5 ;; N=5 manatees 1 2 3 4 5 1 2 3 4 5 5 4 1 2 3 0 ;; End of first test case 6 ;; N=6 manatees 1 2 3 4 5 6 6 5 4 3 2 1 0 ;; End of second test case 0 ;; No more test cases (The text in blue is commentary and will not be present in the inp
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Given the desired order in which the manatees are to arrive down south, how do I dermine if there is any way Hugh can use the drainpipe to achive the desired order?
This problem can be solved by using a stack. A stack is an ordered collected of elements that operations to push(), peek(), pop(). In Java the interface Deque has those operations. The ArrayDeque class implements the Deque Interface.
Input
All input is to be read from the standard input stream.
The input consists of several blocks of lines and is terminated by a line with just 0.
The first line of each block indicates the number of manatees 0 < N < 100,000. In each of the next lines of the block there is a permutation of the numbers 1, 2, ..., N. For N=3, the permutation 2 3 1 means that the first manatee needs to be placed at the end of line and that the second manatee needs to be placed at the front of the line. The last line of each block contains just 0 signaling the end of the block.
Output
For each permutation in the input, the output is either the word "Yes" or "No" on a line by itself. See the sample below.
Sample Input
5 ;; N=5 manatees 1 2 3 4 5
1 2 3 4 5
5 4 1 2 3
0 ;; End of first test case
6 ;; N=6 manatees 1 2 3 4 5 6
6 5 4 3 2 1
0 ;; End of second test case
0 ;; No more test cases
(The text in blue is commentary and will not be present in the input.)
Sample Output
Yes ;; 1 2 3 4 5 <-- 1 2 3 4 5 is possible
No ;; 5 4 1 2 3 <-- 1 2 3 4 5 is NOT possible
Yes ;; 6 5 4 3 2 1 <-- 1 2 3 4 5 6 is possible
(The text in blue is commentary and must not appear in the output.)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

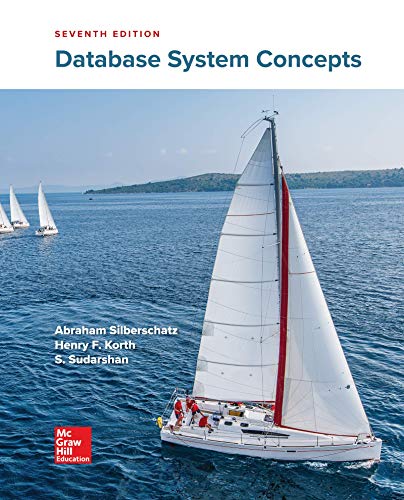
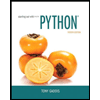
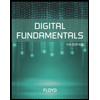
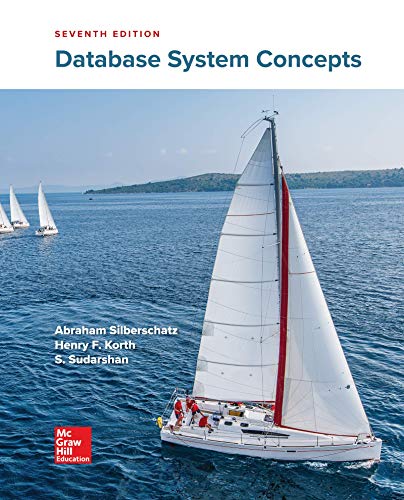
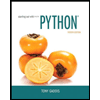
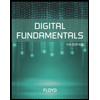
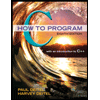
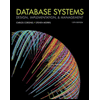
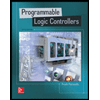