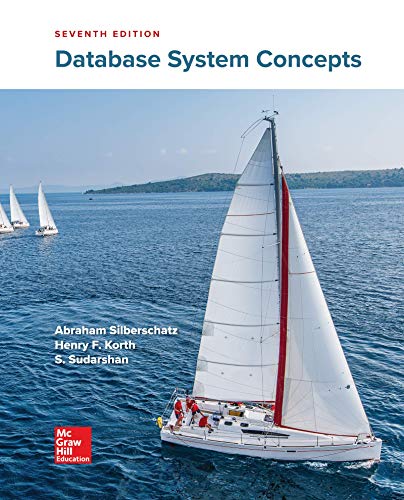
17.37 PRACTICE: Vectors **: Longest sequence C++
A common statistic of interest is the longest sequence of some pattern in a list of items. For example, in football, one may be interested in the longest sequence of consecutive complete passes. Given a list in which each item is either the letter "I" (for an incomplete pass) or a number (for a completed pass), output the length of the longest sequence of complete passes. The list is preceded by the number of items. Given 8 4 15 9 I 30 2 I 20, the output is 3 (because the longest sequence of complete passes is 4 15 9).
Hints:
-
Use two variables, one that keeps track of the current sequence length, and another for the longest sequence length.
-
In a for loop that iterates through the vector, if you see an "I", set the current sequence length to 0.
-
Otherwise, you are in sequence (either at the beginning or later in the sequence), so increment the current sequence length.
-
A simple way to compute the longest sequence is to potentially update that variable every time you update the current sequence length. Check if the current is greater than the longest -- if so, update the longest.
-
When done iterating, just output the value of the longest sequence length variable.
#include <iostream>
#include <vector>
#include <string>
using namespace std;
int main() {
int numItems;
vector<string> listItems;
string currItem;
int i;
// Get items
cin >> numItems;
for (i = 0; i < numItems; ++i) {
cin >> currItem;
listItems.push_back(currItem);
}
/* Type your code here */
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- 2.15 LAB: Descending selection sort with output during execution Write a program that takes an integer list as input and sorts the list into descending order using selection sort. The program should use nested loops and output the list after each iteration of the outer loop, thus outputting the list N-1 times (where N is the size of the list). Important Coding Guidelines: Use comments, and whitespaces around operators and assignments. Use line breaks and indent your code. Use naming conventions for variables, functions, methods, and more. This makes it easier to understand the code. Write simple code and do not over complicate the logic. Code exhibits simplicity when it’s well organized, logically minimal, and easily readable. Ex: If the input is: 20 10 30 40 the output is: [40, 10, 30, 20] [40, 30, 10, 20] [40, 30, 20, 10] Ex: If the input is: 7 8 3 the output is: [8, 7, 3] [8, 7, 3] Note: Use print(numbers) to output the list numbers and achieve the format shown in the…arrow_forwardExams.cpp) Suppose a teacher weights the fourexams he gives 10%, 25%, 30%, and 35%. Write a programthat reads ten sets of four grades, prints the weightedaverage of each set, and prints the unweighted average ofeach test. The number of students should be in a globalconstant.arrow_forward11.10 LAB: All permutations of names C++ Write a program that lists all ways people can line up for a photo (all permutations of a list of strings). The program will read a list of one word names (until -1), and use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia -1 then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Julia Please help with //TODO #include <vector>#include <string>#include <iostream> using namespace std; // TODO: Write method to create and output all permutations of the list of names.void AllPermutations(const vector<string> &permList, const vector<string> &nameList) { } int main(int argc, char* argv[]) {vector<string> nameList;vector<string> permList;string name; // TODO: Read in a list of names; stop when -1 is read. Then call…arrow_forward
- Python: 6.28 LAB: Replacement words Write a program that replaces words in a sentence. The input begins with word replacement pairs (original and replacement). The next line of input is the sentence where any word on the original list is replaced. Ex: If the input is: automobile car manufacturer maker children kids The automobile manufacturer recommends car seats for children if the automobile doesn't already have one. the output is: The car maker recommends car seats for kids if the car doesn't already have one.arrow_forward13) What's a Map? Homework • Unanswered Select all true statements from the below. Multiple answers: Multiple answers are accepted for this question Select one or more answers and submit. For keyboard navigation. SHOW MORE v a The idea of a map is to be able to look up one value with another. Often the lookup value is called the key and the looked-up value is called simply the value. As such, we see that any data structure essentially performs the actions required of a map.arrow_forwardET-574 - Lists II - Week 4 LAB 1. Lists and loops. a) Implement a list of the numbers 1 2 3 4 5. b) Use a loop upon this list to compute and print the multiplication table of the number 3. Output Example 3 3 3 = 6 3* 3 = 9 3 12 3 15 1 2 4 5 1 2. List and range. a) Implement an empty list. b) Use a loop and range to store the values 1 2 3 4 5 6 7 8 9 10 in the list. c) Use a loop to compute and print the square of each value in the list on a single line separated by spaces. Output Example 1 4 9 16 25 36 49 64 81 100 3. List and range with step. a) Implement an empty list. b) Use a loop and range with the step parameter to store the multiplication table of 5 in the list from 0 to 50. c) Print the list on a comma separated line. Output Example 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50,arrow_forward
- 3.6 LAB: Number pattern Write a recursive function called print_num_pattern() to output the following number pattern. Given a positive integer as input (Ex: 12), subtract another positive integer (Ex: 3) continually until 0 or a negative value is reached, and then continually add the second integer until the first integer is again reached. For coding simplicity, output a space after every integer, including the last. Do not end output with a newline. Ex. If the input is: 12 3 the output is: 12 9 6 3 0 3 6 9 12arrow_forwardCoin flips and events. 1)A coin is flipped four times. For each of the events described below, express the event as a set in roster notation. Each outcome is written as a string of length 4 from {H, T}, such as HHTH. Assuming the coin is a fair coin, give the probability of each event. (b) There are at least two consecutive flips that come up heads. (c) The first flip comes up tails and there are at least two consecutive flips that come up heads.arrow_forward4. List comprehension. a) Implement a list comprehension that creates a list of all odd numbers between 1 and 20 inclusive. b) Print the list with the elements separated by a space. Output Example 1 3 5 7 9 11 13 15 17 19 5. List slicing. a) Use a list comprehension to generate a list of all even numbers from 0 to 100 inclusive. Print the list. b) Use slicing to print the first five numbers in the list. c) Use slicing to print the last five numbers in the list. Hint: use len () d) Use slicing to print all list numbers between 25 and 30 inclusive. 2 Output Example [0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24, 26, 28, 30, 32, 34, 36, 38, 40, 42, 44, 46, 48, 50] [0, 2, 4, 6, 8] [32, 34, 36, 38, 40, 42, 44, 46, 48, 50] [20, 22, 24, 26, 28, 30]arrow_forward
- C Programming plz 5.17 LAB: Middle item Given a sorted list of integers, output the middle integer. A negative number indicates the end of the input (the negative number is not a part of the sorted list). Assume the number of integers is always odd. Ex: If the input is: 2 3 4 8 11 -1 the output is: Middle item: 4 The maximum number of list values for any test case should not exceed 9. If exceeded, output "Too many numbers". Hint: First read the data into an array. Then, based on the array's size, find the middle item. #include <stdio.h> int main(void) { const int NUM_ELEMENTS = 9; int userValues[NUM_ELEMENTS]; // Set of data specified by the user /* Type your code here. */ return 0;}arrow_forward6.9 LAB: All permutations of names Write a program that lists all ways people can line up for a photo (all permutations of a list of Strings). The program will read a list of one word names (until -1), and use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia -1 then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Julia import java.util.Scanner;import java.util.ArrayList; public class PhotoLineups { // TODO: Write method to create and output all permutations of the list of names.public static void allPermutations(ArrayList<String> permList, ArrayList<String> nameList) {} public static void main(String[] args) {Scanner scnr = new Scanner(System.in);ArrayList<String> nameList = new ArrayList<String>();ArrayList<String> permList = new ArrayList<String>();String name;//…arrow_forward6.34 LAB: Contact list C++ A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Ex: If the input is: 3 Joe 123-5432 Linda 983-4123 Frank 867-5309 Frank the output is: 867-5309 Your program must define and call the following function. The return value of GetPhoneNumber is the phone number associated with the specific contact name.string GetPhoneNumber(vector<string> nameVec, vector<string> phoneNumberVec, string contactName) Hint: Use two vectors: One for the string names, and the other for the string phone numbers.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
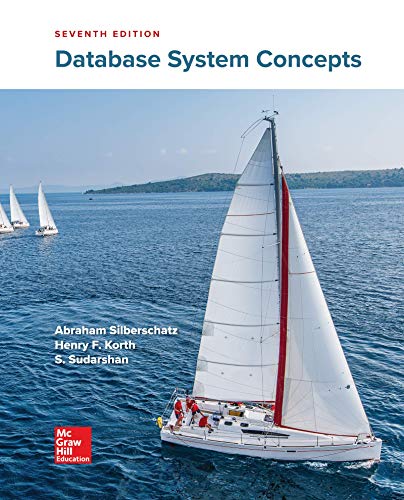
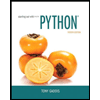
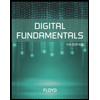
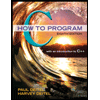
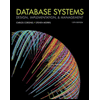
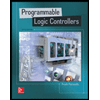