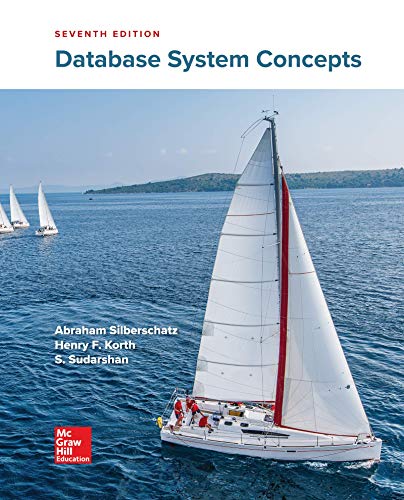
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![1. Consider a 5-stage pipeline (IF, ID, EX, MEM, WB) processor implementation that does NOT use bypassing/forwarding and does NOT use delayed branches. Assume that register reads can occur in the same cycle that the register write-backs are done. For the below code, how many stalls will be observed? Assume that the loop runs a total of 1024 times.
```
Label: ldw r8, 0(r10) ; load into r8 from MEM[0+r10]
add r9, r8, r7 ; r9 = r8 + r7
stw r9, 0(r12) ; store r9 into MEM[0+r12]
addi r10, r10, 4 ; r10 = r10 + 4
addi r12, r12, 4 ; r12 = r12 + 4
bne r10, r11, Label; branch to Label if r10 != r11
```
**Explanation of the Code and Pipeline Considerations:**
- **Pipeline Stages**:
- IF: Instruction Fetch
- ID: Instruction Decode
- EX: Execute
- MEM: Memory Access
- WB: Write Back
- **Operations**:
- `ldw r8, 0(r10)`: Loads value into `r8` from memory address computed as `0 + value in r10`.
- `add r9, r8, r7`: Adds values in `r8` and `r7` and stores the result in `r9`.
- `stw r9, 0(r12)`: Stores the value from `r9` to memory address computed as `0 + value in r12`.
- `addi r10, r10, 4`: Increments the value in `r10` by 4.
- `addi r12, r12, 4`: Increments the value in `r12` by 4.
- `bne r10, r11, Label`: Branches back to `Label` if `r10` does not equal `r11`.
- **Considerations**:
- The pipeline has a potential for stalls due to data hazards, as there is no bypassing/forwarding.
- Data dependencies may occur,](https://content.bartleby.com/qna-images/question/e8fe02c7-ed3b-4cf1-b18f-0db9f6ecbcc2/24373b96-2b18-4279-a3ed-02c25ebc7448/c1unvsr_thumbnail.png)
Transcribed Image Text:1. Consider a 5-stage pipeline (IF, ID, EX, MEM, WB) processor implementation that does NOT use bypassing/forwarding and does NOT use delayed branches. Assume that register reads can occur in the same cycle that the register write-backs are done. For the below code, how many stalls will be observed? Assume that the loop runs a total of 1024 times.
```
Label: ldw r8, 0(r10) ; load into r8 from MEM[0+r10]
add r9, r8, r7 ; r9 = r8 + r7
stw r9, 0(r12) ; store r9 into MEM[0+r12]
addi r10, r10, 4 ; r10 = r10 + 4
addi r12, r12, 4 ; r12 = r12 + 4
bne r10, r11, Label; branch to Label if r10 != r11
```
**Explanation of the Code and Pipeline Considerations:**
- **Pipeline Stages**:
- IF: Instruction Fetch
- ID: Instruction Decode
- EX: Execute
- MEM: Memory Access
- WB: Write Back
- **Operations**:
- `ldw r8, 0(r10)`: Loads value into `r8` from memory address computed as `0 + value in r10`.
- `add r9, r8, r7`: Adds values in `r8` and `r7` and stores the result in `r9`.
- `stw r9, 0(r12)`: Stores the value from `r9` to memory address computed as `0 + value in r12`.
- `addi r10, r10, 4`: Increments the value in `r10` by 4.
- `addi r12, r12, 4`: Increments the value in `r12` by 4.
- `bne r10, r11, Label`: Branches back to `Label` if `r10` does not equal `r11`.
- **Considerations**:
- The pipeline has a potential for stalls due to data hazards, as there is no bypassing/forwarding.
- Data dependencies may occur,
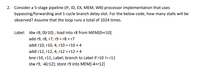
Transcribed Image Text:The given assembly code is to be analyzed for stalls in a 5-stage pipeline processor that includes the stages: Instruction Fetch (IF), Instruction Decode (ID), Execute (EX), Memory Access (MEM), and Write Back (WB). The processor utilizes bypassing/forwarding and includes a 1-cycle branch delay slot.
### Pipeline Stages and Code Analysis:
**Code:**
1. `ldw r8, 0(r10)`
- Load word into `r8` from memory address `0 + r10`.
2. `add r9, r8, r7`
- Add `r8` and `r7`, store the result in `r9`.
3. `addi r10, r10, 4`
- Add immediate value `4` to `r10`, store result in `r10`.
4. `addi r12, r12, 4`
- Add immediate value `4` to `r12`, store result in `r12`.
5. `bne r10, r11, Label`
- Branch to `Label` if `r10` is not equal to `r11`.
6. `stw r9, -4(r12)`
- Store word from `r9` into memory at address `-4 + r12`.
### Key Considerations:
- **Bypassing/Forwarding:** This is used to minimize stalls by directly forwarding data to dependent instructions without waiting for them to be written back to the register file.
- **1-Cycle Branch Delay Slot:** The instruction immediately following a branch will execute regardless of the branch outcome.
### Stalls:
1. **Data Hazard between instructions 1 (`ldw`) and 2 (`add`):**
- The `add` instruction depends on the result of the `ldw`, which might cause a stall if forwarding fails to resolve the dependency.
2. **Branch Hazard at instruction 5 (`bne`):**
- Even with a 1-cycle delay slot, control hazards (branch instructions) can cause stalls due to instruction fetches that might get invalidated if the branch is taken.
### Total Stalls:
Without explicit mention of forwarding mechanisms resolving these dependencies, generally:
- **1 Stall** can be assumed for the data hazard from `ldw` to `add`.
- **1 Stall** for the branch instruction `bne`, given the delay slot utilization.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- On an ARM processor, assuming that [N-bit] = 0, [Z-bit] = 0, [C-bit] = 1, [V-bit] = 1, predict whether each of the following branch instruction is going to make the flow of control branch to the instruction labeled by NEXT (i.e., YES or NO). (These instructions are NOT executed one after the other one; instead, each instruction starts with the initial conditions given in the statement.) (a) BLS NEXT (b) BNE NEXT (c) BLE NEXT (d) BVC NEXTarrow_forward:- We will be implementing a new instruction within the MIPS architecture. New instruction will decrement a Variable stored in a memory location and store the decremented value in a register (Rt). This new instruction will be called DECR. Its usage and interpretation is Usage: DECR Offset(4*Rs),Rt Interpretation: Reg[Rt]= Mem[4*Rs+Offset] - 1 Which blocks are used and which control signals are generated for this instruction. How would the Instruction code fields look like ? Do we need to add an extra hardware logic, explain ? (You may draw a simplified datapath flow)arrow_forwardCWDE converts word to double-word extending using EAX: AX . in which the sign of the 16-bit register, AX, is extended into the 32-bit register, EAX. What is the value for registers AX and EAX after executing the mnemonic opcode/operand instruction below? MOV EAX, 0 MOV AX, 0x429FCDA5 CWDE a. AX= CDA5 EAX=0000CDA5 O b. AX=CDA5 EAX=EFFFCDA5 O C. AX=CDA5 EAX=FFFE000 O d. AX= CDA5 EAX=FFFFCDA5arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
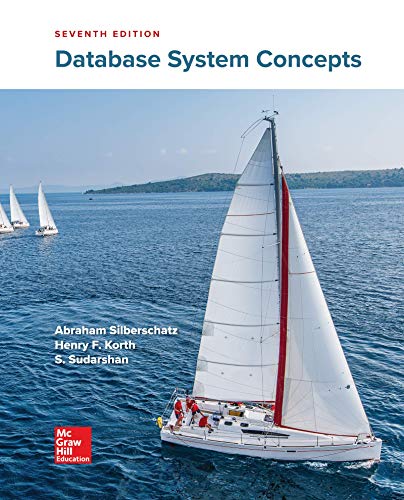
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
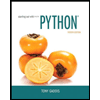
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
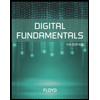
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
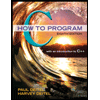
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
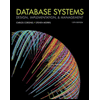
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
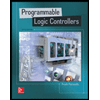
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education