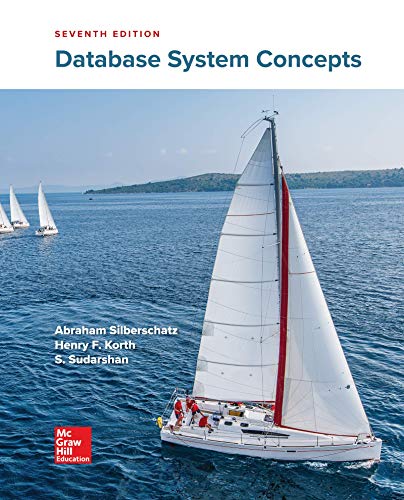
13.12 LAB: Merge sort
The class is the same as shown at the end of the Merge sort section, with the following changes:
- Numbers are entered by a user in a separate helper method, readNums(), instead of defining a specific array in main(). The first number is how many integers to be sorted, and the rest are the integers.
- Output of the array has been moved to the method printNums().
- An output has been added to mergeSort(), showing the indices that will be passed to the recursive method calls.
Add code to the merge sort
Add code at the end of main() that outputs "comparisons: " followed by the number of comparisons performed (Ex: "comparisons: 12")
Hint: Use a static variable to count the comparisons.
Note: Take special care to look at the output of each test to better understand the merge sort algorithm.
Ex: When the input is:
6 3 2 1 5 9 8
the output is:
unsorted: 3 2 1 5 9 8 0 2 | 3 5 0 1 | 2 2 0 0 | 1 1 3 4 | 5 5 3 3 | 4 4 sorted: 1 2 3 5 8 9 comparisons: 8
import java.util.Scanner;
public class LabProgram {
// Read size and create an array of size ints.
// Read size ints, storing them in the array.
// Return the array.
static int compare = 0;
public static int[] readNums() {
Scanner scnr = new Scanner(System.in);
int size = scnr.nextInt(); // Read array size
int[] nums = new int[size]; // Create array
for (int j = 0; j < size; ++j) { // Read the numsbers
nums[j] = scnr.nextInt();
}
return nums; // Return the array
}
// Output the numbers in nums, separated by spaces.
// No space or newline will be output before the first number or after the last.
public static void printNums(int[] nums) {
for (int i = 0; i < nums.length; i++) {
System.out.print(nums[i]);
if (i < nums.length) {
System.out.print(" ");
}
}
}
public static void merge(int[] numbers, int i, int j, int k) {
int mergedSize = k - i + 1;
int mergedNumbers[] = new int[mergedSize];
int mergePos;
int leftPos;
int rightPos;
mergePos = 0;
leftPos = i;
rightPos = j + 1;
while (leftPos <= j && rightPos <= k) {
if (numbers[leftPos] < numbers[rightPos]) {
mergedNumbers[mergePos] = numbers[leftPos];
++leftPos;
}
else {
mergedNumbers[mergePos] = numbers[rightPos];
++rightPos;
}
++mergePos;
}
while (leftPos <= j) {
mergedNumbers[mergePos] = numbers[leftPos];
++leftPos;
++mergePos;
}
while (rightPos <= k) {
mergedNumbers[mergePos] = numbers[rightPos];
++rightPos;
++mergePos;
}
for (mergePos = 0; mergePos < mergedSize; ++mergePos) {
numbers[i + mergePos] = mergedNumbers[mergePos];
}
}
public static void mergeSort(int numbers[], int i, int k) {
int j;
if (i < k) {
j = (i + k) / 2;
/* Trace output added to code in book */
System.out.printf("%d %d | %d %d\n", i, j, j+1, k);
mergeSort(numbers, i, j);
mergeSort(numbers, j + 1, k);
merge(numbers, i, j, k);
}
}
public static void main(String[] args) {
int[] numbers = readNums();
System.out.print("unsorted: ");
printNums(numbers);
System.out.println("\n");
mergeSort(numbers, 0, numbers.length - 1);
System.out.print("\nsorted: ");
printNums(numbers);
System.out.println("\ncomparisons: " + compare);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- Fasssst answer faaaasssst else downvotearrow_forward7.4 LAB: Find student with highest GPA Complete the Course class by implementing the findStudent Highest Gpa() method, which returns the Student object with the highest GPA in the course. Assume that no two students have the same highest GPA. Given classes: • Class LabProgram contains the main method for testing the program. • Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) • Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: getGPA) returns a student's GPA.) Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9- the output is: Top student: Sonya King (GPA: 3.9)arrow_forwardIn c++. Please helparrow_forward
- 1) Write a method in java that performs the union operation on two integer arrays, i.e. it will createa new array that has the values from the two input arrays but with no duplicates.• The method must have the following signature:public static int[] union(int[] a1, int[] a2)• You can assume that no input array element will have a value less than 1.• Your method can return an array that is the same size as the two input arrays puttogether. This will mean, in general, the returned array will have too many elements.This is fine. Any elements you don’t need, you simply set to zero.• Implement this method using Java primitives only. You may use the array lengthproperty.• If you want to implement other methods to help you, you may do so. You must writethe method specified above, but you may write other methods to help you if you wantto. You must show the full implementation of any methods you create, however.arrow_forwardjavaarrow_forward6.19 LAB: Word frequencies - methods Define a method named getWordFrequency that takes an array of strings, the size of the array, and a search word as parameters. Method getWordFrequency() then returns the number of occurrences of the search word in the array parameter (case insensitive). Then, write a main program that reads a list of words into an array, calls method getWordFrequency() repeatedly, and outputs the words in the arrays with their frequencies. The input begins with an integer indicating the number of words that follow. Assume that the list will always contain less than 20 words.arrow_forward
- 11:06 A 57% Cs101: Lab #5 Using Classes and Objects Part II 1. Write a program that computes the area of each color in an archery target. For simplicity, we will consider a simple circular archery target consisting of the colors (in order from inside to outside): yellow, red, blue, black, and white. We also assume that each color in the target is equal 'width'. You must prompt the user for a radius (in cm) of the entire target and report the following information: a. The area of each colored section. Output only four decimal places. b. The percentage (using DecimalFormat) of each colored section (compared to the area of the entire target). You must output the information, in color order, according to the following example. The color yellow has area 3.1416 cm^2 with 48 of the total area. 2. Write a program that takes an input integer from the keyboard by autoboxing to an Integer. Then the program outputs the binary, octal, and hexadecimal representation of the input integer. Your output…arrow_forward23.5 LAB: Random values (Use Python) In main.py define the RandomNumbers class, which has three integer attributes: var1, var2, and var3. Write getter method for each attribute: get_var1(), get_var2() and get_var3(). Then write the following 2 instance methods: set_random_values( ) - accepts a low and high integer values as parameters, and sets var1, var2, and var3 to random numbers within the range of the low and high input values (inclusive). get_random_values( ) - prints out the 3 random numbers in the format: "Random values: var1 var2 var3" Ex: If the input is: 15 20 the output may be: Random values: 17 15 19 where 17, 15, 19 can be any random numbers within 15 and 20 (inclusive). Note: When submitted, your program will be tested against different input range to verify if the three randomly generated values are within range.arrow_forward**Java Code** Think Java Exercise 14.2 Write a loop that plays the game 100 times and keeps track of how many times each player wins. If you implemented multiple strategies in the previous exercise, you can play them against each other to evaluate which one works best. Hint: Design a Genius class that extends Player and overrides the play method, and then replace one of the players with a Genius object.arrow_forward
- Java scriptarrow_forwardLAB, 16.17 LAB: Swapping variables Define a method named swapValues that takes an array of four integers as a parameter, swaps array elements at indices 0 and 1, and swaps array elements at indices 2 and 3. Then write a main program that reads four integers from input and stores the integers in an array in positions 0 to 3. The main program should call function swapValues() to swap array's values and print the swapped values on a single line separated with spaces.?.arrow_forwardExercise 6.14 JHTP: (Variable-Length Argument List) Write an application that calculates the product of a series of integers that are passed to method product using a variable-length argument list. Test your method with several calls, each with a different number of arguments. Exercise 6.17 JHTP: (Dice Rolling) Write an application to simulate the rolling of two dice. The application should use an object of class Random once to roll the first die and again to roll the second die. The sum of the two values should then be calculated. Each die can show an integer value from 1 to 6, so the sum of the clause will vary from 2 to 12, with 7 being the most frequent sum, and 2 and 12 the least frequent. Figure 6.21 shows the 36 possible combinations of the two die. Your application should roll the dice 36,000 times. Use a one-dimensional array to tally the number of times each possible sum appears. Display the results in a tabular format. Fig. 6.21 | The possible sums of two dice.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
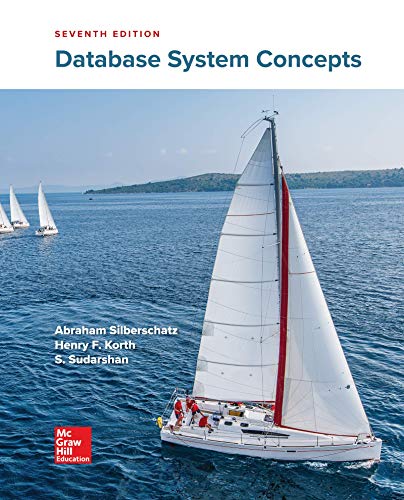
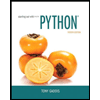
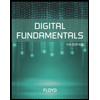
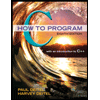
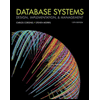
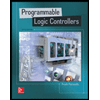