Based on the image attached, draw the flowchart
Based on the image attached, draw the flowchart. (Here I've provided the coding in c language as references)
//header file
#include<stdio.h>
//function prototype
int findHighest (int num[20]);
int findLowest (int num[20]);
int calculateSum(int num[20]);
float calculateAverage(int num[20]);
int main (){
//declare a counter variable for the loop
int i;
//declare variables
int max, min, total, num[20];
float avg;
int choice;
//taking 20 numbers and storing it in array
printf("\n");
printf("\t This Program is to find highest, lowest, total and average of the numbers\n");
printf("\t____________________________________________________________________________________\n");
printf("\n");
printf("\tEnter 20 numbers separated by space : ");
for(i=0;i<20;++i){
scanf("%d",&num[i]);
}
//display menu and take a choice from user
printf("\t------------------------------------------------------------------------------------\n");
printf("\tMenu based program \n\n");
printf("\tChoose what you want to display\n");
printf("\t1: Display highest\n\t2: Display lowest\n\t3: Display total\n\t4: Display average\n");
printf("\t------------------------------------------------------------------------------------\n");
for(i=0;i<4;i++){
printf("\tEnter your choice:");
scanf("%d",&choice);
//use switch case to display the choice
switch (choice)
{
//display highest value if choice = 1
case 1:
//num array is passed to findHighest
max= findHighest(num);
//display the highest number in the array
printf ("\tThe highest value in the array : %d\n", max);
break;
//display lowest value if choice = 2
case 2:
//num array is passed to findLowest
min= findLowest(num);
//display the lowest number in the array
printf ("\tThe lowest value in the array : %d\n", min);
break;
//display total if choice = 3
case 3:
//num array is passed to calculateSum
total =calculateSum(num);
//display the sum of the array elements
printf ("\tTotal of the array elements = %d\n", total);
break;
//display average if choice = 4
case 4:
//num array is passed to calculateAverage
avg = calculateAverage(num);
printf("\tAverage: %.2f\n", avg);
break;
//operator doesn't match any case constant 1, 2, 3, 4
default:
printf("\tWRONG INPUT\n");
}
printf("\t*****************************************\n");
}
return 0;//return zero
}
//function definition
int findHighest(int num[20]){
//declare a variable to hold the highest value
int highest;
//declare a counter variable for the loop
int i;
//consider the first element as highest
highest = num[0];
//use loop to
//step through the rest of the array,
//beginning at the element 1.
//when a value is greater than highest is found,
//assign that value to the highest.
//when the loop finishes,the highest
//variable will contain the highest value
//in the array.
for (i=0;i<20;i++){
if (num[i]>highest){
highest=num[i];
}
}
return highest; //return value
}
int findLowest(int num[20]){
//declare a variable to hold the lowest value
int lowest;
//declare a counter variable for the loop
int i;
//consider the first element as lowest
lowest = num[0];
//use loop to
//step through the rest of the array,
//beginning at the element 1.
//when a value is less than lowest is found,
//assign that value to the lowest.
//when the loop finishes,the lowest
//variable will contain the lowest value
//in the array.
for (i=0;i<20;i++){
if (num[i]<lowest){
lowest=num[i];
}
}
return lowest; //return value
}
int calculateSum (int num[]){
//declare and initialized an accumalator variable
int sum=0;
//declare a counter variable for the loop
int i;
//use loop to
//step through the rest of the array,
//adding the value of each array element to the accumulator.
for (i=0;i<20;++i){
sum += num[i];
}
return sum; //return value
}
float calculateAverage(int num[]) {
//declare a counter variable for the loop
int i;
//declare variables to hold the average
float avg;
//declare and initialize an accumulator variable
int sum = 0;
// calculate total
for (i = 0; i < 20; i++) {
sum += num[i];
}
// calculate average
avg = sum / 20;
// return average
return avg;
}


Step by step
Solved in 2 steps with 2 images

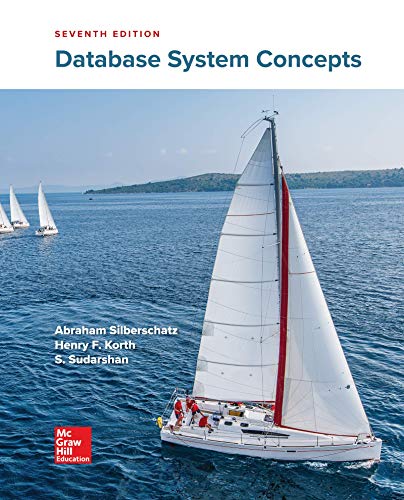
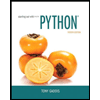
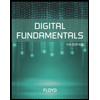
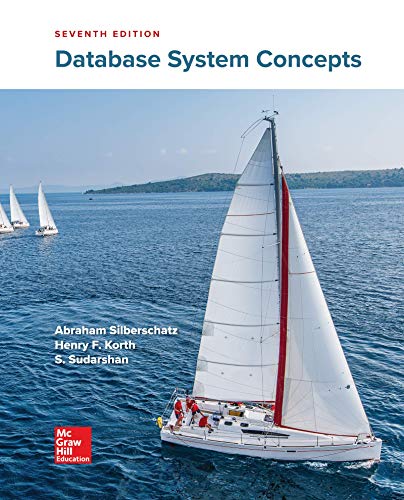
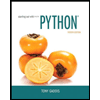
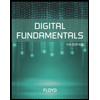
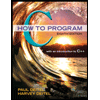
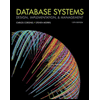
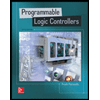