After every word on a line is spellchecked, it should be written to an output file called checked.txt. The arrangement of words on lines should be the same in the document and in checked.txt. The difference between the document and checked.txt is that in checked.txt each word is in lowercase and there is no punctuation. Also, some words might be changed by the spellcheck process. For example, suppose the document contains the following: The Hunter Moon waxed round in the night sky, and put to flight all the lesser stars. But low in the South one star shone red. Then checked.txt will contain: the hunter moon waxed round in the night sky and put to flight all the lesser stars but low in the south one star shone red (In a real spellchecker, the output will be in the same case as the original, and all punctuation will be retained. For simplicity, we will not worry about case and punctuation.) How to Process Words Which Are Not in the Dictionary The spellcheck method also needs to handle the processing for words that are not found. If a word is not found in the dictionary, the program will print a message indicating that the word was not found. Then it will ask the user whether to add the word to the dictionary, whether the word should be changed, or whether processing should continue with no change. yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 3 [yikes will remain and the dictionary is unchanged] If user chooses to change the word, then the user will be prompted for the new word and the new word will replace the original word. If the new word is not in the dictionary, it will be added to the dictionary. For example, yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 1 enter word to replace yikes ==> yippee [yippee will appear instead of yikes yippee will be added to the dictionary if it is not already there] If the user chooses to add the word to the dictionary, the word is added to the dictionary and it is not changed. yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 2 yikes added to dictionary [yikes will remain] If the user chooses to continue, no change is made. The word is unchanged and the dictionary is unchanged. In the first case, yikes will be replaced by yippee in the output file checked.txt. In the second case yikes will appear in checked.txt and it will be added to the dictionary. In the third case yikes will appear in checked.txt but will not be added to the dictionary. Do not write one giant method to do all the spellchecking! Create some private methods that the spellcheck method will call to do parts of the processing. Client Code The main method will prompt for the name of the file containing the dictionary and the name of the input file to be spellchecked. Create the Scanner for the dictionary file and pass it to the Dictionary constructor. Create the Scanner for the input file, perform the spellcheck, and write out the updated dictionary to the file newDict.txt. Use exception handling in the main method to ensure the input files were found and opened properly. If not handle the situation by prompting the user again for the correct file name. Do not use any set or get methods. Hints Write your Dictionary class first. Create a driver program to test the Dictionary. Create a file that has only a few words (say 25) to use when testing your Dictionary class. Write your client code next. Write your Spellcheck class next. Start with a spellCheck method that doesn't do any spellchecking. Write the code to use split and the other String methods to separate and clean up the words and then write them to checked.txt. Write the code to implement option 3: if a word is not in the dictionary, don't change the word and don't change the dictionary. Test it with your small dictionary file. Add the code for option 2: if a word is not in the dictionary, add the word to the dictionary. Test it with your small dictionary file so that it's easy to check whether the word is added to the dictionary. Start with just one word that's not in the dictionary, then test with more than one, including more than one on the same line. Lastly, add the code for option 1: if a word is not in the dictionary, prompt for a replacement word, add the replacement word to the dictionary and change the original word to the replacement word in the input. Again, test it with your small dictionary file so that it's easy to check whether the word is added to the dictionary. Also make sure that the word is replaced in checked.txt. Test with the full dictionary. Lab Standards No global variables. Ever. When prompting a user in a loop, tell him/her what to enter to get out of the loop. Don't give an error message after the user enters a code that should exit from a loop. Use a priming read to avoid this. Use meaningful variable names. Names like i, j, or k should only be used for loop counters. Don't write infinite loops with a break inside
After every word on a line is spellchecked, it should be written to an output file called checked.txt. The arrangement of words on lines should be the same in the document and in checked.txt. The difference between the document and checked.txt is that in checked.txt each word is in lowercase and there is no punctuation. Also, some words might be changed by the spellcheck process. For example, suppose the document contains the following:
The Hunter Moon waxed round in the night sky, and put to flight all the lesser stars. But low in the South one star shone red.
Then checked.txt will contain:
the hunter moon waxed round in the night sky and put to flight all the lesser stars but low in the south one star shone red
(In a real spellchecker, the output will be in the same case as the original, and all punctuation will be retained. For simplicity, we will not worry about case and punctuation.)
How to Process Words Which Are Not in the Dictionary
The spellcheck method also needs to handle the processing for words that are not found.
If a word is not found in the dictionary, the program will print a message indicating that the word was not found. Then it will ask the user whether to add the word to the dictionary, whether the word should be changed, or whether processing should continue with no change.
yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 3 [yikes will remain and the dictionary is unchanged]
- If user chooses to change the word, then the user will be prompted for the new word and the new word will replace the original word. If the new word is not in the dictionary, it will be added to the dictionary. For example, yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 1 enter word to replace yikes ==> yippee [yippee will appear instead of yikes yippee will be added to the dictionary if it is not already there]
- If the user chooses to add the word to the dictionary, the word is added to the dictionary and it is not changed. yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 2 yikes added to dictionary [yikes will remain]
- If the user chooses to continue, no change is made. The word is unchanged and the dictionary is unchanged.
In the first case, yikes will be replaced by yippee in the output file checked.txt. In the second case yikes will appear in checked.txt and it will be added to the dictionary. In the third case yikes will appear in checked.txt but will not be added to the dictionary.
Do not write one giant method to do all the spellchecking! Create some private methods that the spellcheck method will call to do parts of the processing.
Client Code
The main method will prompt for the name of the file containing the dictionary and the name of the input file to be spellchecked. Create the Scanner for the dictionary file and pass it to the Dictionary constructor. Create the Scanner for the input file, perform the spellcheck, and write out the updated dictionary to the file newDict.txt.
Use exception handling in the main method to ensure the input files were found and opened properly. If not handle the situation by prompting the user again for the correct file name.
Do not use any set or get methods.
Hints
- Write your Dictionary class first. Create a driver program to test the Dictionary. Create a file that has only a few words (say 25) to use when testing your Dictionary class.
- Write your client code next.
- Write your Spellcheck class next. Start with a spellCheck method that doesn't do any spellchecking. Write the code to use split and the other String methods to separate and clean up the words and then write them to checked.txt.
- Write the code to implement option 3: if a word is not in the dictionary, don't change the word and don't change the dictionary. Test it with your small dictionary file.
- Add the code for option 2: if a word is not in the dictionary, add the word to the dictionary. Test it with your small dictionary file so that it's easy to check whether the word is added to the dictionary. Start with just one word that's not in the dictionary, then test with more than one, including more than one on the same line.
- Lastly, add the code for option 1: if a word is not in the dictionary, prompt for a replacement word, add the replacement word to the dictionary and change the original word to the replacement word in the input. Again, test it with your small dictionary file so that it's easy to check whether the word is added to the dictionary. Also make sure that the word is replaced in checked.txt.
- Test with the full dictionary.
Lab Standards
- No global variables. Ever.
- When prompting a user in a loop, tell him/her what to enter to get out of the loop.
- Don't give an error message after the user enters a code that should exit from a loop. Use a priming read to avoid this.
- Use meaningful variable names. Names like i, j, or k should only be used for loop counters.
- Don't write infinite loops with a break inside

![The split method (String[] split(String regex)) separates the items (for us these are the words; in split they
are called "tokens") in a String and stores them in an array of Strings. In general, tokens can be separated
by whitespace, commas, slashes, or any other character(s). The parm to split specifies how to separate the
tokens. In our input the tokens are words and they are separated by one or more whitespace characters.
The value we will use to specify how to separate is "\\s+". Here is an explanation of "\\s+": '\s' represents
any whitespace character. The extra \ indicates that '\s' represents one character, not the character '\'
followed by the character 's'. The + means "one or more", so all together "\\s+" means "one or more
whitespace character". When we call split with this parm, it returns an array of Strings where each element
of the array is one word of this.
For example, if we have a String inputLine which contains the String
All
that is
GOLD, does not
glitter!
After the call
String[] words =
inputLine.split("\\s+");
the
array
words will contain
words[0]
"All"
words[1]
"that"
words[2]
"is"
words[3]
"GOLD,"
words[4]
"does"
words[5]
"not"
words[6]
"glitter!"
Cleaning up words before spellchecking: Once the input line is split, then each word mut be cleaned up
before it can be looked up in the Dictionary: it must be changed to lowercase and any punctuation at the
end of the word must be removed. There are String methods you can use for this. One String method you
will use is the one that returns a lowercase version of a String. You can use other String and Character
methods to check whether the last character of a word is a letter, and if not (if it's a punctuation mark like a
comma or a period), create a String with that character removed. Look up the Java String class to find these
methods.
If the word is found in the Dictionary, then it is spelled correctly. If the word is not in the Dictionary, then the
user has some options. These are described below.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcdafc640-2a59-4881-b26f-9da9a278f06e%2F668e69f7-b85d-4bb6-ad39-a7acea52e629%2Fttwxd4c_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

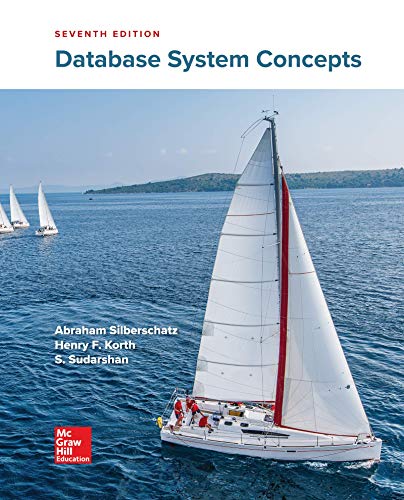
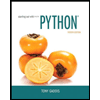
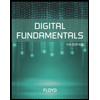
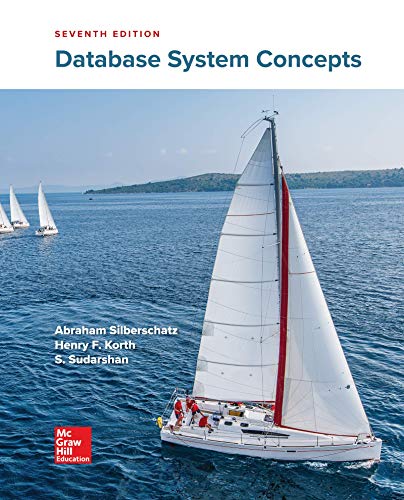
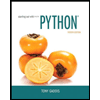
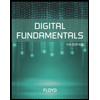
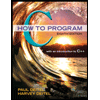
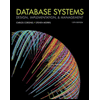
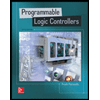