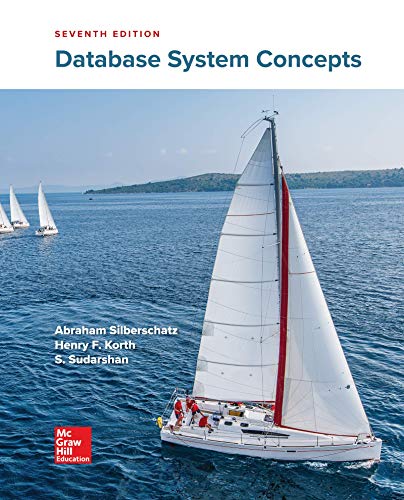
Concept explainers
Write in Python:
(1) Prompt the user to enter four numbers, each corresponding to a person's weight in pounds. Store all weights in a list. Output the list.
Ex:
Enter weight 1: 236.0 Enter weight 2: 89.5 Enter weight 3: 176.0 Enter weight 4: 166.3 Weights: [236.0, 89.5, 176.0, 166.3]
(2) Output the average of the list's elements with two digits after the decimal point. Hint: Use a conversion specifier to output with a certain number of digits after the decimal point.
(3) Output the max list element with two digits after the decimal point.
Ex:
Enter weight 1: 236.0 Enter weight 2: 89.5 Enter weight 3: 176.0 Enter weight 4: 166.3 Weights: [236.0, 89.5, 176.0, 166.3] Average weight: 166.95 Max weight: 236.00
(4) Prompt the user for a number between 1 and 4. Output the weight at the user specified location and the corresponding value in kilograms. 1 kilogram is equal to 2.2 pounds.
Ex:
Enter a list location (1 - 4): 3 Weight in pounds: 176.00 Weight in kilograms: 80.00
(5) Sort the list's elements from least heavy to heaviest weight.
Ex:
Sorted list: [89.5, 166.3, 176.0, 236.0]
Output the average and max weights as floating-point values with two digits after the decimal point, which can be achieved as follows:
print(f'{your_value:.2f}')

Trending nowThis is a popular solution!
Step by stepSolved in 8 steps with 6 images

- Given the following list: numbers = [15, 27, 17, 23, 10, 2, 46] a) Provide a statement that stores the value of the fourth item in the list in variable a: b) Provide a statement that store the value of the last list item in the variable b: c) What is the value of a / b (provide answer in the same format Python would)?arrow_forwardplease code in python Create a program that uses list comprehension to take the following list and create a new list out of elements that have at least one ‘a’ character. Print out the new list.[‘Ford’, ‘Mazda’, ‘BMW’, ‘Nissan’, ‘Hyundai’, ‘Tesla’, ‘Honda’, ‘Subaru’, ‘Kia’, ‘Audi’, ‘Fiat’, ‘Bentley’, ‘Toyota’, ‘Lexus’]arrow_forwardPython programming only NEED HELP PLEASEarrow_forward
- python LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forwardIN PYTHON! finish the incomplete program by writing the code that can be placed below the #write the code. use loops to calculate the sums and avg of each row of scores in the list. the following output should be displayed. dont change any part of the code. OUTPUT: Average scores for students: Student # 1: 12.0 Student # 2: 22.0 CODE: students =[ [11, 12, 13], [21, 22, 23] ] avgs = [] # Write your code here: print('Average scores for students: ") for i, avg in enumerate (avgs): print("Student #', i + 1, ':', avg)arrow_forwardPython code. Please write your own code. Thank you!arrow_forward
- Task 5 Write a Python program that takes numbers as input into a list, removes multiple occurences of any number and then finally prints a list without duplicate values. Hint: You may create a third list to store the results. You can use membership operators (in, not in) to make sure no duplicates are added. Sample Input 1: 0, 0, 1, 2, 3, 4, 4, 5, 6, 6, 6, 7, 8, 9, 4, 4 Sample Output 1: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] Sample Input 2: 7, 7, 7, 1, 0, 3, 3, 55, 9 Sample Output 2: [7, 1, 0, 3, 55, 9]arrow_forwardWrite a program in python that allows the user to enter the total rainfall amounts from your home town for each of 12 months of 2020 into a list. (Use whatever numbers you want) Display the month and the rainfall amounts. The program should calculate and display the total rainfall amount for the year and the average monthly rainfall, Be sure to identify the amounts displayed. Also determine and display the months with the highest and lowest rainfall amounts. When displaying the months with the highest and lowest rainfall, use a string method to display just the first three letters of the months with the highest and lowest rain amounts. REMEMBER to put comments, and write comments through both programs (file creation program, and display program) telling what the program is doing.arrow_forwardAssume, you have been given two lists: List_one and List_two. [Your program should work for any two given lists; change the following lists and check whether your program works correctly for the code you have written] Write a Python program that prints "True", if the given two lists have at least one common member. Otherwise, print "False". Hint: use a boolean variable as a flag to indicate if the two lists have at least one common element. Use break to end the loop when seeing a commom element. =================================================================== Given lists 1:List_one : [1, 4, 3, 2, 6]List_two : [5, 6, 9, 8, 7] Sample Output 1:True =================================================================== Given lists 2:List_one : [1, 4, 3, 2, 5]List_two : [8, 7, 6, 9] Sample Output 2:False #assign the boolean result (True/False) to variable "common_ele" (flag). def task5(list_1, list_2): # YOUR CODE HERE return common_ele Expert Solutionarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
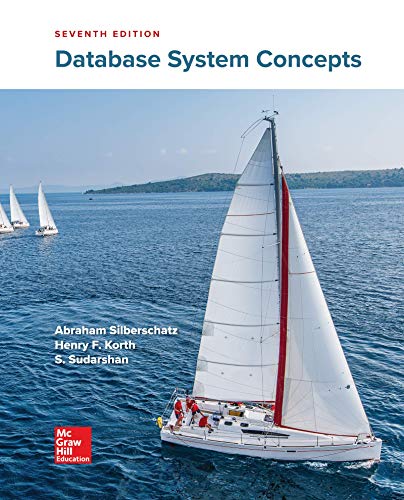
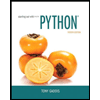
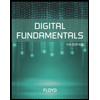
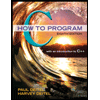
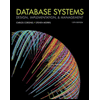
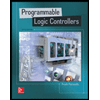