HW5_Fall2023_Soln
pdf
School
University of Illinois, Urbana Champaign *
*We aren’t endorsed by this school
Course
311
Subject
Mechanical Engineering
Date
Dec 6, 2023
Type
Pages
10
Uploaded by MajorRose12050
1. First we need to relate spin rate to lift. We can use the Kutta Jukowski theorem for this.
L
′
=
ρU
inf
Γ
Γ =
v
θ
2
πR
v
θ
=
ωR
ω
=
2
πs
60
Where
s
is the spin rate in rpms.
Plugging in the values given, the lift per unit depth
L
‘ =
mg
2
R
= 19
.
86 N/m, which is generated by a
spin rate of 447.83 rpms.
To plot the streamlines, we will build up the flow field using known velocity fields from a doublet,
vortex and uniform flow.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.colors import BoundaryNorm
from matplotlib.ticker import MaxNLocator
def get_velocity_doublet(k, xd, yd, X, Y):
"""
Returns the velocity field generated by a doublet.
Parameters
----------
k:
float
Strength of the doublet.
xd: float
x-coordinate of the doublet.
yd: float
y-coordinate of the doublet.
X: 2D Numpy array of floats
x-coordinate of the mesh points.
Y: 2D Numpy array of floats
y-coordinate of the mesh points.
Returns
-------
u: 2D Numpy array of floats
x-component of the velocity vector field.
v: 2D Numpy array of floats
y-component of the velocity vector field.
"""
u = (-k / (2 * np.pi) *
((X - xd)**2 - (Y - yd)**2) /
((X - xd)**2 + (Y - yd)**2)**2)
v = (-k / (2 * np.pi) *
2 * (X - xd) * (Y - yd) /
((X - xd)**2 + (Y - yd)**2)**2)
return u, v
1
def get_velocity_vortex(gamma, xv, yv, X, Y):
"""
Returns the velocity field generated by a vortex.
Parameters
----------
gamma: float
Strength of the vortex.
xv: float
x-coordinate of the vortex.
yv: float
y-coordinate of the vortex.
X: 2D Numpy array of floats
x-coordinate of the mesh points.
Y: 2D Numpy array of floats
y-coordinate of the mesh points.
Returns
-------
u: 2D Numpy array of floats
x-component of the velocity vector field.
v: 2D Numpy array of floats
y-component of the velocity vector field.
"""
u = +gamma / (2 * np.pi) * (Y - yv) / ((X - xv)**2 + (Y - yv)**2)
v = -gamma / (2 * np.pi) * (X - xv) / ((X - xv)**2 + (Y - yv)**2)
return u, v
def get_velocity_freestream(U_inf, theta, x, y, dim=2):
"""
Returns the velocity field generated by a vortex.
Parameters
----------
U_inf: float
freestream velocity
theta: float
angle of freestream flow
X: 2D Numpy array of floats
x-coordinate of the mesh points.
Y: 2D Numpy array of floats
y-coordinate of the mesh points.
Returns
-------
u: 2D Numpy array of floats
x-component of the velocity vector field.
v: 2D Numpy array of floats
y-component of the velocity vector field.
"""
if dim == 2:
2
u = U_inf * np.cos(theta) * np.ones((x.shape[0], y.shape[0]))
v = U_inf * np.sin(theta) * np.ones((x.shape[0], y.shape[0]))
elif dim == 1:
u = U_inf * np.cos(theta) * np.ones(x.shape[0])
v = U_inf * np.sin(theta) * np.ones(x.shape[0])
return u, v
We then define all of the necessary parameters.
U_inf = 40.2 # m/s
x_center = 0 # m
y_center = 0 # m
R = 0.037 # m
P = 101325 # Pa
F_g = 9.8 * 0.15 # N
F_g_per_depth = F_g / (2*R) # N/m
rho = 1.225 # kg/m^3
#calculate the doublet strength
k = 2*np.pi*U_inf*R**2
gamma = F_g_per_depth / (rho*U_inf)
v_theta = gamma/ (2 * np.pi * R)
omega = v_theta / R
s = 60*omega / (2*np.pi)
Next we build up the velocity field using the three elementary flows. We can add together the velocity
contributions from each of them.
x = np.linspace(-0.2,0.2,100)
y = x
xx,yy = np.meshgrid(x,y)
theta = np.linspace(0,2*np.pi,100)
ball_shape_x = R*np.cos(theta)
ball_shape_y = R*np.sin(theta)
u_freestream, v_freestream = get_velocity_freestream(U_inf,0,xx,yy, dim=2)
u_vortex, v_vortex = get_velocity_vortex(gamma,x_center,y_center,xx,yy)
u_doublet, v_doublet = get_velocity_doublet(k,x_center,y_center,xx,yy)
plt.figure(2, figsize= (4,4))
u = u_freestream + u_doublet + u_vortex
v = v_freestream + v_doublet + v_vortex
plt.streamplot(xx, yy, u, v,density=.9, linewidth=1, arrowsize=1.5, arrowstyle=’->’, broken_streaml
plt.plot(ball_shape_x, ball_shape_y)
plt.xlabel(’x (m)’)
plt.ylabel(’y (m)’)
plt.show()
3
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
As a sanity check, you can verify that
v
r
= 0 on the surface of the baseball.
v
r
=
xu
+
yv
p
x
2
+
y
2
u_freestream, v_freestream = get_velocity_freestream(U_inf,0,ball_shape_x, ball_shape_y, dim=1)
u_vortex, v_vortex = get_velocity_vortex(gamma, x_center, y_center, ball_shape_x, ball_shape_y)
u_doublet, v_doublet = get_velocity_doublet(k, x_center, y_center, ball_shape_x, ball_shape_y)
u = u_freestream + u_doublet + u_vortex
v = v_freestream + v_doublet + v_vortex
v_r = (ball_shape_x * u + ball_shape_y*v) / (np.sqrt(ball_shape_x**2 + ball_shape_y**2))
v_theta = (ball_shape_x*v - ball_shape_y*u) / np.sqrt(ball_shape_x**2 + ball_shape_y**2)
plt.figure(0)
plt.plot(theta, v_r)
plt.ylim([-1,1])
plt.xlabel(’Angle (radians)’)
plt.ylabel(’Raidal Velocity’)
plt.savefig(’5_1_vr’)
plt.show()
4
Now to calculate the coefficient of pressure use the following equation:
C
p
= 1
−
(
U
U
inf
)
2
Because
v
r
= 0 on the surface of the ball,
U
=
v
θ
, which was calculated in the previous code block.
5
2. Here we will solve the problem numerically. We will build off of the code for the previous question,
specifically using the same functions for the elementary flow fields.
U_inf = 40.2 # m/s
x_center = 0
y_center = 0
R = 0.037
P_inf = 101325
F_g = 9.8 * 0.15
F_g_per_depth = F_g / (2*R) # N/m
L_freestream_per_depth = F_g_per_depth
rho = 1.225
#calculate the doublet strength
k = 2*np.pi*U_inf*R**2
#calculate vortex strength
gamma = L_freestream_per_depth / (0.02*rho*U_inf)
Next we will iterate over many different heights and calculate the lift force per unit depth if the ball
is located at that height.
We will start with small heights, and check to see if the lift satisfies our
conditions. As soon as we calculate a lift that is with 1% of the freestream
L
′
, that is the numerical
solution for the smallest height we can use. If you smaller increments between each
h
that you test,
then you will find a more accurate answer.
As part of this, we also numerically integrate the pressure distribution over the surface by calculating
the pressure at a discrete number of points, and adding together the vertical contribution from each
of them.
L_list = []
h_list = np.linspace(0.05, 0.5, 10000)
theta, dtheta = np.linspace(0,2*np.pi,100, endpoint=False, retstep=True)
ball_shape_x = R*np.cos(theta)
ball_shape_y = R*np.sin(theta)
found_min = 0
for h in h_list:
x = np.linspace(-3*h,3*h,101)
y = np.linspace(-3*h,3*h,101)
xx,yy = np.meshgrid(x,y)
# (0,0) is at the center of the ball, meaning the wall is at y = -h
# and the image is at (0, -2h)
lower_wall = -h
lower_image_y = -2*h
#calculate velocity along the surface of the ball
#freestream velocity
u_freestream, v_freestream = get_velocity_freestream(U_inf,0,ball_shape_x,ball_shape_y, dim=1)
# velocity due to the vortex and doublet representing the ball
u_vortex, v_vortex = get_velocity_vortex(gamma,x_center,y_center,ball_shape_x,ball_shape_y)
u_doublet, v_doublet = get_velocity_doublet(k,x_center,y_center,ball_shape_x,ball_shape_y)
# velocity due to the vortex and doublet representing the image
6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
u_vortex_lower, v_vortex_lower = get_velocity_vortex(-gamma,x_center,lower_image_y,ball_shape_x
u_doublet_lower, v_doublet_lower = get_velocity_doublet(k,x_center,lower_image_y,ball_shape_x,b
# add all velocity components together and calculate the magnitude
u = u_freestream + u_doublet + u_vortex + u_vortex_lower + u_doublet_lower
v = v_freestream + v_doublet + v_vortex + v_vortex_lower + v_doublet_lower
V_mag = np.sqrt(u**2 + v**2)
# calculate the pressure coefficient
C_p = 1 - (V_mag/U_inf)**2
# calculate the pressure
P = C_p * 1/2 * rho * U_inf**2 + P_inf
# calculate the lift per depth and store the result
L = np.sum(-P*np.sin(theta) * R * dtheta)
L_list.append(L)
# check if this Lift is within 1% of the free stream lift
if np.abs(L-L_freestream_per_depth)/L_freestream_per_depth < 0.01 and not found_min:
print(f’Minimum height is {h:0.4f} m’)
found_min = True
min_h = h
# sanity check to make sure there’s no flow through the wall
y_lower = np.ones(101)*lower_wall
_, v_freestream = get_velocity_freestream(U_inf,0,x,y_lower, dim=1)
_, v_vortex = get_velocity_vortex(gamma,x_center,y_center,x,y_lower)
_, v_doublet = get_velocity_doublet(k,x_center,y_center,x,y_lower)
_, v_vortex_lower = get_velocity_vortex(-gamma,x_center,lower_image_y,x,y_lower)
_, v_doublet_lower = get_velocity_doublet(k,x_center,lower_image_y,x,y_lower)
v_wall = v_freestream + v_doublet + v_vortex + v_vortex_lower + v_doublet_lower
# raise an error if there is flow through the wall
eps = 1.e-10
if (np.abs(v_wall) > eps).any():
print(’Flow through wall’)
print(v_wall)
Using this code we calculate a minimum height of approximately 0.21 m.
Next we will plot the relationship between height and lift. I’ve added an orange line to represent 1%
less than the freestream lift.
plt.figure(0)
plt.plot(h_list, L_list)
plt.plot([h_list.min(), h_list.max()], [L_freestream_per_depth*0.99, L_freestream_per_depth*0.99])
7
plt.xlabel(’Height (m)’)
plt.ylabel("L’ (N/m)")
plt.savefig(’5_2_lift’)
plt.show()
Lastly, we will plot the streamlines of the flow at this minimum height.
I’ve added a black line to
represent the wall, and an orange circle to represent the ball.
h = min_h
x = np.linspace(-3*h,3*h,101)
y = np.linspace(-3*h,3*h,101)
xx,yy = np.meshgrid(x,y)
lower_wall = -h
lower_image_y = -2*h
#calculate velocity along the surface of the ball
u_freestream, v_freestream = get_velocity_freestream(U_inf,0,xx,yy, dim=2)
u_vortex, v_vortex = get_velocity_vortex(gamma,x_center,y_center,xx,yy)
u_doublet, v_doublet = get_velocity_doublet(k,x_center,y_center,xx,yy)
u_vortex_lower, v_vortex_lower = get_velocity_vortex(-gamma,x_center,lower_image_y,xx,yy)
u_doublet_lower, v_doublet_lower = get_velocity_doublet(k,x_center,lower_image_y,xx,yy)
u = u_freestream + u_doublet + u_vortex + u_vortex_lower + u_doublet_lower
v = v_freestream + v_doublet + v_vortex + v_vortex_lower + v_doublet_lower
plt.figure(2, figsize= (4,4))
plt.streamplot(xx, yy, u, v,density=0.8, linewidth=1, arrowsize=1.5,
arrowstyle=’->’, broken_streamlines=False)
plt.plot(ball_shape_x, ball_shape_y)
plt.plot([-3*h,3*h],[-h,-h], ’k’)
plt.show()
8
Alternatively, if you wanted to solve the problem analytically, these are the first few steps you would
take. We will set up the problem in the same way as in the code, building up a velocity field from the
5 different elementary flows. The ball will be centered at (0,0).
Here is the terms for the freestream flow.
u
freestream
=
U
inf
v
freestream
= 0
Here are the terms for the ball.
u
doublet,ball
=
−
k
2
π
x
2
−
y
2
x
2
+
y
2
v
doublet,ball
=
−
k
2
π
2
xy
x
2
+
y
2
u
vortex,ball
=
Γ
2
π
y
x
2
+
y
2
v
vortex,ball
=
−
Γ
2
π
x
x
2
+
y
2
Here are the terms for the image of the ball, at (0
,
−
2
h
). We flip the direction of the vortex to mirror
9
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
the ball.
u
doublet,image
=
−
k
2
π
x
2
−
(
y
+ 2
h
)
2
x
2
+ (
y
+ 2
h
)
2
v
doublet,image
=
−
k
2
π
2
x
(
y
+ 2
h
)
x
2
+ (
y
+ 2
h
)
2
u
vortex,image
=
−
Γ
2
π
y
+ 2
h
x
2
+ (
y
+ 2
h
)
2
v
vortex,image
=
Γ
2
π
x
x
2
+ (
y
+ 2
h
)
2
Adding these flows together:
u
=
u
freestream
+
u
doublet,ball
+
u
vortex,ball
+
u
doublet,image
+
u
vortex,image
v
=
v
freestream
+
v
doublet,ball
+
v
vortex,ball
+
v
doublet,image
+
v
vortex,image
Next we would plug in all the variables we know, and leave
h
as an unknown. Then we would calculate
the magnitude of the velocity, and use that to find the pressure distribution around the surface of the
ball. That expression could then be integrated to and set equal to our desired lift. At that point we
would be able to solve for the exact minimum height required.
10
Related Documents
Related Questions
Q6. For a point at distance 50 m and angle
450 to the axis which of the following are
correct?
Consider an infinite baffled piston of radius 5 cm driven at 2 kHz in
air with velocity 10 m/s.
You may choose multiple options.
A. k = 18.32 and J1(ka sin(q))/ka sin(q) = 0.17
B. k = 36.64 and J1(ka sin(q))/ka sin(q) = 0.40
C.k=5.83 and J1(ka sin(q))/ka sin(q) = 3.16
D. None of the above
Submit
E
arrow_forward
3.
y
1127
L
THE FIGURE IS NOT DRAWN TO SCALE. Consider the cross-sectional area in the xy plane. The parameters are h = 40 mm, a = 36 mm and L = 126 mm.
arrow_forward
I have Euler parameters that describe the orientation of N relative to Q, e = -0.7071*n3, e4 = 0.7071. I have Euler parameters that describe the orientation of U relative to N, e = -1/sqrt(3)*n1, e4 = sqrt(2/3). After using euler parameter rule of successive rotations, I get euler parameters that describe the orientation of U relative to Q, e = -0.4082*n1 - 0.4082*n2 - 0.5774*n3. I need euler parameters that describe the orientation of U relative to Q in vector basis of q instead of n. How do I get that?
arrow_forward
Make a reorientation maneuver to a stable attitude. The spacecraft has the inertia J:
6400 -76.4 -25.6]
J-76.4 4730
-40 kg-m²
-25.6 -40 8160
(2)
The initial quaterion is w(to) = √√2/2[10017], the initial angular velocity is. The desired
quaternion is the unit quaternion. The initial wheel momentum is w(to) = [0.01 0.01 0.017]
rad/s. The gains are set to kp = 10 and kd = 150.
(a) compare two different suitable control laws. Justify your choices.
arrow_forward
Make a reorientation maneuver to a stable attitude. The spacecraft has the inertia J:
6400 -76.4 -25.6]
J-76.4 4730 -40 kg-m²
-25.6 -40 8160
(2)
The initial quaterion is w(to) = √√2/2[10017], the initial angular velocity is. The desired
quaternion is the unit quaternion. The initial wheel momentum is w(to) = [0.01 0.01 0.017]
rad/s. The gains are set to kp = 10 and k₁ = 150.
(a) compare two different suitable control laws. Justify your choices.
(b) compare two different wheel configurations, compare the pseudo-inverse with the
minimax law in terms of wheel momenta and wheel speeds (state your assumptions).
arrow_forward
answer full process
arrow_forward
Find the natural frequency (in Hertz) of the system illustrated in the following figure. Assume the disk is rolling without slipping. K1 = 4 MN/m, K2 = 2K1, M = 165 kg, and R =
0.2 m. Assume the moment of inertia is A
K,
R.
K2
www
M
No Slip
220.19 Hz
35.04 Hz
25.58 Hz
17.94 Hz
71.49 Hz
O 0 O O O
arrow_forward
Develop the Weber number by starting with estimates for the inertia and surface tension forces. p is density, Vis velocity, I is length,
and o is a surface temsion.
X Your answer is incorrect.
Inertia force ~
O pV²1
O pVI
0 pv ²1²
arrow_forward
Suppose the optimum spacing of
wind turbines is estimated to be 4
rotor diameters between wind
turbines within a row and 7
diameters between rows. Estimate
the total area onshore required
constructing a farm of thousand
turbines of diameter 100 m each
built in 20 rows.
wind
4 diameters
7 diameters
arrow_forward
Wng
Consider systems A and B shown in the figure. The ratio of natural frequency of system A(wn,) to natural frequency of system B(wng), i.e. 4 is: (for the disc, assume moment
of inertia about its centre is
Assume g = 9.81 m/s?
juiny
m
m.
g = 9.81 m/s?
A
a
frictionless
No slip
V3
о ООО о
arrow_forward
I need help writing a code in MATLAB. Please help me with question b.6
arrow_forward
Figure below shows an aircraft fighter. The wing of the aircraft may be modelled as a clamped wing with
two lump masses at its centre and tip. The two masses represent the mass of the wing and the missiles.
The equivalent model shown in the figure is used to estimate the first two natural frequencies of the
wing and therefore the fuselage can be assumed rigid.
24EI
If m1 = am, m2 = bm and bending stiffnesses of the beams are k
where a = 6.3005 and b =
13
%3D
5.2174 and if w1 and w2 are the first and second natural frequencies of the system, w2/w1 is:
x, (t)
x2(t)
EI
m,
EI
(m,)
1/2
1/2
3.4053
4.9188
2.5225
1.3621
1.8414
arrow_forward
Please just question C. Instead of solving part C how it says can you solve by using k1=k2=1N/m m1=m2=1kg, l=1m, g=10m/s^2, use [X,wn2]=eig(K,M) to find the natural frequencies for both sets of coordinates. Thank you
arrow_forward
please show all work for parts A-C. :)
arrow_forward
I am using eq 3.117 in Shigley's 10ed:
m*x-double dot+b*x-dot+k*x=(1/2)C_x*(e_c)^2
Since x-double dot=X-dot=0, equations becomes:
k*x=(1/2)C_x*(e_c)^2
then plug in eq 3.110, C_x=(n*E_0*w)/d
Then solve for x. Does this look correct?
arrow_forward
Direction: Answer the following, make sure to show your complete solution and box your finals answers. Make sure it is readable!!!
arrow_forward
SOLVE IN DIGITAL FORMAT STEP BY STEP
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
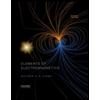
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
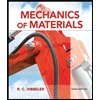
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
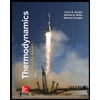
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
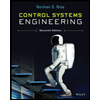
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
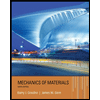
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
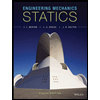
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- Q6. For a point at distance 50 m and angle 450 to the axis which of the following are correct? Consider an infinite baffled piston of radius 5 cm driven at 2 kHz in air with velocity 10 m/s. You may choose multiple options. A. k = 18.32 and J1(ka sin(q))/ka sin(q) = 0.17 B. k = 36.64 and J1(ka sin(q))/ka sin(q) = 0.40 C.k=5.83 and J1(ka sin(q))/ka sin(q) = 3.16 D. None of the above Submit Earrow_forward3. y 1127 L THE FIGURE IS NOT DRAWN TO SCALE. Consider the cross-sectional area in the xy plane. The parameters are h = 40 mm, a = 36 mm and L = 126 mm.arrow_forwardI have Euler parameters that describe the orientation of N relative to Q, e = -0.7071*n3, e4 = 0.7071. I have Euler parameters that describe the orientation of U relative to N, e = -1/sqrt(3)*n1, e4 = sqrt(2/3). After using euler parameter rule of successive rotations, I get euler parameters that describe the orientation of U relative to Q, e = -0.4082*n1 - 0.4082*n2 - 0.5774*n3. I need euler parameters that describe the orientation of U relative to Q in vector basis of q instead of n. How do I get that?arrow_forward
- Make a reorientation maneuver to a stable attitude. The spacecraft has the inertia J: 6400 -76.4 -25.6] J-76.4 4730 -40 kg-m² -25.6 -40 8160 (2) The initial quaterion is w(to) = √√2/2[10017], the initial angular velocity is. The desired quaternion is the unit quaternion. The initial wheel momentum is w(to) = [0.01 0.01 0.017] rad/s. The gains are set to kp = 10 and kd = 150. (a) compare two different suitable control laws. Justify your choices.arrow_forwardMake a reorientation maneuver to a stable attitude. The spacecraft has the inertia J: 6400 -76.4 -25.6] J-76.4 4730 -40 kg-m² -25.6 -40 8160 (2) The initial quaterion is w(to) = √√2/2[10017], the initial angular velocity is. The desired quaternion is the unit quaternion. The initial wheel momentum is w(to) = [0.01 0.01 0.017] rad/s. The gains are set to kp = 10 and k₁ = 150. (a) compare two different suitable control laws. Justify your choices. (b) compare two different wheel configurations, compare the pseudo-inverse with the minimax law in terms of wheel momenta and wheel speeds (state your assumptions).arrow_forwardanswer full processarrow_forward
- Find the natural frequency (in Hertz) of the system illustrated in the following figure. Assume the disk is rolling without slipping. K1 = 4 MN/m, K2 = 2K1, M = 165 kg, and R = 0.2 m. Assume the moment of inertia is A K, R. K2 www M No Slip 220.19 Hz 35.04 Hz 25.58 Hz 17.94 Hz 71.49 Hz O 0 O O Oarrow_forwardDevelop the Weber number by starting with estimates for the inertia and surface tension forces. p is density, Vis velocity, I is length, and o is a surface temsion. X Your answer is incorrect. Inertia force ~ O pV²1 O pVI 0 pv ²1²arrow_forwardSuppose the optimum spacing of wind turbines is estimated to be 4 rotor diameters between wind turbines within a row and 7 diameters between rows. Estimate the total area onshore required constructing a farm of thousand turbines of diameter 100 m each built in 20 rows. wind 4 diameters 7 diametersarrow_forward
- Wng Consider systems A and B shown in the figure. The ratio of natural frequency of system A(wn,) to natural frequency of system B(wng), i.e. 4 is: (for the disc, assume moment of inertia about its centre is Assume g = 9.81 m/s? juiny m m. g = 9.81 m/s? A a frictionless No slip V3 о ООО оarrow_forwardI need help writing a code in MATLAB. Please help me with question b.6arrow_forwardFigure below shows an aircraft fighter. The wing of the aircraft may be modelled as a clamped wing with two lump masses at its centre and tip. The two masses represent the mass of the wing and the missiles. The equivalent model shown in the figure is used to estimate the first two natural frequencies of the wing and therefore the fuselage can be assumed rigid. 24EI If m1 = am, m2 = bm and bending stiffnesses of the beams are k where a = 6.3005 and b = 13 %3D 5.2174 and if w1 and w2 are the first and second natural frequencies of the system, w2/w1 is: x, (t) x2(t) EI m, EI (m,) 1/2 1/2 3.4053 4.9188 2.5225 1.3621 1.8414arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
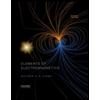
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
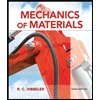
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
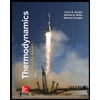
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
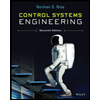
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
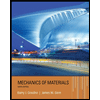
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
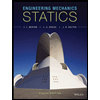
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY