Tutorial-code-with-quality-2023-questions
.docx
keyboard_arrow_up
School
City University of Hong Kong *
*We aren’t endorsed by this school
Course
5351
Subject
Computer Science
Date
Nov 24, 2024
Type
docx
Pages
5
Uploaded by modalinghu
1
CS5351 Software Engineering
2023/2024 Semester A
Tutorial on Code with Quality
Instructions
Q1
is an individual
exercise.
Q2
is a team-based
exercise like what we did in our exercise on Requirement Elicitation. 1.
Form a team of 2 students. Find a partner team for your team: Team A and Team B.
2.
Each team completes Question 2(a)-(c) in 30 minutes:
Plan your design revision and evaluate
what design principles you apply.
3.
A member of your team elaborates on your design plan and explains the design principles to a member of your partner team in 10 minutes
. Your partner team member may raise questions on design rationales for clarification and suggestions.
4.
Your team takes feedback from your partner teams and revises the design in 5 minutes
.
5.
Your team writes a short plan of your design revision and submits it in Canvas (see the assignment section). Ensure to include the names of both students.
Q1. Abstraction, Information Hiding, and Encapsulation
We exercise how to model data structure by inter-relating its operators. This style of abstract data type is known as the functional style (or you can think of the Lisp programming language). Stack Example. The following illustrates an example to represent the behavior of Stack
by their interfaces in the functional style.
new
: create a new Stack.
pop
: return the Stack
identical to the given Stack but with the top element removed.
top
: return the first element of the stack. If the Stack
is empty, it returns an error
.
empty
: return whether the stack is empty (
true
for empty, false
otherwise).
push
: return the Stack
with an additional and given element at the top.
Note that we do not create “local variables.” All variables are the arguments of the corresponding operators.
Style 1
Style 2
pop(new()) = new() pop(push(S,i)) = S top(new()) = error top(push(S,i)) = i empty(new()) = true empty(push(S,i)) = false
pop(new()) = new() pop(push(S,i)) = S top(S) = if empty(S) = true then error
top(push(S,i)) = i empty(new()) = true empty(push(S,i)) = false
Example 1:
push(pop(push(push(new(),4),7)),3)
= push( push(new(),4) ,3)
Example 2:
top(pop(push(push(pop(new()), x), y))))
= top(pop(push(push( new() , x), y))))
= top( push( new() , x) )
= x
How do we implement a stack accordingly?
S = new Stack()
2
pop(.) and push(.) maintain the stack (e.g., S.pop(), S.push(i)
)
top(.) and empty(.) return the stack state info (e.g., S.top(), S.empty()
)
Your Action:
Define a Set
in the functional style by inter-relating the following operators.
empty: create a new empty Set
.
insert: return a Set
with one more element than the given Set
, and the additional element is the given one.
delete: return a Set
without the given element from the given Set
.
member: return whether the Set
contains the element.
Note: A condition “if
a = b
then c
else d
” can be used. Ensure that all variables are used on both sides of the = operator.
Q2. Design Principles
The C++ code mockup on page 3 implements the following sequence diagram for a player to fight
with a single monster in a computer game.
3
#include
<iostream>
using namespace std;
class Player { };
class PlayerController
;
class MonsterController
;
class Monster
;
class Monster {
protected
:
PlayerController
* opponent = NULL;
bool alive = true
; // true - alive, false - killed
int lifespan = 3; // if 0 => alive = false, otherwise true
public
:
void fightWithPlayer(
PlayerController
* o
) { opponent = o
; }
bool isAlive() { return alive; }
void damage(
int point
) {
lifespan = lifespan - point
;
cout << "monster is hurt (" << lifespan << ")" << endl;
if (lifespan <= 0) {
cout << "monster is killed" << endl;
alive = false
;
}
}
void fight();
};
class MonsterController {
Monster
* m;
public
:
MonsterController() { m = new Monster
(); }
Monster
* fightWithMonster(
PlayerController
* pc
) { m-
>fightWithPlayer(
pc
); return m; }
player is hurt (4)
monster is hurt (2)
player is hurt (3)
monster is hurt (1)
player is hurt (2)
monster is hurt (0)
monster is killed
Console window output
https://www
.onlinegdb.c
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Central Pacific University:On a warm, sunny day in late October, Chip Puller parks his car and walks into his office at CentralPacific University. It feels good to be starting as a systems analyst, and he is looking forward tomeeting the other staff. In the office, Anna Liszt introduces herself. “We’ve been assigned to work asa team on a new project. Why don’t I fill you in with the details, and then we can take a tour of thefacilities?”“That sounds good to me,” Chip replies. “How long have you been working here?” “About five years,”answers Anna. “I started as a programmer analyst, but the last few years have been dedicated toanalysis and design. I’m hoping we’ll find some ways to increase our productivity,” Anna continues.“Tell me about the new project,” Chip says. “Well,” Anna replies, “like so many other organizations,we have a large number of microcomputers with different software packages installed on them. Fromwhat I understand, in the 1980s there were few personal computers and…
arrow_forward
Design involves making many decisions among numerous alternatives. Design rationale provides an explicit means of recording those design decisions and the context in which the decisions were made. Explain this through an example.
Book Used: www.hcibook.com/e3/plain/chapsSubject: Human Computer InteractionPs: this is the whole question, it is in regards to design, there are no other references or parts to it.
arrow_forward
Senario
Programming Competition System – Schools and Universities always want to participate in a programming competition. Currently, everything such as competition rules, and the organisation is handled manually. This should be automated so that participants just log onto the system to see if they are eligible to participate or not and be able to monitor their requests online.
Question 1
Create a User Requirement Specification Based on a given Senario
Expected answer with:
1. INTRODUCTION1.1. PURPOSE
1.2. PROJECT OVERVIEW
1.3. PROJECT SCOPE
1.4. PROJECT OBJECTIVES
arrow_forward
Facts:
Software design is the process of defining software methods, functions, objects, and the overall structure and interaction of your code so that the resulting functionality will satisfy your user's
requirements. There are many different ways of designing software, almost all of which involve coming up with an initial design and refining it as necessary. Different developers prefer different
amounts of design upfront or during the implementation phase. Generally, the overall design should be well thought out and reviewed before coding starts. Refer to our section on Design
reviews to learn how to review your design. It is easier to try out different designs upfront and discover problems early in the development cycle than to make a major design change after much
of the code has been written.
Your software design should include a description of the overall architecture. This should include the hardware, databases, and third-party frameworks your software will use or interact with.…
arrow_forward
Q3:Your company has just acquired a smaller company that sells office automation software. The smaller company's spreadsheet software has a large market share, with many satisfied users (the major reason for the acquisition was that these existing users are potential customers for your company's other products). Unfortunately, no documentation for the spreadsheet software can be found, and the source code is not commented. How would you go about maintaining this software to keep the customers happy?
arrow_forward
Computer Science
create DFDS
(i.e. Context, Level-0 and Level-1) /ERDS
and UseCases/Class/Sequence, Activity diagrams.
Design - User interface, input/output screen shots
you have designed for the system
The Grocery store currently uses manual systems
for storing information and billing purposes. This
leads to manual labor and #orts, time
consumptions, manual counts.
An automated system to replace the existing
manual systems is required to store the valuable
data of the store about the customers, suppliers,
stock, and billing details which can be easily
accessed whenever needed through computerized
equipment and software.
The software will help in managing and
maintaining
information about the Product, its types, Suppliers,
Customers, Stock available, etc.
The Grocery Store management system is a
project
that deals with store data computerization and it
includes both purchasing and selling of items,
arrow_forward
Create mulitple IPO charts for a top-down design: grade individual tests for 3 sections of a course, calculate the average in each class, take the average across the 3 sections, calculate a curve, then apply it to each class.
arrow_forward
Plag free answer Don;t copy from chegg
arrow_forward
Scenario: The Best Events Company organizes events, and business is booming, so much so that they need your help to manage their service. They want you to create a software system to manage their employees, clients, suppliers, and events. A part of their software requirement is given below. You are required to do a self-study on how event-organizing companies work and create a design for managing the company's functions. In addition to the requirements below, you are encouraged to add more attributes and functionalities to the system. The company has different types of employees, such as, Sales Managers, Salespersons, Marketing Managers, Marketers, Accountants, Designers and Handymen. Some details required for employees include name, employee ID, department, job title, basic salary, age, date of birth, and passport details are also stored in the system. The table below provides a glimpse of how employees are managed. It is clear from the table that Susan Meyers manages both Shyam…
arrow_forward
115.
Model which is useful when developer is not sure of requirements, or efficiency of an algorithm , business rules, response time, etc is
a.
Waterfall model
b.
Incremental model
c.
Prototyping model
d.
RAD model
arrow_forward
Question#2) Case Study:
JazzN!ghts is a famous Jazz festival, held in Zurich every year. Since its first edition in 1986, it has gone
through several major changes regarding its structure, length and location, but the tickets have always
been sold in a traditional way: through two events agencies. The organizers decided to completely
modernize the tickets selling system and created the following concept. From this year on, the tickets will
be sold in three distinct ways: traditionally, i.e. by the two events agencies, in electronic format directly
on the festival website, and through SBB. All parties will have access to the same unique tickets database
of the new system, to avoid double selling. A partnership with the SBB railway company needs to be set
up, such that SBB can sell combi-tickets including both the festival admission fee and the train ride to the
festival venue at reduced price, from anywhere in Switzerland. This way, more music fans would have
easier and cheaper access…
arrow_forward
Explain the metrics for the design model.
Explain the metrics for testing.
arrow_forward
Task5
arrow_forward
Design Patterns in software development: benefits? Can you provide three design patterns?
arrow_forward
Week 2 - Lecture 3: Introduction to Software Engineering
Class Activity: What is a process model in Software Engineering? Give examples of
different process models followed in Software Engineering and explain any one of them in
detail.
arrow_forward
Software Management
1. Let's assume that you are a project manager and are assigned to develop a visualisation and simulation
system based on the quarry environment in Malaysia. It is an educational learning system that school
children later use to learn and increase awareness of environmental safety and science and technology
matters. This is a group project with four members. As the initial requirement, the team visited a quarry site
in Langkawi to study and understand the activities in the quarry. The proposed system will visualise and
simulate the actions in the quarry (such as blasting, crushing, transportation, and drilling) for learning
purposes. The users (school children) will key in relevant parameters to experience the simulation of quarry
activities. The system development must be completed within twelve months. You may name this proposed
system EELS, which stands for Environment Educational Learning System.
a. Establish the Work Breakdown Structure (WBS) based on the…
arrow_forward
Define the top-down design approach and describe how it works.
arrow_forward
Define the relationship between user-centered design concepts and the software development lifecycle in detail.
A189
arrow_forward
subject: software process and management
arrow_forward
Facts:
Successful projects are managed well. To manage a project efficiently, the manager or development team must choose the software development methodology that will work best for the project at hand. All methodologies have different strengths and weaknesses and exist for different reasons. Here’s an overview of the most commonly used software development methodologies and why different methodologies exist. -Synopsys (Links to an external site.
Question:
Which methodology you're most likely interested to use and why?
arrow_forward
Topic Project: University System
Objective: The aim of this project is to give the students an overview of an Object Oriented Analysis and Design techniques during the System development process.
Outcomes:
1. Understanding the concept of visual modeling using UML.
2. Use a CASE tool to construct appropriate analysis / design diagrams addressing a clearly defined problem
3. Analyze and design a computer-based solution to a clearly defined problem using object oriented techniques.
Project Task:
Creating a System Requirement Specification (SRS) Document
Students are expected to produce an analysis and design document that specifies the architecture of an information system for a fictitious/existent small business/organization. Such a document is known as a SRS Document.
• Project Deliverable 1: Project Report 10 Marks• Project Deliverable 2: Project Presentation 5 Marks
Read the following guidelines, understands and present your response for the tasks assigned in a…
arrow_forward
Project - ITDR2104 (10 Marks)
General Instructions:
This project work consists of four parts. This is not a group work. Every student must
work on this project individually.
This project has to be completed as we make progress in this course.
Each part of the project can be completed within 3 weeks from the completion of relevant
part in class.
Two or more students submitting a similar report will get ZERO marks.
• Submit this report on 23/12/2021 4nm through Blackboard only. No email submission
will be acknowledged/accepted/marked.
Part-1:5 marks
Create a Java Project (with a class and a test class) to represent some real-world entity such as a
technology item (TV, Fan etc), an animal, a person, a vehicle, etc. In our class, we have already
seen example classes such as Circle, Student, Rectangle, TV etc.
The class will:
a) Create a total of 5 member variables for the class by selecting the appropriate data types
for each field. For example, a class to represent a lamp might include…
arrow_forward
LECTURE: Software Design & Architecture
Please draw the use case diagram for the below system. Do it by assuming that you computerized the system.
Girne Tourism Association got funding from ABC and is intended to have a new cafe in Girne to socialize young people in the area. The cafe should enable customers to use tablets for coffee/cake orders. In traditional Cafe’s the waitress can see the status of tables from diagrams in blackboards. Once customers are seated a waiter write down the orders onto a piece of paper and delivers it to the barista in the kitchen for drink preparation. After barista prepares the order, he clicks the bell for the waitress to deliver the food. Once the customer wants to pay the bill, the waitress makes sure to keep each bill organized and “synchronized” with the proper table number. The manager of the cafe used to collect sales information, number of customers information from the waitress and the barista to end up statistical information.
You are…
arrow_forward
When and how should design patterns be used during software development? To what extent can you demonstrate the variety of design patterns?
arrow_forward
In software development, a variety of models are used, with each model having a varying level of significance based on the application. Please sort those models i
arrow_forward
I need the answer as soon as possible
arrow_forward
QUESTION 10
You are a project manager overseeing the program design for a client. The team lead for the project sketches the following on your office whiteboard. What is your best
response?
Note: No assumptions are needed.
O"Fan-in of modules is presented. The program design is approved."
O Fan-through flow of modules is presented. The program design is approved."
O "Control and data couples couples are flowing in wrong direction. The program design is rejected
O"Fan-in of modules is presented. The program design is rejected
O"Fan-out of modules is presented. The program design is approved."
arrow_forward
Explain the concept of design patterns in software development, and provide examples of situations where you might apply the Singleton or Factory pattern.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
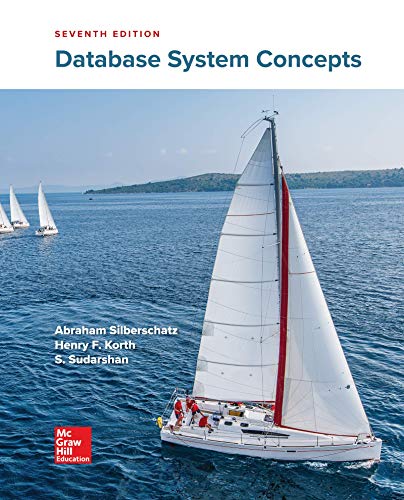
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
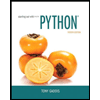
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
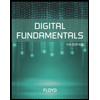
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
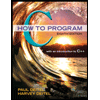
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
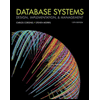
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
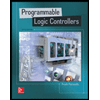
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Central Pacific University:On a warm, sunny day in late October, Chip Puller parks his car and walks into his office at CentralPacific University. It feels good to be starting as a systems analyst, and he is looking forward tomeeting the other staff. In the office, Anna Liszt introduces herself. “We’ve been assigned to work asa team on a new project. Why don’t I fill you in with the details, and then we can take a tour of thefacilities?”“That sounds good to me,” Chip replies. “How long have you been working here?” “About five years,”answers Anna. “I started as a programmer analyst, but the last few years have been dedicated toanalysis and design. I’m hoping we’ll find some ways to increase our productivity,” Anna continues.“Tell me about the new project,” Chip says. “Well,” Anna replies, “like so many other organizations,we have a large number of microcomputers with different software packages installed on them. Fromwhat I understand, in the 1980s there were few personal computers and…arrow_forwardDesign involves making many decisions among numerous alternatives. Design rationale provides an explicit means of recording those design decisions and the context in which the decisions were made. Explain this through an example. Book Used: www.hcibook.com/e3/plain/chapsSubject: Human Computer InteractionPs: this is the whole question, it is in regards to design, there are no other references or parts to it.arrow_forwardSenario Programming Competition System – Schools and Universities always want to participate in a programming competition. Currently, everything such as competition rules, and the organisation is handled manually. This should be automated so that participants just log onto the system to see if they are eligible to participate or not and be able to monitor their requests online. Question 1 Create a User Requirement Specification Based on a given Senario Expected answer with: 1. INTRODUCTION1.1. PURPOSE 1.2. PROJECT OVERVIEW 1.3. PROJECT SCOPE 1.4. PROJECT OBJECTIVESarrow_forward
- Facts: Software design is the process of defining software methods, functions, objects, and the overall structure and interaction of your code so that the resulting functionality will satisfy your user's requirements. There are many different ways of designing software, almost all of which involve coming up with an initial design and refining it as necessary. Different developers prefer different amounts of design upfront or during the implementation phase. Generally, the overall design should be well thought out and reviewed before coding starts. Refer to our section on Design reviews to learn how to review your design. It is easier to try out different designs upfront and discover problems early in the development cycle than to make a major design change after much of the code has been written. Your software design should include a description of the overall architecture. This should include the hardware, databases, and third-party frameworks your software will use or interact with.…arrow_forwardQ3:Your company has just acquired a smaller company that sells office automation software. The smaller company's spreadsheet software has a large market share, with many satisfied users (the major reason for the acquisition was that these existing users are potential customers for your company's other products). Unfortunately, no documentation for the spreadsheet software can be found, and the source code is not commented. How would you go about maintaining this software to keep the customers happy?arrow_forwardComputer Science create DFDS (i.e. Context, Level-0 and Level-1) /ERDS and UseCases/Class/Sequence, Activity diagrams. Design - User interface, input/output screen shots you have designed for the system The Grocery store currently uses manual systems for storing information and billing purposes. This leads to manual labor and #orts, time consumptions, manual counts. An automated system to replace the existing manual systems is required to store the valuable data of the store about the customers, suppliers, stock, and billing details which can be easily accessed whenever needed through computerized equipment and software. The software will help in managing and maintaining information about the Product, its types, Suppliers, Customers, Stock available, etc. The Grocery Store management system is a project that deals with store data computerization and it includes both purchasing and selling of items,arrow_forward
- Create mulitple IPO charts for a top-down design: grade individual tests for 3 sections of a course, calculate the average in each class, take the average across the 3 sections, calculate a curve, then apply it to each class.arrow_forwardPlag free answer Don;t copy from cheggarrow_forwardScenario: The Best Events Company organizes events, and business is booming, so much so that they need your help to manage their service. They want you to create a software system to manage their employees, clients, suppliers, and events. A part of their software requirement is given below. You are required to do a self-study on how event-organizing companies work and create a design for managing the company's functions. In addition to the requirements below, you are encouraged to add more attributes and functionalities to the system. The company has different types of employees, such as, Sales Managers, Salespersons, Marketing Managers, Marketers, Accountants, Designers and Handymen. Some details required for employees include name, employee ID, department, job title, basic salary, age, date of birth, and passport details are also stored in the system. The table below provides a glimpse of how employees are managed. It is clear from the table that Susan Meyers manages both Shyam…arrow_forward
- 115. Model which is useful when developer is not sure of requirements, or efficiency of an algorithm , business rules, response time, etc is a. Waterfall model b. Incremental model c. Prototyping model d. RAD modelarrow_forwardQuestion#2) Case Study: JazzN!ghts is a famous Jazz festival, held in Zurich every year. Since its first edition in 1986, it has gone through several major changes regarding its structure, length and location, but the tickets have always been sold in a traditional way: through two events agencies. The organizers decided to completely modernize the tickets selling system and created the following concept. From this year on, the tickets will be sold in three distinct ways: traditionally, i.e. by the two events agencies, in electronic format directly on the festival website, and through SBB. All parties will have access to the same unique tickets database of the new system, to avoid double selling. A partnership with the SBB railway company needs to be set up, such that SBB can sell combi-tickets including both the festival admission fee and the train ride to the festival venue at reduced price, from anywhere in Switzerland. This way, more music fans would have easier and cheaper access…arrow_forwardExplain the metrics for the design model. Explain the metrics for testing.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
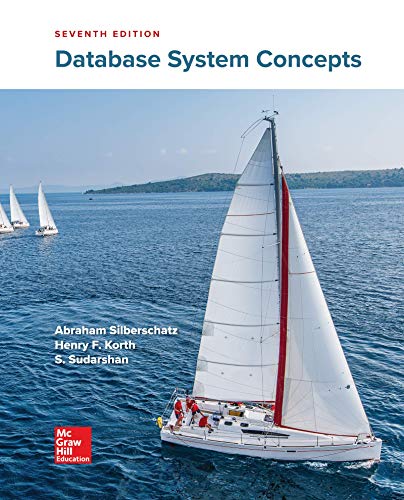
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
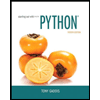
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
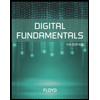
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
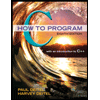
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
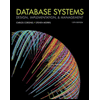
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
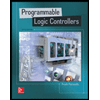
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education