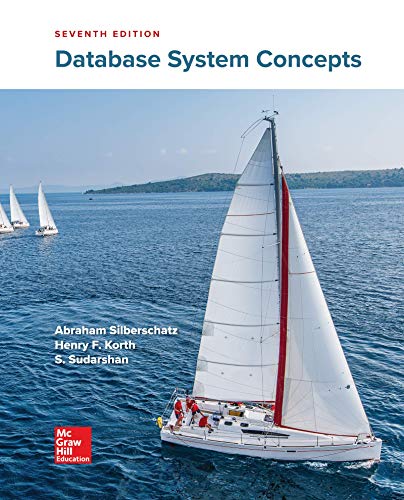
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
eqweqweq
python please.
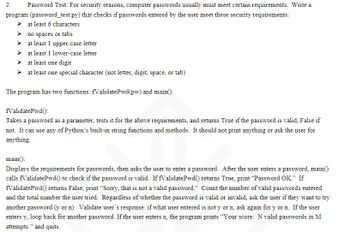
Transcribed Image Text:### Password Test Program Overview
This guide describes how to create a program (`password_test.py`) ensuring that user-entered passwords meet specific security requirements. The program will check if the passwords fulfill the following criteria:
- Minimum of 6 characters
- No spaces or tabs
- At least one uppercase letter
- At least one lowercase letter
- At least one digit
- At least one special character (not a letter, digit, space, or tab)
### Program Components
The program is composed of two functions: `fValidatePwd(pw)` and `main()`.
#### 1. `fValidatePwd(pw)`
- **Purpose**: To validate if a password meets the specified security requirements.
- **Functionality**:
- Takes a password as an input parameter.
- Checks against the stated requirements.
- Returns `True` if valid, `False` otherwise.
- Utilizes Python's built-in string functions and methods without printing or asking for user inputs.
#### 2. `main()`
- **Purpose**: To interact with the user, obtain password input, and validate it.
- **Functionality**:
- Displays password requirements to the user.
- Prompts the user to enter a password.
- Calls `fValidatePwd()` to validate the entered password.
- Prints "Password OK." if the password is valid.
- Prints "Sorry, that is not a valid password." if invalid.
- Keeps a count of the total and the number of valid passwords attempted.
- Regardless of validity, asks the user if they want to try another password:
- If yes (`y`), the loop continues for another attempt.
- If no (`n`), the program prints "Your score: N valid passwords in M attempts." and exits.
This program ensures that passwords are strong and comply with security standards by systematically checking through a series of criteria and providing user feedback.
Expert Solution

arrow_forward
Step 1
Please find the answer below :
Step by stepSolved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Attach File Browse My Computer QUESTION 6 A. Simplify the following expression by using the laws of logic. Suppose the bit strings of A & B are A: 0100001010, B: 0100111011. Find the bit strings of ANB AUB Attach File Browse My Computer BAAAarrow_forwardPlease give me the html code of this programarrow_forwardplease helparrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
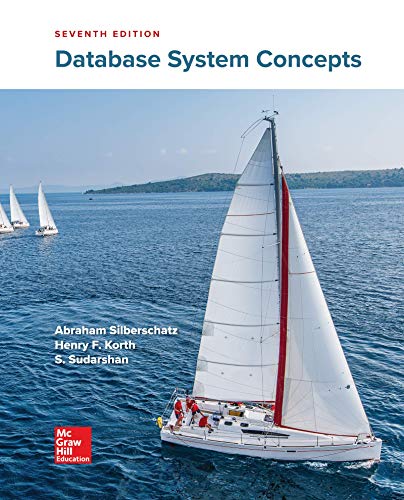
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
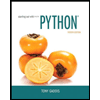
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
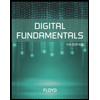
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
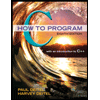
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
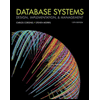
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
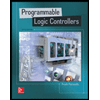
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education