Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator Here is a working code: please modify this code so it works in Hypergrade when I submit it so it passes all the test casses in Hypergrade.Because right now when I upload it to Hypergrade it says 0 out of 5 casses pased. It has to pass all the test casses in Hypergrade Please fix this code. I do not need thanks for playing or goodbye in the program. import java.util.Scanner; public class RetailPriceCalculator { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); while (true) { double wholesaleCost = getWholesaleCost(keyboard); if (wholesaleCost == -1) { break; } double markupPercentage = getMarkupPercentage(keyboard); if (markupPercentage == -1) { break; } double retailPrice = calculateRetail(wholesaleCost, markupPercentage); System.out.printf("The retail price is: %.2f%n", retailPrice); } keyboard.close(); } public static double getWholesaleCost(Scanner keyboard) { System.out.println("Please enter the wholesale cost or -1 to exit:"); double cost = keyboard.nextDouble(); if (cost == -1) { return -1; } else if (cost < 0) { System.out.println("Wholesale cost cannot be a negative value."); return getWholesaleCost(keyboard); } return cost; } public static double getMarkupPercentage(Scanner keyboard) { System.out.println("Please enter the markup percentage or -1 to exit:"); double percentage = keyboard.nextDouble(); if (percentage == -1) { return -1; } else if (percentage < -100) { System.out.println("Markup cannot be less than -100%."); return getMarkupPercentage(keyboard); } return percentage; } public static double calculateRetail(double wholesaleCost, double markupPercentage) { return wholesaleCost * (1 + markupPercentage / 100); } } Test Case 4 Please enter the wholesale cost or -1 exit:\n -200ENTER Wholesale cost cannot be a negative value.\n Please enter the wholesale cost again or -1 exit:\n 10ENTER Please enter the markup percentage or -1 exit:\n -200ENTER Markup cannot be less than -100%.\n Please enter the markup again or -1 exit:\n 50ENTER The retail price is: 15.00\n Please enter the wholesale cost or -1 exit:\n -1ENTER Test Case 5 Please enter the wholesale cost or -1 exit:\n -1ENTER
import java.util.Scanner;
public class RetailPriceCalculator {
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
while (true) {
double wholesaleCost = getWholesaleCost(keyboard);
if (wholesaleCost == -1) {
break;
}
double markupPercentage = getMarkupPercentage(keyboard);
if (markupPercentage == -1) {
break;
}
double retailPrice = calculateRetail(wholesaleCost, markupPercentage);
System.out.printf("The retail price is: %.2f%n", retailPrice);
}
keyboard.close();
}
public static double getWholesaleCost(Scanner keyboard) {
System.out.println("Please enter the wholesale cost or -1 to exit:");
double cost = keyboard.nextDouble();
if (cost == -1) {
return -1;
} else if (cost < 0) {
System.out.println("Wholesale cost cannot be a negative value.");
return getWholesaleCost(keyboard);
}
return cost;
}
public static double getMarkupPercentage(Scanner keyboard) {
System.out.println("Please enter the markup percentage or -1 to exit:");
double percentage = keyboard.nextDouble();
if (percentage == -1) {
return -1;
} else if (percentage < -100) {
System.out.println("Markup cannot be less than -100%.");
return getMarkupPercentage(keyboard);
}
return percentage;
}
public static double calculateRetail(double wholesaleCost, double markupPercentage) {
return wholesaleCost * (1 + markupPercentage / 100);
}
}
Test Case 4
-200ENTER
Wholesale cost cannot be a negative value.\n
Please enter the wholesale cost again or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-200ENTER
Markup cannot be less than -100%.\n
Please enter the markup again or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 5
-1ENTER



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

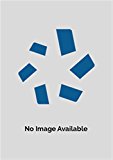
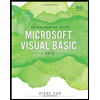
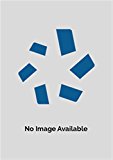
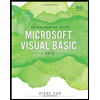
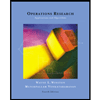
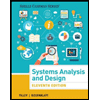