Send these three components to a function called printout (table, dish, chef) You need to create the code for this function. The function definition and the parameter is given to you in the starter code. Do not change the name of this function. The output of this function is a string with the order details that is nicely formatted so the chef can easily read it. First, add "T", the table number, and a space to the string. Next, process the dish name. Add the dish name to the string. a. If the length of the dish name is 10 characters or fewer, add the text "easy" to the string to let the chef know it's an easy dish to cook. b. If the length of the dish name is more than 10 characters, add the text "RUSH" to the string to let the chef know they're going to have to cook quickly to get the dish out on time. Finally, look at the last letter in the order. If it is 'c', add 'cold' to the string. If it is 'g', add 'grill' to the string. If it is 's', add 'saute' to the string. If it is 'p', add 'pastry' to the string. Include spaces in the string like in normal writing. Using the example from above, the order "3&creme brulee$p" would result in a string: T3 creme brulee RUSH pastry Return this string back to kitchen (). Back inside kitchen () You need to continue creating the code for this function. Store the string returned from printout (). Print it for the user. kitchen () does not need to return anything. Back inside the main part of your program This kicks the program back down to the main part (outside of the functions) where your code should automatically pull the next order from the list and send it to kitchen (). You need to create this function. Once you've processed all the orders, your program can end. Clarifications • The table number can be more than one digit. Therefore, don't try to get the table number by indexing the first character of the order. ● The chef type (c, g, s, or p) will always be one lowercase letter; however, don't try to index to get that either. You want to allow for flexibility in case Chef Angela adds more chef types in the future that may have two or more characters. The dish name can contain spaces. You don't have to worry about capitalization. The rest of the order will be typed in without spaces. Reminder: the user can type in as many orders as they want until they enter 'x'. ● ●
Send these three components to a function called printout (table, dish, chef) You need to create the code for this function. The function definition and the parameter is given to you in the starter code. Do not change the name of this function. The output of this function is a string with the order details that is nicely formatted so the chef can easily read it. First, add "T", the table number, and a space to the string. Next, process the dish name. Add the dish name to the string. a. If the length of the dish name is 10 characters or fewer, add the text "easy" to the string to let the chef know it's an easy dish to cook. b. If the length of the dish name is more than 10 characters, add the text "RUSH" to the string to let the chef know they're going to have to cook quickly to get the dish out on time. Finally, look at the last letter in the order. If it is 'c', add 'cold' to the string. If it is 'g', add 'grill' to the string. If it is 's', add 'saute' to the string. If it is 'p', add 'pastry' to the string. Include spaces in the string like in normal writing. Using the example from above, the order "3&creme brulee$p" would result in a string: T3 creme brulee RUSH pastry Return this string back to kitchen (). Back inside kitchen () You need to continue creating the code for this function. Store the string returned from printout (). Print it for the user. kitchen () does not need to return anything. Back inside the main part of your program This kicks the program back down to the main part (outside of the functions) where your code should automatically pull the next order from the list and send it to kitchen (). You need to create this function. Once you've processed all the orders, your program can end. Clarifications • The table number can be more than one digit. Therefore, don't try to get the table number by indexing the first character of the order. ● The chef type (c, g, s, or p) will always be one lowercase letter; however, don't try to index to get that either. You want to allow for flexibility in case Chef Angela adds more chef types in the future that may have two or more characters. The dish name can contain spaces. You don't have to worry about capitalization. The rest of the order will be typed in without spaces. Reminder: the user can type in as many orders as they want until they enter 'x'. ● ●
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Sample Output:
HELLO AMAZING ANGELA'S KITCHEN WAIT STAFF
Enter orders one at a time or x to quit
Order: 2&steak$g
Order: 1&salad$c
Order: 456&churros$p
Order: 3&xiao long bao$s
Order: x
Here are the orders for each chef:
T2 steak easy grill
T1 salad easy cold
T456 churros RUSH pastry
T3 xiao long bao RUSH saute

Transcribed Image Text:Send these three components to a function called printout (table,
dish, chef)
You need to create the code for this function. The function definition and the parameter is given to you in
the starter code. Do not change the name of this function.
The output of this function is a string with the order details that is nicely formatted so the chef can easily
read it.
First, add "T", the table number, and a space to the string.
Next, process the dish name. Add the dish name to the string.
a. If the length of the dish name is 10 characters or fewer, add the text "easy" to the string to let the
chef know it's an easy dish to cook.
b.
If the length of the dish name is more than 10 characters, add the text "RUSH" to the string to let
the chef know they're going to have to cook quickly to get the dish out on time.
Finally, look at the last letter in the order. If it is 'c', add 'cold' to the string. If it is 'g', add 'grill' to the string.
If it is 's', add 'saute' to the string. If it is 'p', add 'pastry' to the string.
Include spaces in the string like in normal writing.
Using the example from above, the order "3&creme brulee$p" would result in a string:
T3 creme brulee RUSH pastry
Return this string back to kitchen ().
Back inside kitchen ()
You need to continue creating the code for this function.
Store the string returned from printout (). Print it for the user.
kitchen () does not need to return anything.
Back inside the main part of your program
This kicks the program back down to the main part (outside of the functions) where your code should
automatically pull the next order from the list and send it to kitchen (). You need to create this function.
Once you've processed all the orders, your program can end.
Clarifications
The table number can be more than one digit. Therefore, don't try to get the table number by
indexing the first character of the order.
● The chef type (c, g, s, or p) will always be one lowercase letter; however, don't try to index to get
that either. You want to allow for flexibility in case Chef Angela adds more chef types in the future
that may have two or more characters.
The dish name can contain spaces. You don't have to worry about capitalization. The rest of the
order will be typed in without spaces.
Reminder: the user can type in as many orders as they want until they enter 'x'.
![Case scenario
You have been hired at Angela's Kitchen, a restaurant in Los Angeles. Chef and owner Angela has been
asking her wait staff to take orders on paper. She would like a new system where the wait staff can type in
orders. The system should also print tickets for the kitchen staff that are easy to read so they know what
dish to make and what table it belongs to.
What your Python program should do
Your starter code should be (do not change this)
def printout(table, dish, chef):
# Finish the rest of this function
def kitchen(order):
# Finish the rest of this function
# Start your main block of code here
In the main part of your code outside of the functions
Ask the user to type in an order in this format (without the square brackets):
[table number] & [the name of the dish] $ [c, g, s, or pl
The number is the table number. This is followed by an ampersand '&'.
Next is the name of the dish. This is followed by a dollar sign '$'.
At the end is c, g, s, or p, in lowercase. This denotes which chef will receive the order. 'c' means that the
order will go to the cold bar (salads, appetizers). 'g' means that it will go to the grill. 's' means that it will go
to the saute station. 'p' means that it will go to the pastry chef.
For example:
3&creme brulee$p
This means table number 3, the dish is called 'creme brulee', and it is going to the pastry chef.
The user will type all of this on one line with no spaces except for in the dish name.
Store this order into a list.
Let the user keep typing in orders. Store all orders in the same list. The user stops entering orders by
typing in 'x' without quotes.
Once you have all of the orders, access each order in the list and send it to a function called kitchen ()
that takes in the order as a parameter.
Send each order to a function called kitchen (order)
You need to create the code for this function. The function definition and the parameter is given to you in
the starter code. Do not change the name of this function.
The function separates out the various components of the order: table number, dish name, and which chef
it is going to.
You need to send these three components to another function that will return a string.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1adae9eb-3ce4-42d1-a66e-dfadef5e5ab4%2Fcd6d577b-695f-4c65-9451-77dfd3de539b%2Fl4ooa3_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Case scenario
You have been hired at Angela's Kitchen, a restaurant in Los Angeles. Chef and owner Angela has been
asking her wait staff to take orders on paper. She would like a new system where the wait staff can type in
orders. The system should also print tickets for the kitchen staff that are easy to read so they know what
dish to make and what table it belongs to.
What your Python program should do
Your starter code should be (do not change this)
def printout(table, dish, chef):
# Finish the rest of this function
def kitchen(order):
# Finish the rest of this function
# Start your main block of code here
In the main part of your code outside of the functions
Ask the user to type in an order in this format (without the square brackets):
[table number] & [the name of the dish] $ [c, g, s, or pl
The number is the table number. This is followed by an ampersand '&'.
Next is the name of the dish. This is followed by a dollar sign '$'.
At the end is c, g, s, or p, in lowercase. This denotes which chef will receive the order. 'c' means that the
order will go to the cold bar (salads, appetizers). 'g' means that it will go to the grill. 's' means that it will go
to the saute station. 'p' means that it will go to the pastry chef.
For example:
3&creme brulee$p
This means table number 3, the dish is called 'creme brulee', and it is going to the pastry chef.
The user will type all of this on one line with no spaces except for in the dish name.
Store this order into a list.
Let the user keep typing in orders. Store all orders in the same list. The user stops entering orders by
typing in 'x' without quotes.
Once you have all of the orders, access each order in the list and send it to a function called kitchen ()
that takes in the order as a parameter.
Send each order to a function called kitchen (order)
You need to create the code for this function. The function definition and the parameter is given to you in
the starter code. Do not change the name of this function.
The function separates out the various components of the order: table number, dish name, and which chef
it is going to.
You need to send these three components to another function that will return a string.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
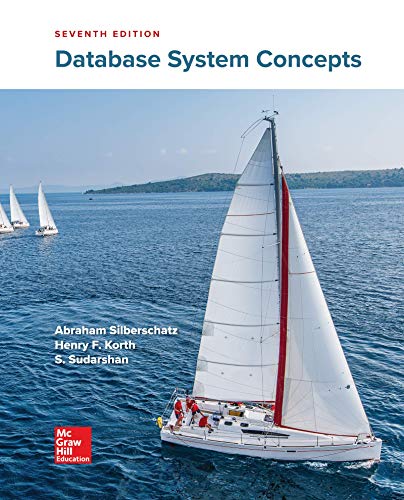
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
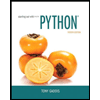
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
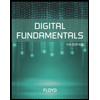
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
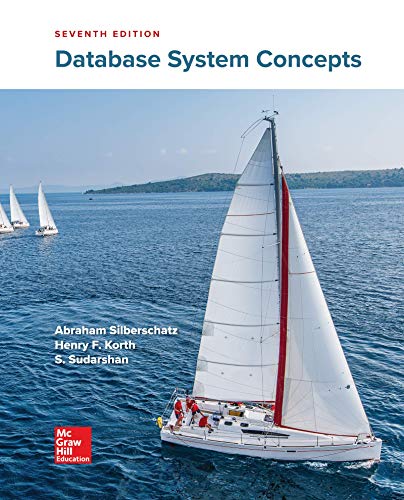
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
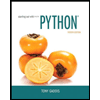
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
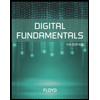
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
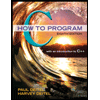
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
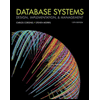
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
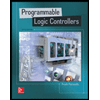
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education