a. Define a class named Inventory. a. Define a member function named_init_ to meet the following requirements: a. Take a self object as a positional parameter. b. Take a filename string as a keyword parameter. c. Define a self.data instance variable to hold the dictionary. d. Open the filename JSON file, if it exists and contains data, assign the JSON deserialized data (a dictionary) to self.data, otherwise cleanly handle the error.
a. Define a class named Inventory. a. Define a member function named_init_ to meet the following requirements: a. Take a self object as a positional parameter. b. Take a filename string as a keyword parameter. c. Define a self.data instance variable to hold the dictionary. d. Open the filename JSON file, if it exists and contains data, assign the JSON deserialized data (a dictionary) to self.data, otherwise cleanly handle the error.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:# Inventory Management System: Class Design
## Overview
This guide outlines how to implement an inventory management system by creating a Python file named `inventory.py`. This program will manage a collection of inventory items using a class named `Inventory` with several key functionalities.
## Class Definition: `Inventory`
### Initialization
- **Function: `__init__`**
- **Parameters:**
- `self`: The instance of the class.
- `filename`: A string representing the name of the JSON file.
- **Actions:**
- Define `self.data` as an instance variable to store the inventory dictionary.
- Load data from the JSON file, if available, and assign it to `self.data`.
- If the file doesn't exist or there's an error, handle it gracefully.
### Adding Items
- **Function: `add_item`**
- **Parameters:**
- `self`: The class instance.
- `barcode`: A string used as a key in the dictionary.
- `description`: A brief description of the item.
- **Actions:**
- Check the format of `barcode`; return 109 if the format is incorrect.
- Return 101 if the `barcode` already exists.
- Add the item to the dictionary with a quantity of zero.
- Save the updated dictionary to the JSON file.
- Return 100 for a successful addition.
### Modifying Item Descriptions
- **Function: `modify_description`**
- **Parameters:**
- `self`: The class instance.
- `barcode`: The item's barcode.
- `description`: The new description for the item.
- **Actions:**
- Return 102 if the `barcode` is not found.
- Update the item's description.
- Save the changes to the JSON file.
- Return 100 for a successful update.
### Adding Quantity
- **Function: `add_qty`**
- **Parameters:**
- `self`: The class instance.
- `barcode`: The item's barcode.
- `qty`: The quantity to add.
- **Actions:**
- Return 102 if the `barcode` doesn't exist.
- Validate the `qty`; return 108 if invalid.
- Update the quantity and save changes.
- Return 100 for a successful addition.
### Removing Quantity
- **Function: `remove_qty`
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
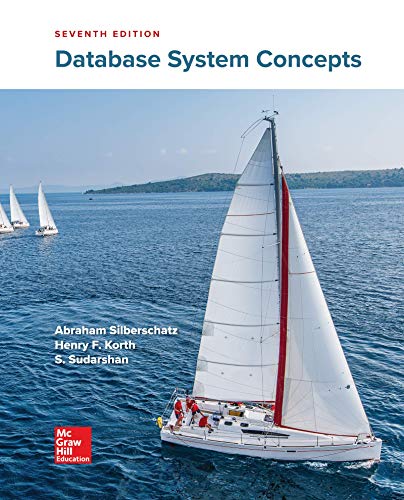
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
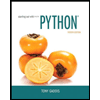
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
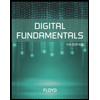
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
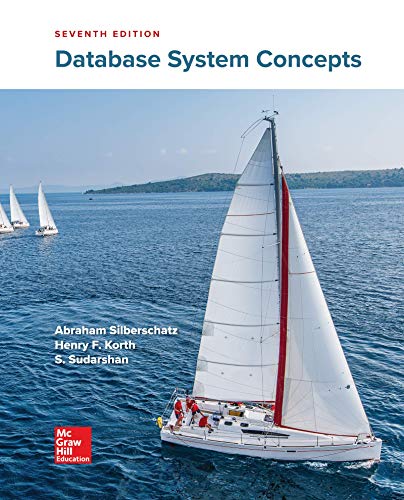
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
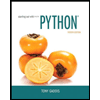
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
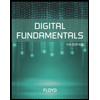
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
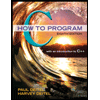
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
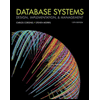
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
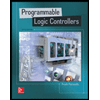
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education