Assign variable maximumVal with the largest value of 9 positive floating-point values read from input. Ex: If the input is 7.8 11.1 7.7 5.9 19.5 3.5 3.4 3.7 12.1, then the output is: 19.5 Note: • The first value read is the largest value seen so far. • All floating-point values are of type double. 1 import java.util.Scanner; 3 public class MaximumValues { 1234567 7 8 9 10 11 12 13 } D public static void main(String[] args) { Scanner scnr = new Scanner(System.in); double inval; double maximumVal = 0.0; int i; /* Your code goes here */ System.out.println(maximumVal); 14}
Assign variable maximumVal with the largest value of 9 positive floating-point values read from input. Ex: If the input is 7.8 11.1 7.7 5.9 19.5 3.5 3.4 3.7 12.1, then the output is: 19.5 Note: • The first value read is the largest value seen so far. • All floating-point values are of type double. 1 import java.util.Scanner; 3 public class MaximumValues { 1234567 7 8 9 10 11 12 13 } D public static void main(String[] args) { Scanner scnr = new Scanner(System.in); double inval; double maximumVal = 0.0; int i; /* Your code goes here */ System.out.println(maximumVal); 14}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![## Understanding Maximum Value in Java
In this tutorial, we will learn how to find the largest value among a series of floating-point numbers using Java.
### Problem Statement
You need to assign the variable `maximumVal` to the largest value among 9 positive floating-point numbers that are read from input.
**Example**:
If the input is `7.8, 11.1, 7.7, 5.9, 19.5, 3.5, 3.4, 3.7, 2.1`, then the output should be:
```
19.5
```
### Key Points:
- **Initial Value**: Start by assuming the first value read is the largest.
- **Data Type**: All the floating-point numbers are of type `double`.
### Java Code Structure
```java
import java.util.Scanner;
public class MaximumValues {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
double inVal;
double maximumVal = 0.0;
int i;
/* Your code goes here */
System.out.println(maximumVal);
}
}
```
### Explanation:
- **Libraries**: Import the `java.util.Scanner` library to read input values.
- **Variable Initialization**: `double inVal` is for storing each input value, and `double maximumVal` is initialized to `0.0` to hold the maximum value found.
- **Code Logic**: Within the comment section `/* Your code goes here */`, you'll write code to read the input values and update `maximumVal` if a larger value is found.
- **Output**: Finally, print the largest value using `System.out.println(maximumVal);`.
By implementing this logic, you can efficiently determine the maximum of the given floating-point numbers.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9e6fa127-a003-469d-9a51-b1d4610918ed%2Fe82d5362-4fd8-40e6-9b31-5494fc0c1417%2F2e1w1lc_processed.png&w=3840&q=75)
Transcribed Image Text:## Understanding Maximum Value in Java
In this tutorial, we will learn how to find the largest value among a series of floating-point numbers using Java.
### Problem Statement
You need to assign the variable `maximumVal` to the largest value among 9 positive floating-point numbers that are read from input.
**Example**:
If the input is `7.8, 11.1, 7.7, 5.9, 19.5, 3.5, 3.4, 3.7, 2.1`, then the output should be:
```
19.5
```
### Key Points:
- **Initial Value**: Start by assuming the first value read is the largest.
- **Data Type**: All the floating-point numbers are of type `double`.
### Java Code Structure
```java
import java.util.Scanner;
public class MaximumValues {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
double inVal;
double maximumVal = 0.0;
int i;
/* Your code goes here */
System.out.println(maximumVal);
}
}
```
### Explanation:
- **Libraries**: Import the `java.util.Scanner` library to read input values.
- **Variable Initialization**: `double inVal` is for storing each input value, and `double maximumVal` is initialized to `0.0` to hold the maximum value found.
- **Code Logic**: Within the comment section `/* Your code goes here */`, you'll write code to read the input values and update `maximumVal` if a larger value is found.
- **Output**: Finally, print the largest value using `System.out.println(maximumVal);`.
By implementing this logic, you can efficiently determine the maximum of the given floating-point numbers.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
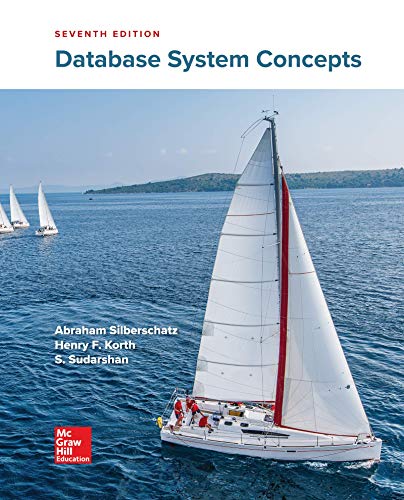
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
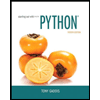
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
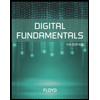
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
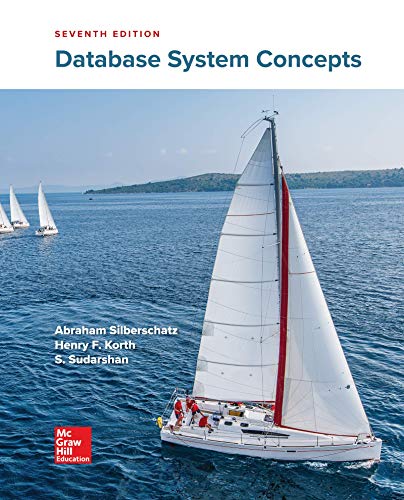
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
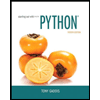
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
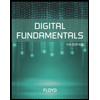
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
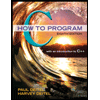
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
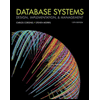
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
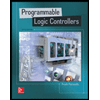
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education