** Below is the image of my code so far, but it still needs work** * This program asks the user to enter an integer from 3 to 9 * and prints a pattern of numbers based on that number. * For example, given the number 4 as input, the output would be: * * 1* * 21* * 321* * 4321* * 321* * 21* * 1* * * Print a newline between the prompt and the beginning of the stars. * Note that no input validation is required.
** Below is the image of my code so far, but it still needs work** * This program asks the user to enter an integer from 3 to 9 * and prints a pattern of numbers based on that number. * For example, given the number 4 as input, the output would be: * * 1* * 21* * 321* * 4321* * 321* * 21* * 1* * * Print a newline between the prompt and the beginning of the stars. * Note that no input validation is required.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
** Below is the image of my code so far, but it still needs work**
* This
* and prints a pattern of numbers based on that number.
* For example, given the number 4 as input, the output would be:
*
* 1*
* 21*
* 321*
* 4321*
* 321*
* 21*
* 1*
*
* Print a newline between the prompt and the beginning of the stars.
* Note that no input validation is required.

Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
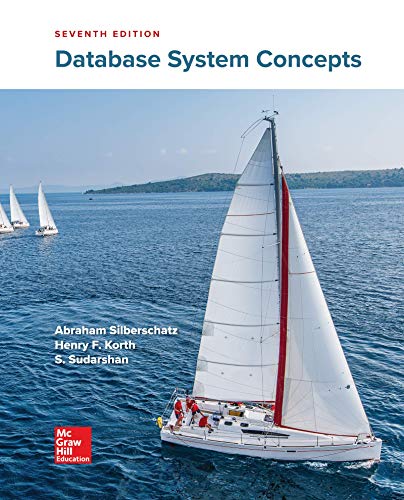
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
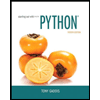
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
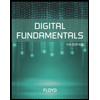
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
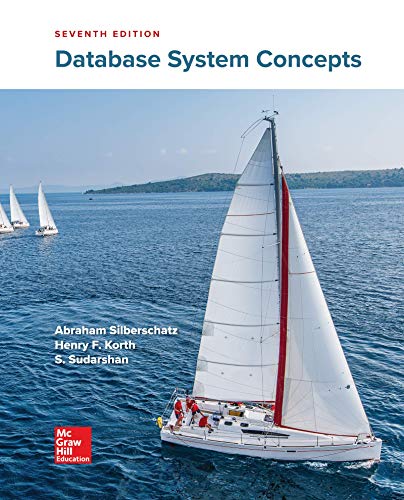
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
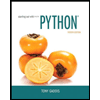
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
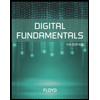
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
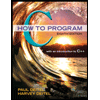
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
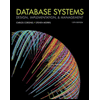
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
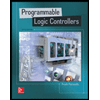
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education