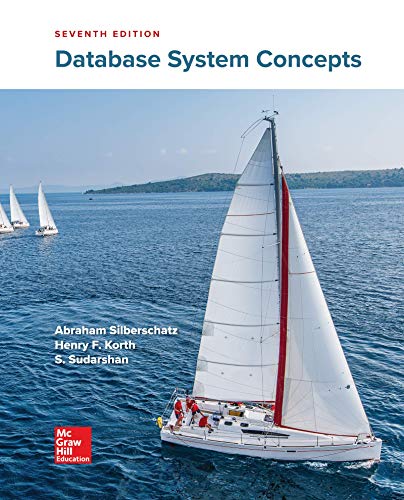
17.37 PRACTICE: Vectors **: Longest sequence C++
A common statistic of interest is the longest sequence of some pattern in a list of items. For example, in football, one may be interested in the longest sequence of consecutive complete passes. Given a list in which each item is either the letter "I" (for an incomplete pass) or a number (for a completed pass), output the length of the longest sequence of complete passes. The list is preceded by the number of items. Given 8 4 15 9 I 30 2 I 20, the output is 3 (because the longest sequence of complete passes is 4 15 9).
Hints:
-
Use two variables, one that keeps track of the current sequence length, and another for the longest sequence length.
-
In a for loop that iterates through the vector, if you see an "I", set the current sequence length to 0.
-
Otherwise, you are in sequence (either at the beginning or later in the sequence), so increment the current sequence length.
-
A simple way to compute the longest sequence is to potentially update that variable every time you update the current sequence length. Check if the current is greater than the longest -- if so, update the longest.
-
When done iterating, just output the value of the longest sequence length variable.
Please answer in c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
int main() {
int numItems;
vector<string> listItems;
string currItem;
int i;
// Get items
cin >> numItems;
for (i = 0; i < numItems; ++i) {
cin >> currItem;
listItems.push_back(currItem);
}
/* Type your code here */
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Q5/ write a program to compute the value of R from X,Y and Z values which are X=[0,1,2......9], Y= [2,4,6,.,20], and Z=(1,3,5,...,19]. Print the values of X,Y,Z, and R as adjacent columns. R = √A²+B²+C² X √x² + y² +2² A = . B=√36X* +9Y²+252² T C = √9+sin³Y+Z MATLABarrow_forward9. Write function getMoveRow to do the following a. Return type integer b. Parameter list i. 1-d character array (i.e. move), size 3 c. Convert the row portion of the player’s move to the associated integer index for the board array d. Example: i. move = ‘b2’ ii. 2 is the row iii. 2 is index 1 in the board array e. Return the row array index that corresponds to the player’s move f. Return a -1 if the row is not valid (i.e. INVALID) 10. Write function getMoveCol to do the following a. Return type integer b. Parameter list i. 1-d character array (i.e. move), size 3 c. Convert the column portion of the player’s move to the associated integer index for the board array d. Example: i. move = ‘b2’ ii. b is the column iii. b is index 1 in the board array e. Return the column array index that corresponds to the player’s move f. Return a -1 if the column is not valid (i.e. INVALID) I am getting an error when entering the code at the very end. please fix it. This is the code in C int…arrow_forwardFASTarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
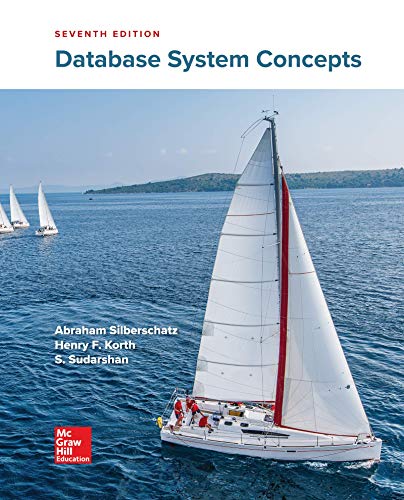
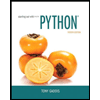
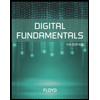
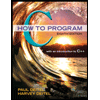
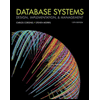
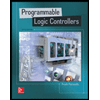