Assume you are a developer working on a class as part of a software package for running artificial neural networks. The network is made of Nodes (objects that implement a Node interface) and Connections (objects that implement a Connection interface). To create a new connection you call the constructor for a concrete class that implements the Connection interface, passing it three parameters: Node origin, Node target, and double weight. The weight is a double that is greater than or equal to 0, and represents how strongly the target node should weigh the input from the origin node. So with a weight of 0.1 the target node will only slightly weight the input from the origin, but with a weight of 725.67 the target node will strongly weight the input. The method throws an IllegalArgumentException. Which of the following are true? A. All of the above are true. B. The code: Connection c = new ConvLayerConnection(origin, target, 100000.0); will throw an IllegalArgumentException because the input is too large. C. The code: Connection c = new ConvLayerConnection(origin,target, 0); will create a new connection with 0 weight, meaning the target will ignore the input from this layer. D. The code: Connection c = new ConvLayerConnect(origin, target, -0.1); will throw an IllegalArgumentException. E. The code: Connection c = new ConvLayerConnection(origin, target, -5); Will create a new connection with a negative weight, meaning the target node will do the opposite of the input from the origin node.
Please help answer this Java multiple choice question.
Assume you are a developer working on a class as part of a software package for running artificial neural networks. The network is made of Nodes (objects that implement a Node interface) and Connections (objects that implement a Connection interface). To create a new connection you call the constructor for a concrete class that implements the Connection interface, passing it three parameters: Node origin, Node target, and double weight. The weight is a double that is greater than or equal to 0, and represents how strongly the target node should weigh the input from the origin node. So with a weight of 0.1 the target node will only slightly weight the input from the origin, but with a weight of 725.67 the target node will strongly weight the input. The method throws an IllegalArgumentException. Which of the following are true?
Connection c = new ConvLayerConnection(origin, target, 100000.0);
will throw an IllegalArgumentException because the input is too large.
Connection c = new ConvLayerConnection(origin,target, 0);
will create a new connection with 0 weight, meaning the target will ignore the input from this layer.
Connection c = new ConvLayerConnect(origin, target, -0.1);
will throw an IllegalArgumentException.
Connection c = new ConvLayerConnection(origin, target, -5);
Will create a new connection with a negative weight, meaning the target node will do the opposite of the input from the origin node.

Step by step
Solved in 3 steps

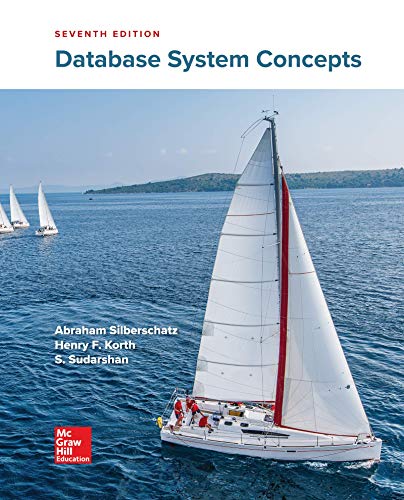
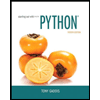
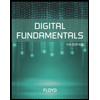
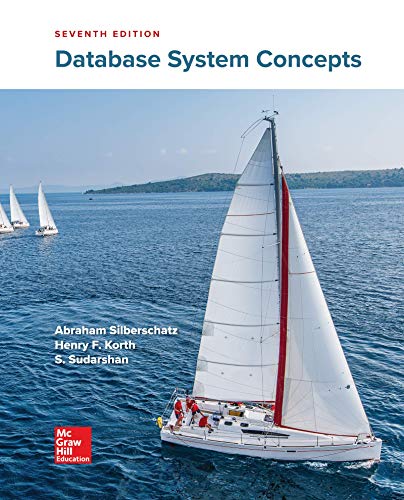
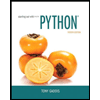
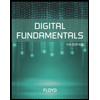
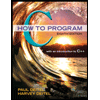
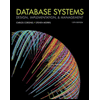
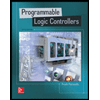