Classes
pdf
School
Toronto Metropolitan University *
*We aren’t endorsed by this school
Course
109
Subject
Computer Science
Date
Dec 6, 2023
Type
Pages
15
Uploaded by ramabarham18
#################
## EXAMPLE: everything
#################
import
import
unittest
unittest
'''
Completing this class rundown should mean that you are pretty well-prepared for the
upcoming exam questions (or at least most of them) on classes.
Generally, concepts you should be familiar with are:
1. Creating an "__init__" method for your class
(Including how to make default variables within your class)
2. Accessing, modifying, and defining variables in the class
3. Creating instance methods within your class
4. How to use helper methods alongside classes
This question will get you to do all of the above, and I'll identify the relevant
sections so you can practice what you need specifically. I'll also be going over
the solution to this during the Review Session on Sunday December 5th at 10 am,
and I'll post the solutions on the Discord too!
'''
'''
Story: The Teletubbies are vanquished, but in the realm of dreams they still haunt
you! Your task is to implement the following classes and helper methods according
to the requirements described in each class/method, so as to conquer your nightmares,
and obliterate all traces of the Teletubbies!
'''
class
class
Dream
Dream
:
'''
Dreams are pretty good. Usually with apple pies and candies and all that good stuff.
Your task is as follows:
Construct the "__init__" method for a Dream class, that takes in 2 arguments (plus self),
self (which you ALWAYS require in your constructors, even if there is nothing else in them!)
a string "title", and
an int "length"
And sets a list dream_elements to start as the empty list: []
[1]
Then, once you have completed your constructor, create an instance method called
change_title(a), which changes the title of your dream instance to the string a.
[2, 3]
Then, create another instance method called add_to_dream(topic), which adds the string
topic to the dream_elements list.
[2, 3]
Finally, create an instance method dreams_to_dust(), which replaces each element of the
dream_elements list with the string "dust".
[2, 3]
'''
def
def
__init__
(
self
, title, length, dream_elements
=
[]):
self
.
title
=
title
self
.
length
=
int
(length)
self
.
dream_elements
=
[]
def
def
change_title
(
self
, a):
self
.
title
=
a
def
def
add_to_dream
(
self
, topic):
# self.topic = topic
self
.
dream_elements
.
append(topic)
def
def
dreams_to_dust
(
self
):
for
for
i
in
in
range
(
len
(
self
.
dream_elements)):
self
.
dream_elements[i]
=
'dust'
class
class
Nightmare
Nightmare
(Dream):
# =============================================================================
# '''
# Nightmares are pretty bad. Usually with Teletubbies and chainsaws and all that bad stuff.
# Your task is as follows:
# Construct the "__init__" method for a Nightmare class, that takes in 0 arguments (except
self of course)!
# However, you should create an instance variable "topic" that defaults to the string
# "Teletubbies", and an instance variable for an attached Dream that the nightmare is
# in, called "attached_dream" (which defaults to None).
# [1]
# Then, create a method attach_to_dream(d), which attached your nightmare instance
# to a dream via setting attached_dream to the argument Dream d.
# [2, 3]
# Then, create a method has_attached_dream() which returns True if your attached_dream
# is not None, and False otherwise.
# [2, 3]
# Finally, create a method WAKE_ME_UP(), which will set the attached_dream's length to 0,
# and call it's dreams_to_dust() method.
# [2, 3]
# '''
# =============================================================================
def
def
__init__
(
self
):
self
.
topic
=
"Teletubbies"
self
.
attached_dream
=
None
def
def
attach_to_dream
(
self
, d):
self
.
attached_dream
=
d
def
def
has_attached_dream
(
self
):
return
return self
.
attached_dream
!=
None
def
def
WAKE_ME_UP
(
self
):
if
if
(
self
.
has_attached_dream()):
self
.
attached_dream
.
length
= 0
self
.
attached_dream
.
dreams_to_dust()
def
def
return_titles
(list_of_dreams):
to_return_list
=
[]
for
for
dream
in
in
list_of_dreams:
to_return_list
.
append(dream
.
title)
return
return
to_return_list
def
def
WAKE_ME_UP_INSIDE
(cant_wake_up):
for
for
i
in
in
cant_wake_up:
i
.
WAKE_ME_UP()
def
def
how_long_are_my_dreams
(list_of_dreams):
summ
= 0
for
for
j
in
in
list_of_dreams:
summ
+=
j
.
length
return
return
summ
def
def
__str__
(
self
):
print
print
(return_titles())
# --------------------------------------------------------------
# The Testing
# --------------------------------------------------------------
class
class
myTests
myTests
(unittest
.
TestCase):
def
def
test1
(
self
):
# Testing Dream constructor
d
=
Dream(
"my awesome dream"
,
5
)
self
.
assertEqual(d
.
title,
"my awesome dream"
)
self
.
assertEqual(d
.
length,
5
)
self
.
assertEqual(d
.
dream_elements, [])
def
def
test2
(
self
):
# Testing nightmare constructor
n
=
Nightmare()
self
.
assertEqual(n
.
topic,
"Teletubbies"
)
self
.
assertEqual(n
.
attached_dream,
None
)
def
def
test3
(
self
):
# Testing Dream.change_title()
d
=
Dream(
"my awesome dream"
,
5
)
d
.
change_title(
"my great dream"
)
self
.
assertEqual(d
.
title,
"my great dream"
)
def
def
test4
(
self
):
# Testing Dream.add_to_dream()
d
=
Dream(
"my awesome dream"
,
5
)
d
.
change_title(
"my great dream"
)
d
.
add_to_dream(
"rainbows"
)
d
.
add_to_dream(
"butterflies"
)
self
.
assertEqual(d
.
dream_elements, [
"rainbows"
,
"butterflies"
])
def
def
test5
(
self
):
# Testing Dream.dreams_to_dust()
d
=
Dream(
"my ruined dream"
,
5
)
d
.
add_to_dream(
"rainbows"
)
d
.
add_to_dream(
"butterflies"
)
d
.
add_to_dream(
"horsies"
)
d
.
dreams_to_dust()
self
.
assertEqual(d
.
dream_elements, [
"dust"
,
"dust"
,
"dust"
])
def
def
test6
(
self
):
# Testing Nightmare.attach_to_dream()
n
=
Nightmare()
d
=
Dream(
"my awesome dream"
,
5
)
n
.
attach_to_dream(d)
self
.
assertEqual(n
.
attached_dream, d)
def
def
test7
(
self
):
n
=
Nightmare()
d
=
Dream(
"my awesome dream"
,
5
)
self
.
assertEqual(n
.
has_attached_dream(),
False
)
n
.
attach_to_dream(d)
self
.
assertEqual(n
.
has_attached_dream(),
True
)
def
def
test8
(
self
):
# Testing Nightmare.WAKE_ME_UP()
n
=
Nightmare()
d
=
Dream(
"my awesome dream"
,
5
)
d
.
add_to_dream(
"pumpkins"
)
n
.
attach_to_dream(d)
n
.
WAKE_ME_UP()
self
.
assertEqual(d
.
length,
0
)
self
.
assertEqual(d
.
dream_elements, [
"dust"
])
def
def
test9
(
self
):
# Testing return_titles()
d1
=
Dream(
"my awesome dream!"
,
5
)
d2
=
Dream(
"my fantastic dream!!"
,
4
)
d3
=
Dream(
"my superb dream!!!"
,
3
)
self
.
assertEqual(return_titles([d1, d2, d3]), [
"my awesome dream!"
,
"my fantastic dream!!"
,
"my superb dream!!!"
])
def
def
test10
(
self
):
# Testing WAKE_ME_UP_INSIDE()
d1
=
Dream(
"my awesome dream!"
,
5
)
d1
.
add_to_dream(
"sprinkles"
)
d2
=
Dream(
"my fantastic dream!!"
,
4
)
d2
.
add_to_dream(
"cake"
)
d2
.
add_to_dream(
"chocolates"
)
n1
=
Nightmare()
n2
=
Nightmare()
n1
.
attach_to_dream(d1)
n2
.
attach_to_dream(d2)
WAKE_ME_UP_INSIDE([n1, n2])
self
.
assertEqual(d1
.
length,
0
)
self
.
assertEqual(d2
.
length,
0
)
self
.
assertEqual(d1
.
dream_elements, [
"dust"
])
self
.
assertEqual(d2
.
dream_elements, [
"dust"
,
"dust"
])
def
def
test11
(
self
):
# Testing how_long_are_my_dreams()
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
d1
=
Dream(
"my awesome dream!"
,
5
)
d2
=
Dream(
"my fantastic dream!!"
,
4
)
d3
=
Dream(
"my superb dream!!!"
,
3
)
self
.
assertEqual(how_long_are_my_dreams([d1, d2, d3]),
12
)
if
if
__name__
==
'__main__'
:
unittest
.
main(
exit
=
True
)
#################
## EXAMPLE: inheritance
#################
'''
Here is the implementation of a parent class called
Animal. As you can see, it has the constructor (that __init__)
already implemented, as well as a member method. Implement
the __eq__ method for animal such that Python returns True if
the name and age of both of the animals being compared are identical.
You are to write a child class of Animal called Cat.
Add another member variable to the Cat class called
fur_colour, eye_colour and is_brushed. is_brushed should
be automatically set to False. Add another class function
called brushies. This function will set the is_brushed
member variable to true and print a cat face to the
screen (^._.^)
Finally, finish the __eq__ function for cat, such that it
also compared the other member variables of self to other.
Make two Cat objects in the main and call your member
method for Brushies on one. Then, compare them and print the
result.
'''
class
class
Animal
Animal
():
def
def
__init__
(
self
, name, age):
self
.
name
=
name
self
.
age
=
age
def
def
__eq__
(
self
, other):
return
return
(
self
.
name
==
other
.
name)
and
and
(
self
.
age
==
other
.
age)
def
def
take_for_walk
(
self
):
print
print
(f
"Your {self.name} has gone for a walk!"
)
'''
Put your cat class here.
'''
class
class
Cat
Cat
(Animal):
def
def
__init__
(
self
, name, age, fur_colour, eye_colour, is_brushed):
Animal
.
__init__
(
self
, name, age)
self
.
fc
=
fur_colour
self
.
ec
=
eye_colour
self
.
ib
=
is_brushed
def
def
brushies
(
self
):
if
if self
.
ib
==
True
:
print
print
(
'(^._.^)'
)
def
def
__eq__
(
self
, other):
return
return self
.
ib
==
other
.
ib
if
if
__name__
==
"__main__"
:
c1
=
Cat(
'Theo'
,
2
,
'black'
,
'green'
,
True
)
c2
=
Cat(
'Mooshi'
,
5
,
'white'
,
'black'
,
False
)
c1
.
brushies()
c2
.
brushies()
print
print
(c1
.
__eq__
(c2))
#################
## EXAMPLE: simple Coordinate class
#################
class
class
Coordinate
Coordinate
(
object
):
""" A coordinate made up of an x and y value """
def
def
__init__
(
self
, x, y):
""" Sets the x and y values """
self
.
x
=
x
self
.
y
=
y
def
def
__str__
(
self
):
""" Returns a string representation of self """
return
return
"<"
+
str
(
self
.
x)
+
","
+
str
(
self
.
y)
+
">"
def
def
distance
(
self
, other):
""" Returns the euclidean distance between two points """
x_diff_sq
=
(
self
.
x
-
other
.
x)
**2
y_diff_sq
=
(
self
.
y
-
other
.
y)
**2
return
return
(x_diff_sq
+
y_diff_sq)
**0.5
c
=
Coordinate(
3
,
4
)
origin
=
Coordinate(
0
,
0
)
print
print
(c
.
x, origin
.
x)
print
print
(c
.
distance(origin))
print
print
(Coordinate
.
distance(c, origin))
print
print
(origin
.
distance(c))
print
print
(c)
#################
## EXAMPLE: simple class to represent fractions
## Try adding more built-in operations like multiply, divide
### Try adding a reduce method to reduce the fraction (use gcd)
#################
class
class
Fraction
Fraction
(
object
):
"""
A number represented as a fraction
"""
def
def
__init__
(
self
, num, denom):
""" num and denom are integers """
assert
assert type
(num)
==
int
and
and
type
(denom)
==
int
,
"ints not used"
self
.
num
=
num
self
.
denom
=
denom
def
def
__str__
(
self
):
""" Retunrs a string representation of self """
return
return str
(
self
.
num)
+
"/"
+
str
(
self
.
denom)
def
def
__add__
(
self
, other):
""" Returns a new fraction representing the addition """
top
=
self
.
num
*
other
.
denom
+
self
.
denom
*
other
.
num
bott
=
self
.
denom
*
other
.
denom
return
return
Fraction(top, bott)
def
def
__sub__
(
self
, other):
""" Returns a new fraction representing the subtraction """
top
=
self
.
num
*
other
.
denom
-
self
.
denom
*
other
.
num
bott
=
self
.
denom
*
other
.
denom
return
return
Fraction(top, bott)
def
def
__float__
(
self
):
""" Returns a float value of the fraction """
return
return self
.
num
/
self
.
denom
def
def
inverse
(
self
):
""" Returns a new fraction representing 1/self """
return
return
Fraction(
self
.
denom,
self
.
num)
a
=
Fraction(
1
,
4
)
b
=
Fraction(
3
,
4
)
c
=
a
+
b
# c is a Fraction object
print
print
(c)
print
print
(
float
(c))
print
print
(Fraction
.
__float__
(c))
print
print
(
float
(b
.
inverse()))
##c = Fraction(3.14, 2.7) # assertion error
##print a*b # error, did not define how to multiply two Fraction objects
##############
## EXAMPLE: a set of integers as class
##############
class
class
intSet
intSet
(
object
):
"""
An intSet is a set of integers
The value is represented by a list of ints, self.vals
Each int in the set occurs in self.vals exactly once
"""
def
def
__init__
(
self
):
""" Create an empty set of integers """
self
.
vals
=
[]
def
def
insert
(
self
, e):
""" Assumes e is an integer and inserts e into self """
if
if
not
not
e
in
in
self
.
vals:
self
.
vals
.
append(e)
def
def
member
(
self
, e):
""" Assumes e is an integer
Returns True if e is in self, and False otherwise """
return
return
e
in
in
self
.
vals
def
def
remove
(
self
, e):
""" Assumes e is an integer and removes e from self
Raises ValueError if e is not in self """
try
try
:
self
.
vals
.
remove(e)
except
except
:
raise
raise
ValueError
ValueError
(
str
(e)
+
' not found'
)
def
def
getMembers
(
self
):
"""Returns a list containing the elements of self.
Nothing can be assumed about the order of the elements."""
return
return self
.
vals[:]
def
def
__str__
(
self
):
""" Returns a string representation of self """
self
.
vals
.
sort()
return
return
'{'
+
','
.
join([
str
(e)
for
for
e
in
in
self
.
vals])
+
'}'
# for e in self.vals:
# result = result + str(e) + ','
# return '{' + result[:-1] + '}' # -1 omits trailing comma
# Instantiation is used to create instances of the class.
# For exampl, the following statement creates a new object of type IntSet.
# s is called an instance of IntSet.
s
=
intSet()
print
print
(s)
s
.
insert(
3
)
s
.
insert(
4
)
s
.
insert(
3
)
print
print
(s)
# Attribute references: use dot notation to access attributes associated with the class.
# For example, s.member refers to the method member associated with the instance s of type
IntSet
s
.
member(
3
)
s
.
member(
5
)
s
.
insert(
6
)
print
print
(s)
#s.remove(5) # leads to an error
print
print
(s)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
s
.
remove(
3
)
##############
## EXAMPLE: class Person
##############
import
import
datetime
datetime
class
class
Person
Person
(
object
):
"""
Design a class that incorpates some of the common attributes (name and birthdate) of
humans.
It makes use of the standard Python libary module datetime, which provides many convenient
methods for creating and manipulating dates.
"""
def
def
__init__
(
self
, name):
"""Create a person"""
self
.
name
=
name
try
try
:
lastBlank
=
name
.
rindex(
' '
)
self
.
lastName
=
name[lastBlank
+1
:]
except
except
:
self
.
lastName
=
name
self
.
birthday
=
None
def
def
getName
(
self
):
"""Returns self's full name"""
return
return self
.
name
import
import
unittest
unittest
'''This class represents a rectangle in the x-y coordincate system
where the edges of the rectangle are aligned with the x- and y- axes.
A Rectangle object has data attributes lowerleft and upperright,
which are tuples representing the (x, y) coordinates of the lower
left corner and the upper right corner.
Fill in functions for the class.
'''
class
class
Rectangle
Rectangle
():
def
def
__init__
(
self
, x1, y1, x2, y2) :
'''Assumes the (x1, y1) are the coordinates of the lower left corner
and (x2, y2) are the coordinates of the upper right corner.
'''
self
.
lowerleft
=
(x1,y1)
self
.
upperright
=
(x2, y2)
def
def
width
(
self
) :
'''Returns the width of the rectangle
'''
return
return self
.
upperright[
0
]
-
self
.
lowerleft[
0
]
def
def
height
(
self
) :
'''Returns the height of the rectangle
'''
return
return self
.
upperright[
1
]
-
self
.
lowerleft[
1
]
def
def
area
(
self
) :
'''Returns the area of the rectangle'''
w
=
self
.
upperright[
0
]
-
self
.
lowerleft[
0
]
l
=
self
.
upperright[
1
]
-
self
.
lowerleft[
1
]
area
=
w
*
l
return
return
area
def
def
__eq__
(
self
, other) :
'''Returns true if the two rectangles have equal area'''
return
return self
.
area()
==
other
.
area()
#!/usr/bin/python3
import
import
unittest
unittest
# --------------------------------------------------------------e137
# REVIEW: condition, loop, approximation, string, list, dict,
# recursion, 2d matrix, exception, CLASS
# --------------------------------------------------------------
class
class
Rectangle
Rectangle
(
object
) :
'''This class represents a rectangle in the x-y coordincate system
where the edges of the rectangle are aligned with the x- and y- axes.
A Rectangle object has data attributes lowerleft and upperright,
which are tuples representing the (x, y) coordinates of the lower
left corner and the upper right corner.
'''
def
def
__init__
(
self
, x1, y1, x2, y2) :
'''Assumes the (x1, y1) are the coordinates of the lower left corner
and (x2, y2) are the coordinates of the upper right corner.
'''
self
.
x1
=
x1
self
.
x2
=
x2
self
.
y1
=
y1
self
.
y2
=
y2
self
.
lowerleft
=
(x1, y1)
self
.
upperright
=
(x2, y2)
def
def
width
(
self
) :
'''Returns the width of the rectangle
'''
return
return self
.
x2
-
self
.
x1
def
def
height
(
self
) :
'''Returns the height of the rectangle
'''
return
return self
.
y2
-
self
.
y1
def
def
area
(
self
) :
'''Returns the area of the rectangle'''
area
=
self
.
width()
*
self
.
height()
return
return
area
def
def
__eq__
(
self
, other) :
'''Returns true if the two rectangles have equal area'''
return
return self
.
area()
==
other
.
area()
class
class
RecTests
RecTests
(unittest
.
TestCase):
def
def
test1
(
self
):
box1
=
Rectangle(
0
,
0
,
2
,
2
)
self
.
assertEqual(box1
.
width(),
2
)
def
def
test2
(
self
):
box1
=
Rectangle(
0
,
0
,
2
,
2
)
self
.
assertEqual(box1
.
height(),
2
)
def
def
test3
(
self
):
box1
=
Rectangle(
0
,
0
,
2
,
2
)
self
.
assertEqual(box1
.
area(),
4
)
def
def
test4
(
self
):
box1
=
Rectangle(
0
,
0
,
2
,
2
)
box2
=
Rectangle(
2
,
2
,
6
,
3
)
self
.
assertTrue(box1
==
box2)
if
if
__name__
==
'__main__'
:
unittest
.
main(
exit
=
True
)
def
def
getLastName
(
self
):
"""Returns self's last name"""
return
return self
.
lastName
def
def
setBirthday
(
self
, birthdate):
"""Assumes birthdate is of type datetime.date
Sets self's birthday to birthdate"""
self
.
birthday
=
birthdate
def
def
getAge
(
self
):
"""Returns self's current age in days"""
if
if self
.
birthday
==
None
:
raise
raise
ValueError
ValueError
return
return
(datetime
.
date
.
today()
-
self
.
birthday)
.
days
def
def
__lt__
(
self
, other):
"""Returns True if self's name is lexicographically less than other's name, and False
otherwise"""
if
if self
.
lastName
==
other
.
lastName:
return
return self
.
name
<
other
.
name
return
return self
.
lastName
<
other
.
lastName
def
def
__str__
(
self
):
"""Returns self's name"""
return
return self
.
name
me
=
Person(
'Michael Guttag'
)
him
=
Person(
'Barack Hussein Obama'
)
her
=
Person(
'Madonna'
)
print
print
(him
.
getLastName())
him
.
setBirthday(datetime
.
date(
1961
,
8
,
4
))
her
.
setBirthday(datetime
.
date(
1958
,
8
,
16
))
print
print
(him
.
getName(),
'is'
, him
.
getAge(),
'days old'
)
##############
## EXAMPLE: RUPerson for inheritance
##############
class
class
RUPerson
RUPerson
(Person):
"""
Create an inherited subclass called RUPerson from class Person;
add a new attribute getIdNum to get the identification number;
override the special methods if there is a need, such as for _init_ and _It_.
"""
nextIdNum
= 0
#identification number
def
def
__init__
(
self
, name):
"override the _init_ method in the superclass Person"
Person
.
__init__
(
self
, name)
self
.
idNum
=
MITPerson
.
nextIdNum
RUPerson
.
nextIdNum
+= 1
def
def
getIdNum
(
self
):
"return the id number"
return
return self
.
idNum
def
def
__lt__
(
self
, other):
"override the _It_ special method in the superclass Person"
return
return self
.
idNum
<
other
.
idNum
p1
=
RUPerson(
'Barbara Beaver'
)
print
print
(
str
(p1)
+
'
\'
\'
s id number is '
+
str
(p1
.
getIdNum()))
#the \ indicates that the apostrophe is part of the string, not a delimiter terminating the
string
p1
=
RUPerson(
'Mark Guttag'
)
p2
=
RUPerson(
'Billy Bob Beaver'
)
p3
=
RUPerson(
'Billy Bob Beaver'
)
p4
=
Person(
'Billy Bob Beaver'
)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
print
print
(
'p1 < p2 ='
, p1
<
p2)
print
print
(
'p3 < p2 ='
, p3
<
p2)
print
print
(
'p4 < p1 ='
, p4
<
p1)
#the interpreter will print
p1
<
p2
=
True
p3
<
p2
=
False
p4
<
p1
=
True
##############
## EXAMPLE: Two kinds of studetns: multiple levels of inheritance
##############
class
class
Student
Student
(MITPerson):
pass
pass
class
class
UG
UG
(Student):
def
def
__init__
(
self
, name, classYear):
RUPerson
.
__init__
(
self
, name)
self
.
year
=
classYear
def
def
getClass
(
self
):
return
return self
.
year
##############
## EXAMPLE: Radius
##############
#Create a Cricle class and intialize it with radius.
#Make two methods getArea and getCircumference
#inside this class.
class
class
Circle
Circle
:
def
def
__init__
(
self
, radius):
self
.
radius
=
radius
def
def
getArea
(
self
):
return
return
3.14*
self
.
radius
*
self
.
radius
def
def
getCircumference
(
self
):
return
return self
.
radius
*2*3.14
##############
## EXAMPLE: Temprature
##############
class
class
Temprature
Temprature
():
def
def
convertFahrenhiet
(
self
,celsius):
return
return
(celsius
*
(
9/5
))
+32
def
def
convertCelsius
(
self
,farenhiet):
return
return
(farenhiet
-32
)
*
(
5/9
)
# =============================================================================
# Create a Student class and initialize it with name and roll number.
# Make methods to :
# 1. Display - It should display all informations of the student.
# 2. setAge - It should assign age to student
# 3. setMarks - It should assign marks to the student.
# =============================================================================
# =============================================================================
# class Student():
# def __init__(self,name,roll):
# self.name = name
# self.roll= roll
# def display(self):
# print self.name
# print self.roll
# def setAge(self,age):
# self.age=age
# def setMarks(self,marks):
# self.marks = marks
# =============================================================================
class
class
Student
Student
:
def
def
__init__
(
self
, name, rolln, age):
self
.
name
=
name
self
.
rolln
=
rolln
self
.
age
=
age
def
def
display
(
self
):
return
return self
.
name
+
' | '
+
str
(
self
.
rolln)
+
' | '
+
str
(
self
.
age)
def
def
setMarks
(
self
, grade):
self
.
grade
=
grade
return
return self
.
grade
d
=
Student(
'Rama'
,
500909499
,
21
)
print
print
(d
.
display())
print
print
(d
.
setMarks(
4.0
))
import
import
unittest
unittest
# =============================================================================
# Create a Time class and initialize it with hours and minutes.
# 1. Make a method addTime which should take two time object and add them. E.g.- (2 hour and 50
min)+(1 hr and 20 min) is (4 hr and 10 min)
# 2. Make a method displayTime which should print the time.
# 3. Make a method DisplayMinute which should display the total minutes in the Time. E.g.- (1
hr 2 min) should display 62 minute.
# =============================================================================
class
class
Time
Time
:
def
def
__init__
(
self
, hours, minutes):
self
.
hours
=
hours
self
.
minutes
=
minutes
def
def
addTime
(
self
, other):
minute
=
self
.
minutes
+
other
.
minutes
hour
=
self
.
hours
+
other
.
hours
if
if
minute
>=60
:
rem
=
minute
%60
y
=
minute
//60 +
hour
return
return
y, rem
else
else
:
return
return
hour, minute
def
def
DisplayTime
(
self
):
return
return
(f
'Time: {self.hours} hours and {self.minutes} minutes'
)
def
def
DisplayMinute
(
self
):
count
= 60
if
if self
.
hours
== 1
:
return
return
count
+
self
.
minutes
elif
elif self
.
hours
> 1
:
y
=
count
*
self
.
hour
return
return
y
+
self
.
minutes
class
class
TimeTests
TimeTests
(unittest
.
TestCase):
def
def
test1
(
self
):
d
=
Time(
1
,
20
)
d2
=
Time(
2
,
50
)
self
.
assertEqual(d
.
addTime(d2), (
4
,
10
))
def
def
test2
(
self
):
d
=
Time(
1
,
45
)
d2
=
Time(
0
,
15
)
self
.
assertEqual(d
.
addTime(d2), (
2
,
0
))
if
if
__name__
==
'__main__'
:
unittest
.
main(
exit
=
True
)
#!/usr/bin/python3
# ---------------------------------------------------------
# Object Oriented Programming
# ---------------------------------------------------------
# type:
# abstract data type:
# implementation independence, representation independence
# representation invariant
# data attributes
# method attributes
# ---------------------------------------------------------
class
class
intset
intset
(
object
) :
'''An intset is a set of integers'''
# docstring => the abstraction, no detail
description
# Abstract Data Type = ADT = set of values and methods on those values
# Information about the implementation:
# The set of values is represented as a list of ints: self._values,
# each int in the list occurs only once.
def
def
__init__
(
self
) :
# called a constructor
'''Create an empty list of integers'''
self
.
_values
=
[]
def
def
insert
(
self
, element) :
'''Assumes element is an integer
and puts it in the set if not there already'''
if
if
element
not
not in
in
self
.
_values :
self
.
_values
.
append(element)
def
def
remove
(
self
, element) :
'''Assumes element is an integer
and removes it from the set
Raises ValueError if element is not in the set'''
if
if
element
not
not in
in
self
.
_values :
raise
raise
ValueError
ValueError
(f
'{element} is not in the set'
)
self
.
_values
.
remove(element)
pass
pass
def
def
member
(
self
, element) :
'''Returns True if element is in the set, False otherwise'''
return
return
element
in
in
self
.
_values
pass
pass
def
def
getmembers
(
self
) :
'''Returns a copy of the set as a list'''
return
return self
.
_values[:]
pass
pass
def
def
union
(
self
, other) :
'''Mutates self so that it has exactly the elements in both self and other'''
for
for
e
in
in
other :
self
.
insert(e)
pass
pass
def
def
__str__
(
self
) :
'''Sorts and shows the contents in set notation.'''
if
if len
(
self
.
_values)
== 0
:
return
return
'{}'
self
.
_values
.
sort()
result
=
''
for
for
e
in
in
self
.
_values :
result
+=
str
(e)
+
','
return
return
'{'
+
result[:
-1
]
+
'}'
# '{2, 5, 7}'
pass
pass
#def __eq__(self, other) :
# '''Implements =='''
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
# pass
#def __add__(self, other) :
# '''Implements +'''
# pass
#def __sub__(self, other) :
# '''Implements -'''
# pass
# ---------------------------------------------------------
# Try it out
# ---------------------------------------------------------
print
print
(
'---------------------------------------'
)
set
=
intset()
set
.
insert(
3
)
print
print
(
'set after inserting 3:'
,
set
)
set
.
remove(
3
)
print
print
(
'set after removing 3:'
,
set
)
try
try
:
set
.
remove(
3
)
print
print
(
'set after removing 3 again:'
,
set
)
except
except
ValueError
ValueError
as
as
msg :
print
print
(msg)
set1
=
intset()
set1
.
insert(
1
)
set1
.
insert(
2
)
set1
.
insert(
3
)
set2
=
intset()
set2
.
insert(
3
)
set2
.
insert(
2
)
set2
.
insert(
1
)
print
print
(
'set1:'
, set1)
print
print
(
'set2:'
, set2)
print
print
(
'set1 == set2?'
, set1
==
set2)
print
print
(
'set1 == set1?'
, set1
==
set1)
print
print
(
'---------------------------------------'
)
#Output string as set
y
=
self
.
intset
+
other
.
intset
x
=
''
for
for
i
in
in
range
(
len
(y)):
y[i]
=
str
(y[i])
x
=
y[i]
+
', '
+
x
x
=
x
.
rstrip(
', '
)
#if stripping, make sure to store it in value
print
print
(
'{'
+
x
+
'}'
)
# ---------------------------------------------------------
# The End
# ---------------------------------------------------------
import
import
unittest
unittest
class
class
Vehicle
Vehicle
:
def
def
__init__
(
self
, brand, model,
type
):
self
.
brand
=
brand
self
.
model
=
model
self
.
type
=
type
self
.
gas_tank_size
= 14
self
.
fuel_level
= 0
def
def
fuel_up
(
self
):
self
.
fuel_level
=
self
.
gas_tank_size
print
print
(
'Gas tank is now full'
)
def
def
drive
(
self
):
print
print
(f
'The {self.brand} is now driving'
)
C1
=
Vehicle(
'Ford'
,
'explorer'
,
'SUV'
)
print
print
(C1
.
model)
C1
.
model
=
'escape'
print
print
(C1
.
model)
print
print
(C1
.
fuel_level)
C1
.
fuel_level
= 10
print
print
(C1
.
fuel_level)
C1
.
fuel_up()
C1
.
drive()
# -------------------------------------------------------------------------------------
#---------------------------------------------------------------------------------------
# big O = order of complexity
# = given the size of the input to your program, n,
# for example length of a list, n = len(someList)
# how many steps to find the maximum in the list?
def
def
findmax
(L) :
n
=
len
(L)
max
=
L[
0
]
# 1 step
for
for
e
in
in
L :
# n steps
if
if
e
>
max
:
# n steps
max
=
e
# n steps
return
return max
# 1 step
How many steps does this program
?
3
n
+ 2
steps
is
is
our estimate
for
for
the numbers
in
in
big Oh complexity theory you disregard constants, like the
3
or
or
2
O(
3
n
+ 2
)
is
is
written O(n) called linear complexity
if
if
you had two loops nested
for
for
i
in
in
range
(n) :
for
for
j
in
in
range
(n) :
# do something
O(n
**2
) steps
is
is
called quadratic complexity
-----------------------------------------
print
print
(
5*
'hello'
)
O(
5
)
is
is
converted to O(
1
) called constant time program
sorting n elements can be done
in
in
O(n
**2
) like insertion sort
or
or
could O(n log(n))
as
as
in
in
Quicksort
or
or
mergesort
these you later,
in
in
CPS305
for
for
quickest to slowest:
O(
1
), O(n), O(log(n)), O(n
*
log(n)), O(n
**2
), O(n
***3
),
...
, O(
2**
n)
def
def
ispal
(S) :
# base condition
if
if len
(S)
<= 1
:
return
return True
#another base case: 'car' the two ends don't match so return False
if
if
S[
0
]
!=
S[
-1
] :
return
return False
#general case return ispal on the shorter string with the ends
return
return
ispal(S[
1
:
len
(S)
-1
)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Recommended textbooks for you
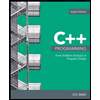
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
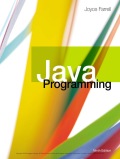
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
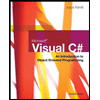
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
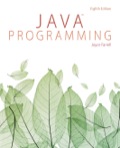
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
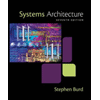
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
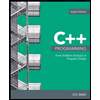
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
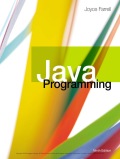
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
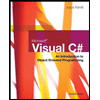
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
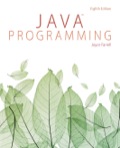
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
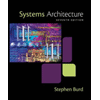
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning