Review the UML diagram provided for a game software application. A text version is available: Text Version for UML diagram Word Document. You may notice that it is incomplete - it is missing attributes and methods, and only includes a small portion of a complete game application. This is quite common! As a software developer, typically you will be given pieces of the puzzle or tasks to complete as part of a larger project and as a member of a larger team. It takes practice to focus on the information you are given to determine what you have and what steps you need to take to complete the task. Specifically, the game application requires that only one instance of the game be able to exist in memory at any given time. This can be accomplished by creating unique identifiers for each instance of the game. Complete the UML diagram to accurately represent a singleton pattern for the Game Service class. You should replace the question marks in the UML diagram with appropriate static and instance attributes and/or methods to make the ordinary class into a singleton. You may create the diagram using Lucidchart or a tool of your choice, such as draw.io or Visio. A tutorial for creating UML diagrams is available: Lucidchart Tutorial for Creating Class Diagrams PDF. Code below just need help completing the UML chart: package com.gamingroom; import java.util.ArrayList; import java.util.List; /** * A singleton service for the game engine * * @author coce@snhu.edu */ public class GameService { /** * A list of the active games */ private static List games = new ArrayList(); /* * Holds the next game identifier */ private static long nextGameId = 1; // FIXME: Add missing pieces to turn this class a singleton private static GameService instance = new GameService(); private void GameService() { } public static GameService getInstance() { return instance; } /** * Construct a new game instance * * @param name the unique name of the game * @return the game instance (new or existing) */ public Game addGame(String name) { // a local game instance Game game = null; for (Game currentGame: games) { if (currentGame.getName().equals(name)) { return currentGame; } } // if not found, make a new game instance and add to list of games if (game == null) { game = new Game(nextGameId++, name); games.add(game); } // return the new/existing game instance to the caller return game; } /** * Returns the game instance at the specified index. * * Scope is package/local for testing purposes. * * @param index index position in the list to return * @return requested game instance */ Game getGame(int index) { return games.get(index); } //Returns game instance with specific id public Game getGame(long id) { // a local game instance Game game = null; // if found, simply assign that instance to the local variable // If a game with name exists it returns the existing instance without adding to data structure for (Game currentGame: games) { if (currentGame.getId() == id) { return currentGame; } } return game; } public Game getGame(String name) { // a local game instance Game game = null; //if a game already exists with this name, return game instance for (Game currentGame: games) { if (currentGame.getName().equals(name)) { game = currentGame; } } return game; } /** * Returns the number of games currently active * * @return the number of games currently active */ public int getGameCount() { return games.size(); } }
Review the UML diagram provided for a game software application. A text version is available: Text Version for UML diagram Word Document. You may notice that it is incomplete - it is missing attributes and methods, and only includes a small portion of a complete game application. This is quite common! As a software developer, typically you will be given pieces of the puzzle or tasks to complete as part of a larger project and as a member of a larger team. It takes practice to focus on the information you are given to determine what you have and what steps you need to take to complete the task.
Specifically, the game application requires that only one instance of the game be able to exist in memory at any given time. This can be accomplished by creating unique identifiers for each instance of the game.
- Complete the UML diagram to accurately represent a singleton pattern for the Game Service class. You should replace the question marks in the UML diagram with appropriate static and instance attributes and/or methods to make the ordinary class into a singleton. You may create the diagram using Lucidchart or a tool of your choice, such as draw.io or Visio. A tutorial for creating UML diagrams is available: Lucidchart Tutorial for Creating Class Diagrams PDF.
- Code below just need help completing the UML chart:
package com.gamingroom;
import java.util.ArrayList;
import java.util.List;
/**
* A singleton service for the game engine
*
* @author coce@snhu.edu
*/
public class GameService {
/**
* A list of the active games
*/
private static List<Game> games = new ArrayList<Game>();
/*
* Holds the next game identifier
*/
private static long nextGameId = 1;
// FIXME: Add missing pieces to turn this class a singleton
private static GameService instance = new GameService();
private void GameService() {
}
public static GameService getInstance() {
return instance;
}
/**
* Construct a new game instance
*
* @param name the unique name of the game
* @return the game instance (new or existing)
*/
public Game addGame(String name) {
// a local game instance
Game game = null;
for (Game currentGame: games) {
if (currentGame.getName().equals(name)) {
return currentGame;
}
}
// if not found, make a new game instance and add to list of games
if (game == null) {
game = new Game(nextGameId++, name);
games.add(game);
}
// return the new/existing game instance to the caller
return game;
}
/**
* Returns the game instance at the specified index.
* <p>
* Scope is package/local for testing purposes.
* </p>
* @param index index position in the list to return
* @return requested game instance
*/
Game getGame(int index) {
return games.get(index);
}
//Returns game instance with specific id
public Game getGame(long id) {
// a local game instance
Game game = null;
// if found, simply assign that instance to the local variable
// If a game with name exists it returns the existing instance without adding to data structure
for (Game currentGame: games) {
if (currentGame.getId() == id) {
return currentGame;
}
}
return game;
}
public Game getGame(String name) {
// a local game instance
Game game = null;
//if a game already exists with this name, return game instance
for (Game currentGame: games) {
if (currentGame.getName().equals(name)) {
game = currentGame;
}
}
return game;
}
/**
* Returns the number of games currently active
*
* @return the number of games currently active
*/
public int getGameCount() {
return games.size();
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

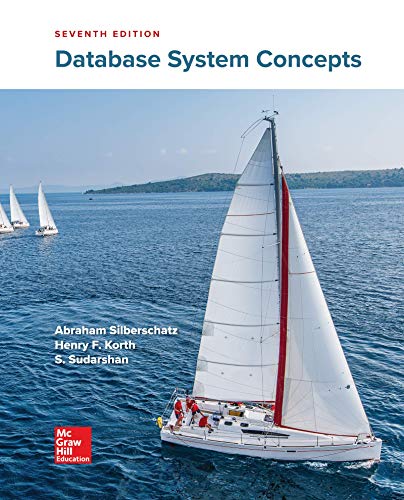
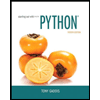
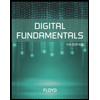
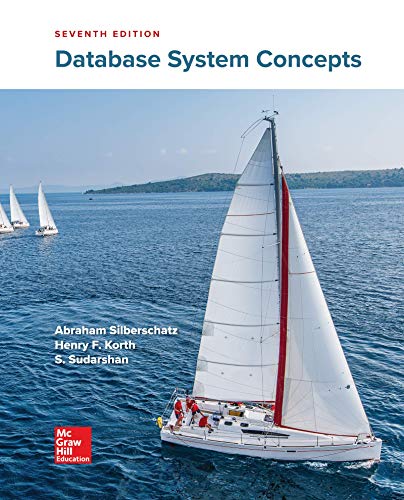
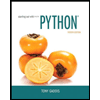
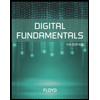
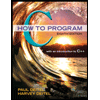
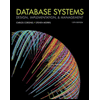
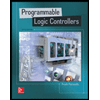