.0 set_name(self, name) - set student's name to parameter name get_name(self) - return student's name set_gpa(self, gpa) - set student's gpa to parameter gpa get_gpa(self) - return student's gpa Ex. If a new Student object is created, the default output is: Louie/1.0 Ex. If the student's name is set to "Felix" and the gpa is set to 3.7, the output becomes: Felix/3.7arrow_forwardInstructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forward7.23 LAB: Product class use Java. Given main(), define the Product class (in file Product.java) that will manage product inventory. Product class has three private member fields: a product code (String), the product's price (double), and the number count of product in inventory (int). Implement the following Constructor and member methods: public Product(String code, double price, int count) - set the member fields using the three parameters public void setCode(String code) - set the product code (i.e. SKU234) to parameter code public String getCode() - return the product code public void setPrice(double p) - set the price to parameter p public double getPrice() - return the price public void setCount(int num) - set the number of items in inventory to parameter num public int getCount() - return the count public void addInventory(int amt) - increase inventory by parameter amt public void sellInventory(int amt) - decrease inventory by parameter amt Ex. If a new Product object is…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
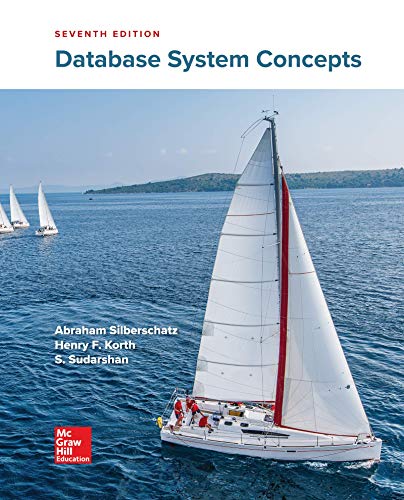
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
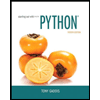
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
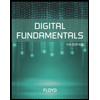
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
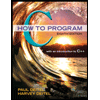
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
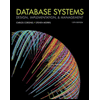
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
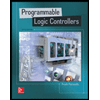
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education