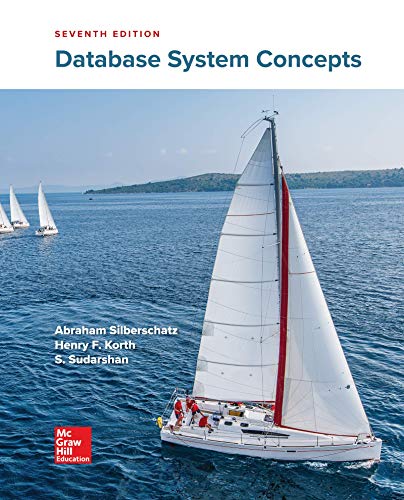
Concept explainers
Assignment #3: Unix shell with redirects and pipes
The purpose of this assignment is to learn to develop multi-process programs. You are expected to extend the myshell.c
-
> - Redirect standard output from a command to a file. Note: if the file already exists, it will be erased and overwritten without warning. For example,
COP4338$ ls > 1
COP4338$ sort myshell.c > 2Note that you're not supposed to implement the Unix commands (ls, sort, ...). You do need to implement the shell that invoke these commands and you need to "wire" up the standard input and output so that they "chain" up as expected.
-
>> - Append standard output from a command to a file if the file exists; if the file does not exist, create one. For example,
COP4338$ sort myshell.c >> 1
COP4338$ echo "Add A Line" >> 1 -
< - Redirect the standard input to be from a file, rather than the keyboard. For example,
COP4338$ sort < myshell.c
COP4338$ sort < myshell.c > 1
COP4338$ sort > 1 < myshell.cThe second and third commands are the same: the sort program reads the file named myshell.c as standard input, sorts it, and then writes to file named 1.
- | - Pass the standard output of one command to another for further processing.
For example,
COP4338$ ls | sort
COP4338$ sort < myshell.c | grep main | cat > output
Make sure you have your parent process fork the children and 'wire' them up using pipes accordingly. There will be spaces separating tokens in the command line. Your program should be able to run with all the Unix commands as expected. Don't assume your program will only be tested by the above commands. Also, there could be more than two commands chained by pipes, like the second example above.
Your program needs to provide necessary sanity-check from the user input. Prompt meaningful errors accordingly as what you'd experience with your shell.
Hint: use strtok() functions to separate the tokens and use strstr() to locate sub-strings.
Submit a zip file FirstnameLastnameA3.zip (of course, you'd use your real name here). You should place the source code and the Makefile in the directory. One should be able to create the executable called myshell by simply 'make'. The Makefile should also contain a 'clean' target for cleaning up the directory (removing all temporary files, object files and executable files). Make sure you don't include intermediate files: *.o, executables, *~, etc., in your submission. (There'll be a penalty for including unnecessary intermediate files).
Please make sure you submit homework before the deadline. Extensions cannot be given for the final program.
If the program does not compile and do something useful when it runs it will not earn any credit.

Trending nowThis is a popular solution!
Step by stepSolved in 9 steps with 7 images

- In the C language, in terms of the Windows API, develop a program that performs the following actions: 1. creates a new process using the CreateProcess function 2. is waiting for this process to complete 3. displays "Bye" 4. shuts down. In the process created by the program, include a wait of 5 seconds and then the output "Hello!".arrow_forwardFor this Assignment you are going to create a script that prints "Hello world" to the PowerShell window. It is recommended that you watch all the lectures in the Module 7 section to complete this assignment. While you have run many cmdlet's individually, as some of you may know, the first script or program typically created in a new language is Hello World. To keep the tradition, lets make a ps1 version. For this assignment, create a script file called Helloworld_FirstName_LaseName.ps1. That script file when run, should write "Hello World" to the Powershell Window.arrow_forwardSuppose a library is processing an input file containing the titlesof books in order to remove duplicates. Write a program thatreads all of the titles from an input file called bookTitles.txtand writes them to an output file called noDuplicates.txt.When complete, the output file should contain all unique titlesfound in the input file.arrow_forward
- Suppose a library is processing an input file containing the titlesof books in order to remove duplicates. Write a program thatreads all of the titles from an input file called bookTitles.txtand writes them to an output file called noDuplicates.txt.When complete, the output file should contain all unique titlesfound in the input file.arrow_forwardWrite a program in C that creates an external file "mylnfo.txt" in the same directory in which the program exists. Then write your name and surname in the first line, your student number in the second line, and your final exam grade in the third line of the newly created text file "mylnfo.txt". Make sure to open and close the file stream at the appropriate steps of your program. Bonus: Modify the program in a way so that before creating the textfile, the program checks whether such a file exists or not and prompts the user if they want to continue and overwrite it if there exists such a file (add a part to the beginning of your program)arrow_forwardThese are utilizing Linux utilities/system callsarrow_forward
- Write an address book program that stores your contacts' names and their email addresses. The names and email addresses are originally stored in a file called phonebook.in, in the format: Harry Potter t..d@hogwarts.edu Hermione Granger b..h@hogwarts.edu Ron Weasley r..b@hogwarts.edu Draco Malfoy m..s@hogwarts.edu Severus Snape h..e@hogwarts.edu Albus Dumbledore a..x@hogwarts.edu Your program should read from the file, storing the names and corresponding email addresses in a dictionary as key-value pairs. Then, the program should display a menu that lets the user enter the numbers 1 through 5, each corresponding to a different menu item: 1) look up an email address 2) add a new name and email address 3) change an email address 4) delete a name and email address 5) save address book and exit When the user enters 1, the program should prompt them for a name, and then print the corresponding email address. If there is no dictionary entry under that name, the program should print, "Sorry,…arrow_forwardwrite c programming in raspberry pi # Write the command to copy the information from info.txt to the text files in each folder test01 to test10 # start i=1 while i<=10 do (i++) cat info.txt >> ./test$i/test$i done #end Does the script run as expected and without errors?arrow_forwardC Program: Write a program that: As its input arguments, accepts a program name followed by any number of command line arguments for that program. Creates a child process that executes the given program, passing all the provided arguments to it. Once the execution of the child process finishes, the parent process receives the child process exit code (X) and prints Exit code was X to the standard error device. If you run ./myexec echo this is just a test, it should produce "this is just a test" on the standard output and "Exit code was 0" on the standard error device. Please show the output as well and make sure there is no errors in the C program codearrow_forward
- On a UNIX-like operating system, a file named "exam.pdf" is owned by user "john". In the file listing, the permissions of this file are given as -rwxrw-r-- What kind of access does user "john" have with respect to this file? Read, Write, and Delete Read, Write, and Execute Read and Write only Read onlyarrow_forwardWhy should an exception be thrown throughout the process?arrow_forwardIf wrong answer this time will downvote it Create a class with a static main that tests the ability to resolve and print a Path: • Create an instance of a FileSystem class. • Resolve an instance of a Path interface from a directory path and filename. • Print the constructed Path with System.out.println() method. 2. Create a class with a static main that tests the ability to resolve and print a Path: • Create an array of Path class. • Instantiate instances of Path with absolute and relative paths. • Print the constructed elements of the array of Path class with System.out.println() method. 3. Create a class to test serialisation class that implements serializable, it should implement the following: • A static void method that serialises an object. • A static void method that deserializes an object. • A static main method that tests the two by moving an object from one to the otherarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
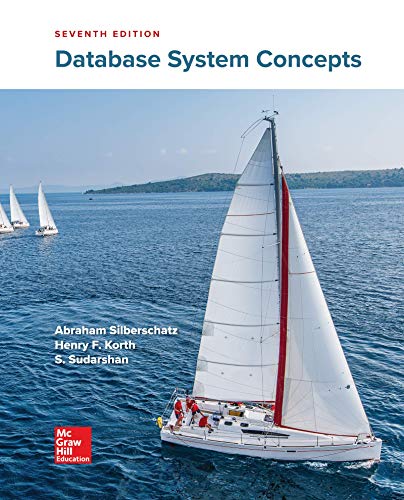
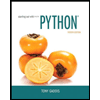
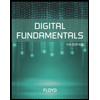
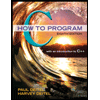
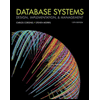
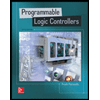