1.A sorted array contains 300,000 elements. The maximum number of operations needed by binary search to check if an element is present in the array would be 2.The correct statement indicating the recurrence relation for mergesort is Blank 1 302: T(n) = T(n/2) + n 303: T(n) = 2T(n/2) + n/2
ANSWER IN ONE NUMBER/WORD/SENTENCE
1.A sorted array contains 300,000 elements. The maximum number of operations needed by binary search to check if an element is present in the array would be
2.The correct statement indicating the recurrence relation for mergesort is Blank 1
302: T(n) = T(n/2) + n
303: T(n) = 2T(n/2) + n/2
304: T(n) = 2T(n/2) + n
306: T(n) = T(n – 1) + 3n
3.Put the most appropriate statement number here
171: Insertion at the end of an array structure takes O(1) time and insertion at the end of a linked list takes O(n) time.
172: Searching the largest element in a random array takes O(log n)
173: Deletion of first element takes O(1) for an array and O(1) for a linked list
174: Searching for mid element takes O(log n) both for a sorted array and a sorted linked list
4.An array contains following elements: 20 42 8 66 12 39 11 26 33
The mergesort method we discussed in the class calls a merge function. The number of times the merge function is going to be called when mergesort is applied on the array is
5.Mergesort is applied on an array containing 50 elements. Once the array gets sorted mergesort is applied a second time on the sorted array. The correct statement number is
6.Find the prefix 7 5 8 * 20 10 – / + 5 –
7.Each node in the list stores an integer and link to next node. Indicate the correct statement number corresponding to following code Blank 1
void fun1(struct Node* head) {
temp = head;
while (temp != NULL)
temp = temp->next;
printf("%d, ", temp->data);
}
200: Fails to print any data of the list 202: Prints the list in first-to-last order.
204: Prints the data of the last node 206: Prints the data in reverse order
8.
struct node {
int data;
struct node *next;
} *AA, *BB;
// create linked list AA with 28 nodes
BB = AA;
Assume that the code shown above creates the linked list AA. Study various choices given below. Out of the following, the most appropriate statement is Blank 1
300: If we use the statement BB= NULL after this,
the complete list is lost and we cannot get access to any of the nodes of the list
302: If we use the statement AA=AA->next after this,
we will not be able to access the first node of AA or BB.
304: If we use the statement AA=AA->next after this,
it will result in AA having 27 nodes while BB will remain unchanged.
306: This will result in creating a copy of the list accessed by BB.
9.M integers have been stored in Stack A, and N integers have been stored in stack B. It is desired to POP all the integers from stack B and PUSH in stack A. The correct statement indicating Big-O for this job is
10. The mergesort method we discussed in the class is applied on the array containing following elements
50 15 39 11 26 33 42 20
After the 3rd merge the correct contents of the array are shown in statement number Blank 1
202: 15 50 11 39 26 33 20 42
204: 11 15 20 26 33 39 42 50
206: 11 15 39 50 26 33 42 20
208: 15 50 11 39 26 33 42 20

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 2 images

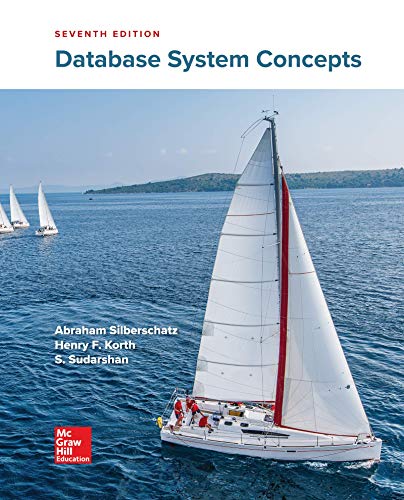
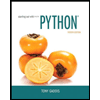
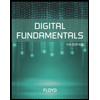
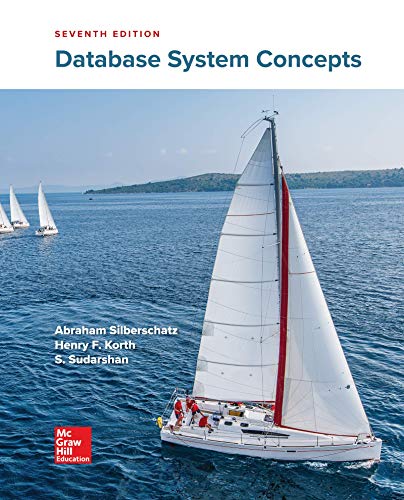
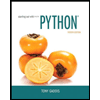
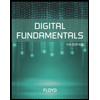
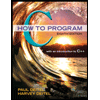
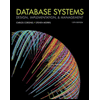
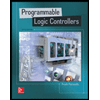