Ask the user to enter three numbers. Prints the three numbers in order. *USING ONLY IF/ELSE IF STATEMENTS
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Ask the user to enter three numbers. Prints the three numbers in order.
*USING ONLY IF/ELSE IF STATEMENTS
![### Java Program to Order Three Numbers
Here is a Java program that takes three integers as input and prints them in ascending order.
#### Code Breakdown
```java
import java.util.Scanner;
public class InOrderThree
{
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Please enter three numbers: ");
int a = in.nextInt();
int b = in.nextInt();
int c = in.nextInt();
// Complete the program
if ( a < b && a < c && b < c) {
System.out.print("Ordered: " + a + b + c);
}
else if (a < b && a < c && c < b) {
System.out.print("Ordered: " + a + c + b);
}
else if (b < a && b < c && a < c) {
System.out.print("Ordered: " + b + a + c);
}
else if(b < a && b < c && c < a) {
System.out.print("Ordered: " + b + c + a);
}
else if (c < a && c < b && a < b) {
System.out.print("Ordered: " + c + a + b);
}
else {
System.out.print("Ordered: " + c + b + a);
}
}
}
```
### Explanation
1. **Import Statement**:
- `import java.util.Scanner;`: This statement imports the `Scanner` class, which is used to get user input.
2. **Class Declaration**:
- `public class InOrderThree`: Declares a public class named `InOrderThree`.
3. **Main Method**:
- `public static void main(String[] args)`: This is the entry point of the program where the execution starts.
4. **Scanner Initialization**:
- `Scanner in = new Scanner(System.in);`: Creates a `Scanner` object to take input from the user.
5. **User Input**:
- `System.out.print("Please enter three numbers: ");`: Prompts the user to enter three integers.
- `int a = in.nextInt();`, `int b = in.nextInt();`, `int c = in.nextInt();`: Reads integer inputs from the user and stores them in variables `a`,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd772767f-fd91-4ca5-b737-b5766e3fef0a%2Fc4f9cef8-0500-40dc-af86-fd0506eb04fb%2Fe6g563q.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

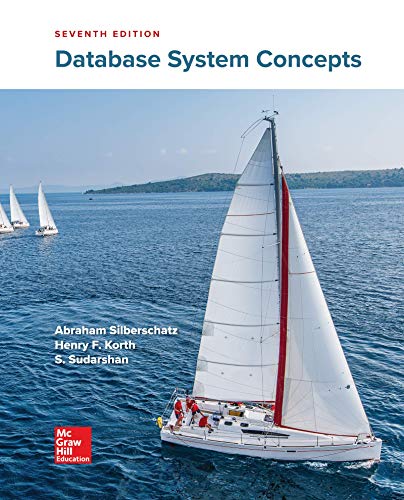
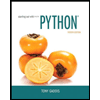
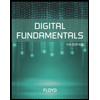
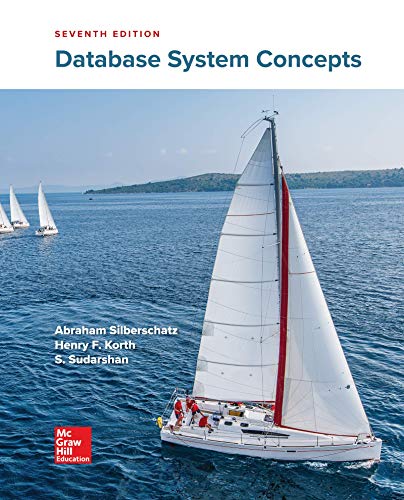
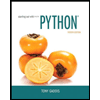
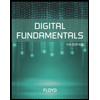
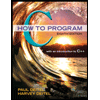
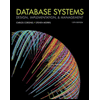
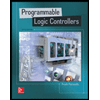