Write in C Language Description A sparse matrix is a matrix in which most of the elements are zero. Representing a sparse matrix by a 2D array leads to wastage of lots of memory as zeroes in the matrix are of no use in most of the cases. So, we can compress the sparse matrix by only store non-zero elements. This means storing non-zero elements with triples- (Row, Column, value). 0 0 0 0 0 0 0 3 0 0 0 0 0 0 0 6 0 0 0 0 9 0 0 0 0 0 0 0 12 0 Here is a 5X6 matrix, there are 4 element non-zero, we using three number represent it 5 6 4 After the three number are (Row, Column, value): row col val 1 1 3 2 3 6 3 2 9 4 4 12 Write a program to read the compressed matrix and print out the original matrix. Input First line consists 3 integers. First and second integers shows the dimensions of original matrix. Third integer k shows number of non-zero elements. Followed k lines are content of the sparse matrix. Each line consists 3 integers, row number, column number, and data. Output Output original matrix. Attach a white space behind each element. Sample Input 1 5 6 4 1 1 3 2 3 6 3 2 9 4 4 12 Sample Output 1 0 0 0 0 0 0 0 3 0 0 0 0 0 0 0 6 0 0 0 0 9 0 0 0 0 0 0 0 12 0 Expert Answer (doesn't match the sample input. it must match the sample input and output) Step 1 The program is written in c Step 2 #include int main() { int a[20][20],n,i,j,k,l; for(i=0;i<20;i++) { for(j=0;j<20;j++) { a[i][j]=0; } } printf("Enter the number of elements\n"); scanf("%d",&n); printf("Enter the elements\n"); for(k=0;k
Write in C Language
Description
A sparse matrix is a matrix in which most of the elements are zero. Representing a sparse matrix by a 2D array leads to wastage of lots of memory as zeroes in the matrix are of no use in most of the cases. So, we can compress the sparse matrix by only store non-zero elements. This means storing non-zero elements with triples- (Row, Column, value).
0 0 0 0 0 0
0 3 0 0 0 0
0 0 0 6 0 0
0 0 9 0 0 0
0 0 0 0 12 0
Here is a 5X6 matrix, there are 4 element non-zero, we using three number represent it
5 6 4
After the three number are (Row, Column, value):
row col val
1 1 3
2 3 6
3 2 9
4 4 12
Write a
Input
First line consists 3 integers. First and second integers shows the dimensions of original matrix. Third integer k shows number of non-zero elements. Followed k lines are content of the sparse matrix. Each line consists 3 integers, row number, column number, and data.
Output
Output original matrix. Attach a white space behind each element.
Sample Input 1
5 6 4Sample Output 1
0 0 0 0 0 0Expert Answer (doesn't match the sample input. it must match the sample input and output)
The program is written in c
#include <stdio.h>
int main()
{
int a[20][20],n,i,j,k,l;
for(i=0;i<20;i++)
{
for(j=0;j<20;j++)
{
a[i][j]=0;
}
}
printf("Enter the number of elements\n");
scanf("%d",&n);
printf("Enter the elements\n");
for(k=0;k<n;k++)
{
scanf("%d",&i);
scanf("%d",&j);
scanf("%d",&a[i][j]);
}
for(k=0;k<=i;k++)
{
for(l=0;l<=j;l++)
{
printf("%d ",a[k][l]);
}
printf("\n");
}
return 0;
}

Step by step
Solved in 3 steps with 1 images

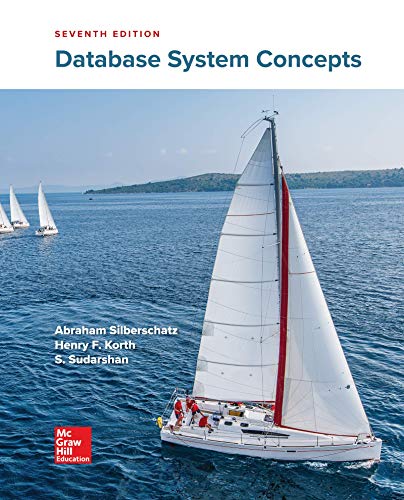
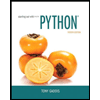
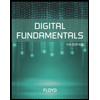
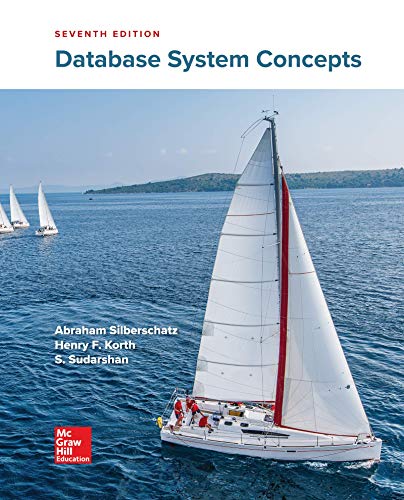
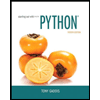
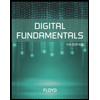
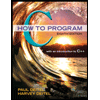
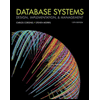
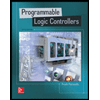