8. Write a function biggestBuried(s), which the parameter s is a string, and the function returns the largest integer buried in the string. If there is no integer inside the string, then return 0. For example, biggestBuried('abcd51kkk3kk19ghi') would return 51, and biggestBuried('kkk32abce@@-33bb14zzz') would return 33, since the " character is treated like any other non digit. Note that a character is a digit if it is greater than or equal to '0' and less than or equal to '9'. Alternatively, you can use string.isdigit() to check if the string is a digit. Use the function in a program to test that it works properly by saying print(biggestBuried('abcd51kkk3kk19ghi')) print(biggestBuried(kkk32abce@@-33bb14zzz')) print(biggestBuried('this15isast22ring-55')) answer should be 51 answer should be 33 answer should be 55
8. Write a function biggestBuried(s), which the parameter s is a string, and the function returns the largest integer buried in the string. If there is no integer inside the string, then return 0. For example, biggestBuried('abcd51kkk3kk19ghi') would return 51, and biggestBuried('kkk32abce@@-33bb14zzz') would return 33, since the " character is treated like any other non digit. Note that a character is a digit if it is greater than or equal to '0' and less than or equal to '9'. Alternatively, you can use string.isdigit() to check if the string is a digit. Use the function in a program to test that it works properly by saying print(biggestBuried('abcd51kkk3kk19ghi')) print(biggestBuried(kkk32abce@@-33bb14zzz')) print(biggestBuried('this15isast22ring-55')) answer should be 51 answer should be 33 answer should be 55
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can u solve 8 and 9 in Python. I will rate you

Transcribed Image Text:Certainly! Here's the transcription of the text for an educational website:
---
### 8. Find the Largest Integer in a String
**Objective:** Write a function `biggestBuried(s)`, where the parameter `s` is a string. The function should return the largest integer found within the string. If there is no integer in the string, the function should return `0`.
**Example Usage:**
- `biggestBuried('abcd51kkk3kk19ghi')` would return `51`.
- `biggestBuried('kkk32abce@@-33bb14zzz')` would return `33`, as the '-' character is treated like any non-digit.
**Important Notes:**
- A character is considered a digit if it falls between '0' and '9'.
- Alternatively, use `string.isdigit()` to check if a character is a digit.
**Verification:**
Use the following to test the function:
```python
print(biggestBuried('abcd51kkk3kk19ghi')) # Expected output: 51
print(biggestBuried('kkk32abce@@-33bb14zzz')) # Expected output: 33
print(biggestBuried('this15isat22ring-55')) # Expected output: 55
```
### 9. Square Root Calculation
**Objective:** Write a function `squareRoot(x, epsilon)` that uses bisection search. The function should return a number `y` that approximates the square root of `x`, such that `abs(y**2 - x) < epsilon`.
**Task:** Test this function in a separate program to ensure accuracy.
---
This content serves as a guide for programming students to understand how to manipulate strings and use numerical methods.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
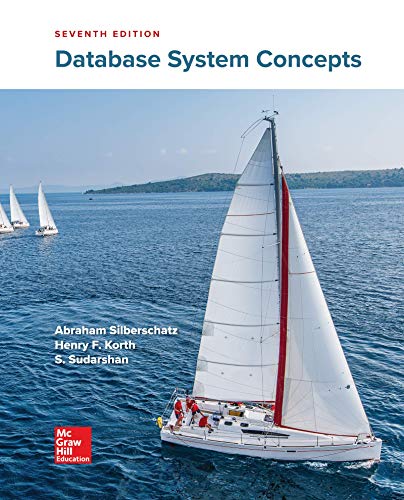
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
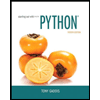
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
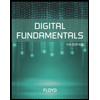
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
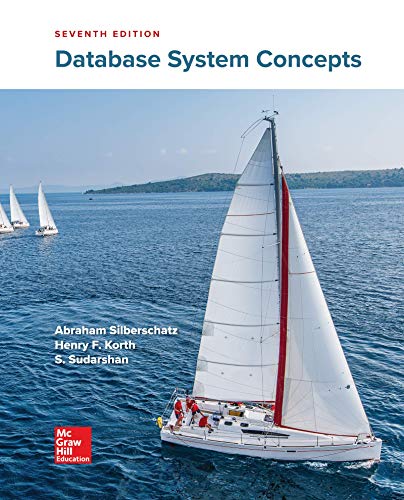
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
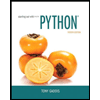
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
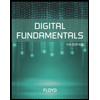
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
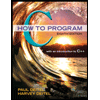
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
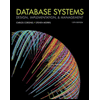
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
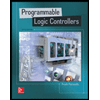
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education