7.4 LAB: Soccer team roster (Arrays) This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. Use a struct named Player to store the name, jersey number and rating for a single player. Use an array of struct to store the data for the entire team. Use a global named constant to store the size of the array (and use it in your program). Jersey numbers are between 0 and 99 and ratings are between 1 and 9. You must use the bolded identifiers above when naming your struct and your last struct member, in order for the test cases to work. The program should keep an array of 5 Player structures. When the program runs, it should initialize the array using the following data: {"Lloyd", 10, 8), {"Pugh", 11, 5), ("Morgan", 13, 7), {"Rapinoe", 15, 5), ("Dunn", 19, 6) Next, it should prompt the user to update player ratings. The user will enter a jersey number and a new rating for each desired player. The user will enter-1 for the jersey (and no new rating) to quit updating player ratings. Your program must define and use the following function: void updateRating (Player t[], int player Jersey, int playerRating) to change a single player's rating. It should not do any input or output. Finally the program should prompt the user for a rating. Then it should print the name, jersey number and rating for all players with ratings above the entered value. Your program must define and use the following function: void aboveRating (Player t[], int playerRating) to output the players above the given rating. Sample output: Update player rating, enter -1 to quit: Enter a jersey number: 13 Enter a new rating for player: 9 Enter a jersey number: 15 Enter a new rating for player: 4 Enter a jersey number: -1
7.4 LAB: Soccer team roster (Arrays) This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. Use a struct named Player to store the name, jersey number and rating for a single player. Use an array of struct to store the data for the entire team. Use a global named constant to store the size of the array (and use it in your program). Jersey numbers are between 0 and 99 and ratings are between 1 and 9. You must use the bolded identifiers above when naming your struct and your last struct member, in order for the test cases to work. The program should keep an array of 5 Player structures. When the program runs, it should initialize the array using the following data: {"Lloyd", 10, 8), {"Pugh", 11, 5), ("Morgan", 13, 7), {"Rapinoe", 15, 5), ("Dunn", 19, 6) Next, it should prompt the user to update player ratings. The user will enter a jersey number and a new rating for each desired player. The user will enter-1 for the jersey (and no new rating) to quit updating player ratings. Your program must define and use the following function: void updateRating (Player t[], int player Jersey, int playerRating) to change a single player's rating. It should not do any input or output. Finally the program should prompt the user for a rating. Then it should print the name, jersey number and rating for all players with ratings above the entered value. Your program must define and use the following function: void aboveRating (Player t[], int playerRating) to output the players above the given rating. Sample output: Update player rating, enter -1 to quit: Enter a jersey number: 13 Enter a new rating for player: 9 Enter a jersey number: 15 Enter a new rating for player: 4 Enter a jersey number: -1
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C++. Not allowed to use
![7.4 LAB: Soccer team roster (Arrays)
This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team.
Use a struct named Player to store the name, jersey number and rating for a single player. Use an array of struct to store the data for the
entire team. Use a global named constant to store the size of the array (and use it in your program). Jersey numbers are between 0 and 99
and ratings are between 1 and 9. You must use the bolded identifiers above when naming your struct and your last struct member, in order
for the test cases to work.
The program should keep an array of 5 Player structures. When the program runs, it should initialize the array using the following data:
{"Lloyd", 10, 8},
{"Pugh", 11, 5},
{"Morgan", 13, 7},
{"Rapinoe",15, 5},
{"Dunn", 19, 6}
Next, it should prompt the user to update player ratings. The user will enter a jersey number and a new rating for each desired player. The
user will enter -1 for the jersey (and no new rating) to quit updating player ratings.
Your program must define and use the following function:
void updateRating (Player t[], int player Jersey, int playerRating) to change a single player's rating. It should not
do any input or output.
Finally the program should prompt the user for a rating. Then it should print the name, jersey number and rating for all players with ratings
above the entered value.
Your program must define and use the following function:
void aboveRating (Player t[], int playerRating) to output the players above the given rating.
Sample output:
Update player rating, enter -1 to quit:
Enter a jersey number:
13
Enter a new rating for player:
9
Enter a jersey number:
15
Enter a new rating for player:
4
Enter a jersey number:
-1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda17b6d9-f7ff-4a56-9763-458bee0c35b1%2F52354105-d74c-4c1a-abf7-2072395512f0%2Fgqqs0pa_processed.png&w=3840&q=75)
Transcribed Image Text:7.4 LAB: Soccer team roster (Arrays)
This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team.
Use a struct named Player to store the name, jersey number and rating for a single player. Use an array of struct to store the data for the
entire team. Use a global named constant to store the size of the array (and use it in your program). Jersey numbers are between 0 and 99
and ratings are between 1 and 9. You must use the bolded identifiers above when naming your struct and your last struct member, in order
for the test cases to work.
The program should keep an array of 5 Player structures. When the program runs, it should initialize the array using the following data:
{"Lloyd", 10, 8},
{"Pugh", 11, 5},
{"Morgan", 13, 7},
{"Rapinoe",15, 5},
{"Dunn", 19, 6}
Next, it should prompt the user to update player ratings. The user will enter a jersey number and a new rating for each desired player. The
user will enter -1 for the jersey (and no new rating) to quit updating player ratings.
Your program must define and use the following function:
void updateRating (Player t[], int player Jersey, int playerRating) to change a single player's rating. It should not
do any input or output.
Finally the program should prompt the user for a rating. Then it should print the name, jersey number and rating for all players with ratings
above the entered value.
Your program must define and use the following function:
void aboveRating (Player t[], int playerRating) to output the players above the given rating.
Sample output:
Update player rating, enter -1 to quit:
Enter a jersey number:
13
Enter a new rating for player:
9
Enter a jersey number:
15
Enter a new rating for player:
4
Enter a jersey number:
-1

Transcribed Image Text:Sample output:
Update player rating, enter -1 to quit:
Enter a jersey number:
13
Enter a new rating for player:
9
Enter a jersey number:
15
Enter a new rating for player:
4
Enter a jersey number:
-1
Enter a rating:
7
ABOVE 7
Lloyd Jersey number: 10, Rating: 8
Morgan
Jersey number: 13, Rating: 9
Do not use vectors.
417118.2791720.qx3zqy7
LAB
ACTIVITY
1 #include <iostream>
2 using namespace std;
3
4 int main() {
6% VSST
5
7.4.1: LAB: Soccer team roster (Arrays)
6 /* Type your code here. */
7
8 return 0;
9}
main.cpp
20 / 62
Load default template...
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
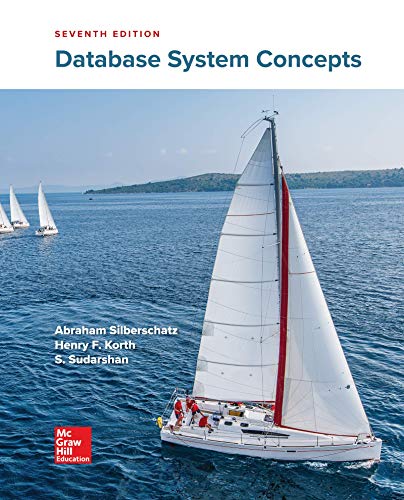
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
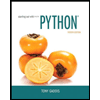
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
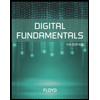
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
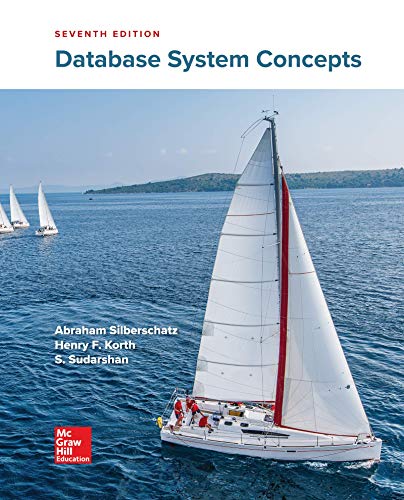
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
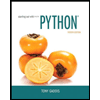
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
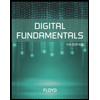
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
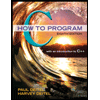
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
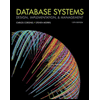
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
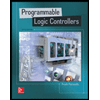
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education