Exam 2 Review
docx
School
Florida International University *
*We aren’t endorsed by this school
Course
3337
Subject
Computer Science
Date
Dec 6, 2023
Type
docx
Pages
15
Uploaded by MinisterBoulderOryx26
Class Activities
1-
Update the attached
code
by adding an interface called "Named" which contains a method called
"getNamed". Make BankAccount, Coin and BritishBankAccount implement the interface.
1.1-
Code provided:
Origin:
src
1(test(folder)/Test(class)) 2(util(folder)/2.1BankAccount(class)-
2.2BritishBankAccount-2.3Coin(class)/2.4Measurable(interface))
Code:
(no changes are going to be made)
package Test;
import util.BankAccount;
import util.BritishBankAccount;
import util.Coin;
import util.Measurable;
import java.util.ArrayList;
public class Test {
public static void main(String[] args){
ArrayList<Measurable> items = new ArrayList<>();
items.add(new BankAccount(100));
items.add(new Coin(.25, "Quarter"));
items.add(new BritishBankAccount(100));
for(Measurable item: items)
System.out.println("This item is a "
+ item.getClass().toString().split("\\.")[1]
+ " and its measurement is " +
item.getMeasure());
}
}
package util;
public class BankAccount implements Measurable{
@Override
public double getMeasure() {
return balance;
}
private double balance;
public double getBalance(){
return balance;
}
public void deposit(double val){
balance += val;
}
public void withdraw(double val){
balance -= val;
}
public BankAccount(double balance){
this.balance = balance;
}
}
(no changes are going to be made because once interface is implemented to bank account this one will inherit it)
package util;
public class BritishBankAccount extends BankAccount{
public BritishBankAccount(double balance) {
super(balance);
}
@Override
public double getMeasure(){
return super.getMeasure() * Measurable.GBP_TO_USD;
}
}
package util;
public class Coin implements Measurable{
private double value;
private String name;
@Override
public double getMeasure() {
return value;
}
public Coin(double value, String name){
this.value = value;
this.name = name;
}
}
package util;
public interface Measurable {
public double getMeasure();
public double INCH_TO_CM = 2.54;
public double GBP_TO_USD = 1.20;
}
1.2 Answer:
Origin:
src
1(test(folder)/Test(class)) 2(util(folder)/2.1BankAccount(class)-
2.2BritishBankAccount-2.3Coin(class)/2.4Measurable(interface)/
Named(interface))
Code:
(Named interface added)
package util;
public interface Named {
String getName();
}
package util;
public class BankAccount implements Measurable,
Named
{
@Override
public double getMeasure() {
return balance;
}
private double balance;
public double getBalance(){
return balance;
}
public void deposit(double val){
balance += val;
}
public void withdraw(double val){
balance -= val;
}
public BankAccount(double balance){
this.balance = balance;
}
private String name;
@Override
public String getName(){
return name;
}
}
package util;
public class Coin implements Measurable,
Named
{
private double value;
private String name;
@Override
public double getMeasure() {
return value;
}
public Coin(double value, String name){
this.value = value;
this.name = name;
}
@Override
public String getName(){
return name;
}
}
2-
Consider the incomplete Java program below. This program first gets a list of students (in
some way that we don't know). Then, it calls Arrays.sort to sort the list of students. Finally, it
prints the sorted list.
Implement the compareTo method in class Student so that the program prints the students
information in the increasing order of gpa. The students with the same gpa should be printed out
in the alphabetical order of their last names. The students with the same gpa and last name
should be printed out in the alphabetical order of their first name. and finally, the students with
the same gpa, first and last names should be printed out in the increasing order of their id.
2.1 Code Provided & 2.2
Answers in Red
import java.util.*;
class Student implements
Comparable(was already implemented on the code)
{
String firstName;
String lastName;
Comparable gpa;
Comparable id;
public String toString(){
return "Student Name is " + firstName + " " + lastName + " with id " + id.toString() + " and GPA " + gpa.toString();
}
public Student(String firstName, String lastName, Comparable gpa, Comparable id){
this.firstName = firstName;
this.lastName = lastName;
this.gpa = gpa;
this.id = id;
}
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
public int compareTo (Object aStudent) {
if(!aStudent.getClass().equals(this.getClass())).
checks that they are the same kind class, object
throw new IllegalArgumentException("can't compare a student with a " + aStudent.getClass());
Student other = (Student) aStudent;
if(this.gpa.compareTo(other.gpa) != 0)
return this.gpa.compareTo(other.gpa);
if(this.lastName.compareTo(other.lastName) != 0)
return this.lastName.compareTo(other.lastName);
if(this.firstName.compareTo(other.firstName) != 0)
return this.firstName.compareTo(other.firstName);
return this.id.compareTo(other.id);
}
}
}
public class Main
{
static Student [] list = getList();
static Student [] getList()
{
// unknown implementation . . .
}
public static void main (String []args){
Arrays.sort(list);
for(Student s: list)
System.out.println(s);
}
}
3-
Use the following class to create a class for DateTime representing a certain time in a certain
date. Override the toString method ("mm/dd/yyyy @ hh:mm:ss PM/AM") and implement the
Comparable interface for it.
3.1 Code Provided
package util;
public class Date implements Comparable<Date>{
private int day;
private int month;
private int year;
@Override
public String toString() {
return String.format("%02d/%02d/%04d", month, day, year);
}
public int getDay() {
return day;
}
public int getMonth() {
return month;
}
public int getYear() {
return year;
}
public Date(int day, int month, int year) {
this.day = day;
this.month = month;
this.year = year;
}
@Override
public int compareTo(Date other) {
if(this.year != other.year)
return this.year - other.year;//new Integer(year).compareTo(other.year);
if(this.month != other.month)
return this.month - other.month;
return this.day - other.day;
//return (this.day - other.day) + 31 * (this.month - other.month)
//
+ 372 * (this.year - other.year);
}
}
3.2 Answers in Red
package util;
public class DateTime implements Comparable<DateTime>{
private Date date;
private int hour;
private int minute;
private int second;
private boolean am;
public DateTime(Date date, int hour, int minute, int second, boolean am) {
this.date = date;
this.hour = hour == 12? 0:hour;
this.minute = minute;
this.second = second;
this.am = am;
}
@Override
public String toString() {
return String.format("%s @ %02d:%02d:%02d %s", date, hour == 0?12:hour,
minute, second, am? "AM": "PM");
}
@Override
public int compareTo(DateTime other) {
if(this.date.compareTo(other.date) != 0)
return this.date.compareTo(other.date);
if(this.am != other.am)
return this.am?-1:1;
if(this.hour != other.hour)
return this.hour - other.hour;
if(this.minute != other.minute)
return this.minute - other.minute;
return this.second - other.second;
}
}
4-Choose all the messages printed by the following program
4.1 Code provided:
public class MainClass
{
public static void main(String[] args){
boolean[] flags = new boolean[] {false,false};
try {
if(flags[1] && Integer.parseInt("1.2") >= 1)
flags[1] is false so if is false
System.out.println("Hello");
else if(flags[2])
no value flags[2] so error indexoutofbound
System.out.println(1/0);
}catch(NumberFormatException e) {
System.out.println("first");
}catch(ArithmeticException e) {
System.out.println("second");
}catch(IndexOutOfBoundsException e) {
indexOutOfBound will be thrown first
System.out.println("third");
first output
}catch(Throwable t) {
System.out.println("fourth");
}finally {
System.out.println("fifth");
last output because finally always execute
}
}
}
4.2 Answer:
third
fifth
5-Choose all the messages that the following program writes on "Test.txt".
5.1 Code provided:
import java.io.*;
public class Test{
public static void writeFile(String id) {
PrintWriter pw = null;
try {
pw = new PrintWriter(new FileOutputStream(sFile));
for (int i=0; i<5; i++)
pw.println("id " + id + ":
i=" + i);
pw.close();
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e);
}
}
private static String sFile = "Test.txt";
public static void main(String[] args) {
for(String s: new String[]{"first", "second", "third", "last"})
writeFile(s);
}
}
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
5.2 Answer:
last: i=4
6-Predict the outcome of the following:
6.1 Code provide:
import java.util.*;
public class Student {
public static void main(String[] args) {
Scanner scnr = new Scanner("8, 3.4, 1.3, 12, 4.0, 5, 6, 7, 1, 2");
scnr.useDelimiter("[, .]+");
while(scnr.hasNext()) {
try {
System.out.println(scnr.nextInt()/scnr.nextInt());
}catch(InputMismatchException e) {
scnr.next();
continue;
}catch(Exception e) {
System.out.println("Something went wrong!");
System.exit(1);
}finally {
System.out.println("Finished!");
}
}
}
}
6.2 Answer
2
Finished!
4
Finished!
0
Finished!
Something went wrong!
7-Predict the outcome of the following:
7.1 Code provided
interface Drawable{
void draw(String tool);
}
interface Drivable{
void drive(String road);
}
class Bike implements Drivable, Drawable{
String name;
public Bike(String aName) {
name = aName;
}
public void draw(String tool){
System.out.println("Drawing "+name+" with "+tool);
}
public void drive(String road){
System.out.println("Driving "+name+" on "+road);
}
}
public class MainClass
{
public static void main(String[] args){
Drawable[]items = new Drawable[] {new Bike("Ann's bike"),new Bike("Bob's bike")};
items[0].draw("pen");
((Drivable)items[1]).drive("i75");
}
}
7.2 Answer
Drawing Ann’s bike with pen
Driving Bob’s bile on i75
8-Given the following program and class Date, pick the correct implementation of the
compareTo method from Comparable interface for class Date so that the program can
successfully sort and print the dates stored in array days. Your implementation should work for
any valid list of dates in the array.
8.1-Code Provided
import java.util.*;
class Date implements Comparable{
int month, day, year;
public int compareTo(Object another){
if(another instanceof Date){
/*your code*/
}
return 0;
}
Date(String rep, char delimiter){
month = Integer.parseInt(rep.substring(0, rep.indexOf(delimiter)));
rep = rep.substring(rep.indexOf(delimiter) + 1);
day = Integer.parseInt(rep.substring(0, rep.indexOf(delimiter)));
year = Integer.parseInt(rep.substring(rep.indexOf(delimiter) + 1));
}
public String toString(){
return month + "/" + day + "/" + year;
}
}
public class MyClass {
public static void main(String args[]) {
Date[] days = new Date[] { new Date ( "2/7/2022" , '/' ) , new Date ( "1 5 2022" , ' ' ) , new Date ( "10,5,2020" , ',' ) };
Arrays.sort(days);//sorts days from earliest to oldest!
for (Date d: days)
System.out.println(d);
}
}
8.2 Answer:
1
st
way : Date d = (Date)another; return year != d.year? year - d.year: month != d.month? month -
d.month: day - d.day;
2
nd
way: Date d = (Date)another; return 366*(year-d.y) + 31*(month-d.month)+(day-d.day);
3
rd
way:
Date d = (Date)another; return 372*(year-d.y) + 31*(month-d.month)+(day-d.day);
9-Predict the output:
9.1 Code provided:
Souf(“%+05d”,12)
9.2 Answer
+ means show + if input is positive
0 means show leading zeros
5 means the number of characters or width that will be displayed
d means interger
output is +0012
10-Predict the output:
10.1 Code provided:
Souf(“%-5.1f”,-1.55)
10.2 Answer
- means left align
5 means total number of characters or width
.1 is the total number of decimal places
f means double
output is -1.6(space)
11-Predict the output
11.1 Code provided
Souf(“%10.7s”, “Good morning”);
11.2 Answer
10 means total number of characters
.7 means precision which means only show me the first 7 character of the string when we are
dealing with strings
S means string
Output is (space)(space)(space)Good mo
12-Predict the outcome
12.1 Code provided
Souf(“%012.4e”,2000000000000./3)
12.2 Answer
0 means show leading zeros
12 means total length
.4 precision or decimal places
E means exponential floating point
Outcome is 006.6667e+11
Definitions
Interface
o
Interface types are used to
express common operations
.
o
Interfaces make it possible to make a service available to a wide set.
o
This restaurant is willing to serve anyone who conforms to the Customer
interface with eat and pay methods.
o
The methods of an interface are
automatically public.
o
An interface type is similar to a class.
o
Differences between classes and interfaces:
An interface type
does not have instance variables.
All methods in an interface
type are abstract
(or in Java 8, static or default)
They have a name, parameters, and a return type, but
no
implementation
.
All methods in an interface type are
automatically public
.
An interface type
has no constructor
.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
You
cannot construct
objects of an interface type.
o
A class can implement
more than one interface
o
Example: public class Country implements Measurable, Named(named being
another interface)
o
A class can only extend (
inherit from
) a
single superclass.
o
An interface specifies the behavior that an implementing class should supply (in
Java 8, an interface can now supply a default implementation).
o
A superclass provides some implementation that a subclass inherits.
o
Develop interfaces when you have code that processes objects of different classes
in a common way
o
Children will
automatically inherit
any implementations or extension the parent
class has
Abstract classes
o
An abstract class may contain instance fields, but an interface can't have instance
fields that aren't final and static.
o
An abstract class needs to have at-least one abstract method.
False
o
An abstract class cannot be instantiated, but any non-abstract class extending an
abstract class can be instantiated.
o
A non-abstract class extending an abstract class needs to implement every abstract
method in the abstract class. However, overriding non-abstract methods of the
abstract class is optional.
o
Although an abstract class cannot be instantiated, but it can have constructors.
o
No one is allowed to instantiate from this class, mean that no one can say New
something
o
Is a mixture of instance fields, abstract methods and no abstract methods.
o
The benefits is that you can extend it in other classes.
o
If your class is no abstract you are
not allowed
to store abstract methods in it.
o
An abstract class
may contain instance fields
, but an interface can't have any
instance fields (variables).
o
An abstract class needs to have at-least one abstract method.
False
o
An abstract method cannot be placed in a non-abstract class.
o
Although an abstract class cannot be instantiated, but it can have constructors.
o
A class extending an abstract class needs to implement every abstract method in
the abstract class. However, overriding non-abstract methods of the abstract class
is optional.
o
An abstract class cannot be instantiated, but any class extending an abstract class
can be instantiated.
o
Example:
public abstract class TwoDObject {
protected String name;
public abstract double getArea();
public abstract double getPerimeter();
@Override
public String toString() {
return "Generic 2-D object";
}
public TwoDObject(String name) {
this.name = name;
}
}
public class CircularObject extends TwoDObject{
int radius;
public CircularObject(String name, int radius) {
super(name);
this.radius = radius;
// TODO Auto-generated constructor stub
}
@Override
public double getArea() {
// TODO Auto-generated method stub
return Math.PI*radius*radius;
}
@Override
public double getPerimeter() {
// TODO Auto-generated method stub
return 2*Math.PI*radius;
}
}
File I/O using scanner and printwritter:
o
Reading a Text File using java.util.Scanner
Construct a File object and pass it to Scanner Constructor
Use Scanner.nextLine method to read a single line
Read lines repetitively until Scanner .hasNextLine method returns false.
Close the Scanner at the end.
If there is a problem with input file, it simply passes the exception to
caller:
Example:
public void readFile(String filename) throws IOException‚{
File inFile = new File(filename);
Scanner in = new Scanner(inFile);
for (int i = 1; in.hasNextLine();i++){
System.out.println("line " + i + " : " + in.nextLine());
in.close(;
}
o
Writing into Text File: java.io.PrintWriter
o
Pass filename (and possibly the path) to PrintWriter Constructor.
o
Use print method to write text into the file.
o
Use printIn method to write text into the file and end the text with a new
line.
o
Use printf method to write text with specific format
o
Use flush method to reflect the changes on the file immediately
o
Close the PrintWriter at the end.
o
If there is a problem with output file, it simply passes the exception to
caller:
o
Example:
void writeFile(String filename, String[] data) throw IOException{
PrintWriter out = new PrintWriter(filename);
for (String line: data) out.println(line);
out.flush();
out. close();
Formatting using printf:
◦
public PrintWriter printf(String format, Object... args)
◦
First input parameter specifies format
◦
format contains placeholders like %d: is replaced by an integer
◦
%f: is replaced by a double/float
%c: is replaced by a character
◦
%s: is replaced by a string
◦
%g: is replaced by a floating-point number in scientific notation
◦
Example:
PrintWriter p = new PrintWriter("out.txt");
p.printf("%d of %c %s 6e"
,-123, 2/3.0, 'b', "hello", 0.0000032);
Table 3 Format Types
Code
Type
Example
d
Decimal
integer
123
f
Fixed floating-
point
12.30
e
Exponential
floating-point
1.23e+1
g
General
floating-point
(exponential
notation is
used for very
large or very
small values)
12.3
a
String
Tax:
Formatting Output
◦
You can customize %d and %f and %e placeholder by adding flags to it: %+e, %(d,
%, f etc
◦
◦
Some flags and their meaning
Table 2 Format Flags
Flag
Meaning
Example
-
Left alignment
1.23 followed by spaces
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
0
Show leading zeroes
001.23
+
Show a plus sign for
positive numbers
+1.23
(
Enclose negative
numbers in parentheses
(1.23)
,
Show decimal
separators
12,300
^
Convert letters to
uppercase
1.23E+1
◦
Moreover, width and precision options are available for %d, %s, %d, and %e
%-4d %5.2s %06.3e
Exceptions:
Designing Your Own Exception Types
► You can design your own exception types - subclasses of E xce p ti on or
R untim eE xception.
► Throw an I n s u f f i c i e n tF u n dsE xce p tio n when the amount to withdraw an
amount from a bank account exceeds the current balance.
i f (amount > balance)
{ th r o w new I n s u f f i c i e n t F u n d s E x c e p t i o n ( " w i t h d r a w a l o f "
amount + " exceeds balance o f " + b a l a n c e ) ;
}
► Make I n s u f f i c ie n tF u n d s E x c e p ti on an unchecked exception
► Programmer could have avoided it by calling get Bal anee first
► Extend Runti meExcepti on or one of its subclasses
Designing Your Own Exception Types
► Supply two constructors for the class
► A constructor with no arguments
► A constructor that accepts a message string describing reason for exception
pu blic class In su fficien tF u n d sE xcep tio n extends RuntimeException
{ p u b lic In s u ffic ie n tF u n d s E x c e p tio n O {}
p u b lic In s u ffic ie n tF u n d s E x c e p tio n (S trin g message)
{ super(message);
}
}
► When the exception is caught, its message string can be retrieved
► Using the getMessage method of the Throwable class.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Recommended textbooks for you
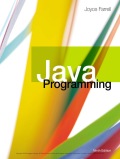
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
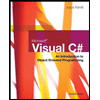
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
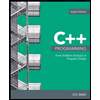
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
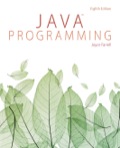
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
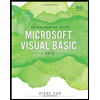
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
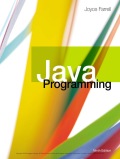
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
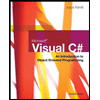
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
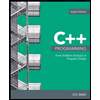
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
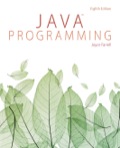
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
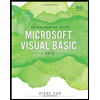
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning