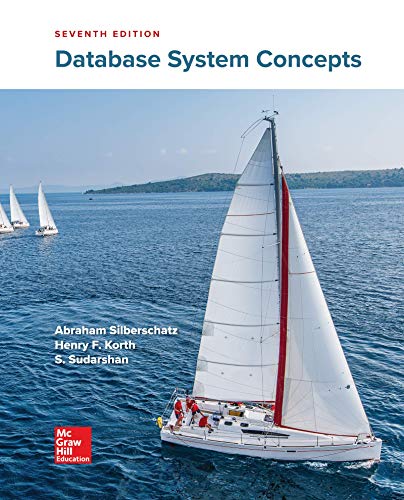
Concept explainers
(1) Prompt the user to enter a string of their choosing. Store the text in a string. Output the string.
Ex:
Enter a sample text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue! You entered: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue!
(2) Implement a PrintMenu() function, which has a string as a parameter, outputs a menu of user options for analyzing/editing the string, and returns the user's entered menu option. Each option is represented by a single character.
If an invalid character is entered, continue to prompt for a valid choice. Hint: Implement Quit before implementing other options. Call PrintMenu() in the main() function. Continue to call PrintMenu() until the user enters q to Quit.
Ex:
MENU c - Number of non-whitespace characters w - Number of words f - Find text r - Replace all !'s s - Shorten spaces q - Quit Choose an option:
(3) Implement the GetNumOfNonWSCharacters() function. GetNumOfNonWSCharacters() has a constant string as a parameter and returns the number of characters in the string, excluding all whitespace. Call GetNumOfNonWSCharacters() in the PrintMenu() function.
Ex:
Number of non-whitespace characters: 181
(4) Implement the GetNumOfWords() function. GetNumOfWords() has a constant string as a parameter and returns the number of words in the string. Hint: Words end when a space is reached except for the last word in a sentence. Call GetNumOfWords() in the PrintMenu() function.
Ex:
Number of words: 35
(5) Implement the FindText() function, which has two strings as parameters. The first parameter is the text to be found in the user provided sample text, and the second parameter is the user provided sample text. The function returns the number of instances a word or phrase is found in the string. In the PrintMenu() function, prompt the user for a word or phrase to be found and then call FindText() in the PrintMenu() function. Before the prompt, call cin.ignore() to allow the user to input a new string.
Ex:
Enter a word or phrase to be found: more "more" instances: 5
(6) Implement the ReplaceExclamation() function. ReplaceExclamation() has a string parameter and updates the string by replacing each '!' character in the string with a '.' character. ReplaceExclamation() DOES NOT output the string. Call ReplaceExclamation() in the PrintMenu() function, and then output the edited string.
Ex.
Edited text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue.
(7) Implement the ShortenSpace() function. ShortenSpace() has a string parameter and updates the string by replacing all sequences of 2 or more spaces with a single space. ShortenSpace() DOES NOT output the string. Call ShortenSpace() in the PrintMenu() function, and then output the edited string.
Ex:
Edited text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue!

Trending nowThis is a popular solution!
Step by stepSolved in 8 steps with 7 images

- Lab Activity for the students: Exercise 5: Write a program that asks the user to input a letter. The program finds and prints if the letter is uppercase or lowercase. Then, the program asks the user to input a string and get a three-character substring from the beginning of the string. (1 Mark ) Example : If the inputs are 'y' and "Community". Then, the program will print: y is lowercase The substring is: Comarrow_forwardAll parts needed 9.arrow_forward(1) Prompt the user to enter a string of their choosing. Store the text in a string. Output the string. Ex: Enter a sample text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue! You entered: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue! (2) Implement the PrintMenu() function to print the following command menu. Ex: MENU c - Number of non-whitespace characters w - Number of words f - Find text r - Replace all !'s s - Shorten spaces q - Quit (3) Implement the ExecuteMenu() function that takes 2 parameters: a character representing the user's choice and the user provided sample text. ExecuteMenu() performs the menu options, according to the…arrow_forward
- Q4: Colouring Book IHaving spent the last couple of hours grading assignments, I decide it's time for a break. I take out my favourite colouring book, turn to a random page I haven't coloured in yet, and lay it on my desk. I then take out all my n crayons and line them up on the desk (it's a very long desk). The colour of the " erayon is a string c11 (eg. "alue"). Many of the crayons have the same colour. In fact, no matter how many crayons I have, there are at most 30 distinct colours amongst them. To start colouring, I always take a sublist (see Qi for a definition) of the crayons laid on the desk and put away the rest (too many options can be overwhelming and can lead to indecision). I take a look at the line art in front of me and wonder, "Ilow many different colours do I need to make this lonk great? One? Two? Mayhe three?". Now you understand my dilemma and are fully aware of my indecision. You kindly decide to help me out by telling me for every number k, if I were to use k…arrow_forwardwhich functions you would use to read the following string from a users input: "This is a fun day" A. cin >> B. cin.get function c. getline function d. all of the abovearrow_forwardcorrect all errors.arrow_forward
- user's input. How could the algorithm be improved so the user does not have to pay End While attention to capitalization when entering a name? 77 This program asks the user to enter a string // and validates the input. Declare String choice // Get the user's response. Display "Cast your vote for Chess Team Captain." Display "Would you like to nominate Lisa or Tim?" Input choice %3D // Validate the input. While choice != "Lisa" AND choice != "Tim" Display "Please enter Lisa or Tim." Display "Cast your vote for Chess Team Captain." Display "Would you like to nominate Lisa or Tim?" Input response End Whilearrow_forward// A standard mortgage is paid monthly over 30 years. // This program is intended to display 360 payment coupons // for a new borrower. Each coupon lists the month number, // year number, and a friendly mailing reminder. start num MONTHS = 12 num YEARS = 30 string MSG = "Remember to allow 5 days for mailing" num monthCounter = 0 num yearCounter = 30 while yearCounter <= YEARS while monthCounter <= MONTHS output monthCounter, yearCounter, MSG endwhile endwhile stop housekeeping() print "Enter account number or ", QUIT, " to quit " return printCoupons() while yearCounter <= YEARS while monthCounter <= MONTHS print acctNum, monthCounter, yearCounter, MSG monthCounter = monthCounter + 1 endwhile yearCounter = yearCounter + 1 endwhile output "Enter account number or ", QUIT, " to quit " input acctNum return finish() output "End of job" returnarrow_forwardcolor: one of the following strings: 'red', 'blue', 'silver', 'white', 'black'year: year of manufacturing. A number between 1990 and 2018company: one of the following strings: 'Honda', 'Toyota', 'Ford', 'Chevrolet'model: one of the following strings: 'sedan', 'hatchback', 'SUV'Give a logical expression that evaluates to a True or False for a set of cars. e.g. Expression for "Red SUV cars" is (color == "red" and model == "SUV") Give an expression for "Ford sedan cars that are red or blue and were manufactured after 2000"arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
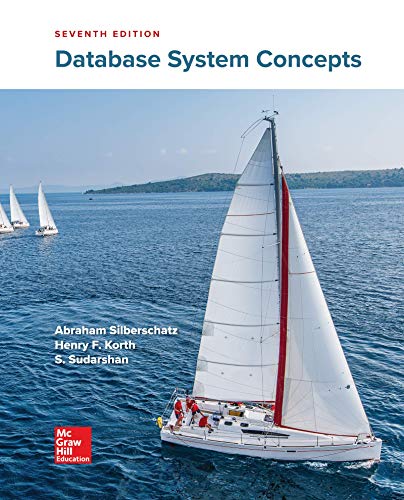
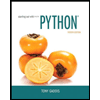
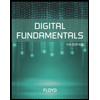
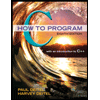
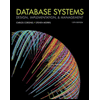
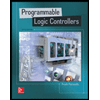