(Querying an Array of Invoice Objects) Use the class Invoice provided in the lab assignment to create an array of Invoice objects. Class Invoice includes four properties—a PartNumber (type int), a PartDescription (type string), a Quantity of the item being purchased (type int) and a Price (type decimal).Write a console application that performs the following queries on the array of Invoice objects and displays the results: a) Use LINQ to sort the Invoice objects by PartDescription. b) Use LINQ to sort the Invoice objects by Price. c) Use LINQ to select the PartDescription and Quantity and sort the results by Quantity. d) Use LINQ to select from each Invoice the PartDescription and the value of the Invoice (i.e., Quantity * Price). Name the calculated column InvoiceTotal. Order the results by Invoice value. [Hint: Use let to store the result of Quantity * Price in a new range variable total.] e) Using the results of the LINQ query in Part d, select the InvoiceTotals in the range $200 to $500. Sample Data 83 Electric sander 7 57.98 24 Power saw 18 99.99 7 Sledge hammer 11 21.50 77 Hammer 76 11.99 39 Lawn mower 3 79.50 68 Screwdriver 106 6.99 56 Jig saw 21 11.00 3 Wrench 34 7.50 Class Invoice public class Invoice { // declare variables for Invoice object private int quantityValue; private decimal priceValue; // auto-implemented property PartNumber public int PartNumber { get; set; } // auto-implemented property PartDescription public string PartDescription { get; set; } // four-argument constructor public Invoice( int part, string description, int count, decimal pricePerItem ) { PartNumber = part; PartDescription = description; Quantity = count; Price = pricePerItem; } // end constructor // property for quantityValue; ensures value is positive public int Quantity { get { return quantityValue; } // end get set { if ( value > 0 ) // determine whether quantity is positive quantityValue = value; // valid quantity assigned } // end set } // end property Quantity // property for pricePerItemValue; ensures value is positive public decimal Price { get { return priceValue; } // end get set { if ( value >= 0M ) // determine whether price is non-negative priceValue = value; // valid price assigned } // end set } // end property Price // return string containing the fields in the Invoice in a nice format public override string ToString() { // left justify each field, and give large enough spaces so // all the columns line up return string.Format( "{0,-5} {1,-20} {2,-5} {3,6:C}", PartNumber, PartDescription, Quantity, Price ); } // end method ToString } // end class Invoice
(Querying an Array of Invoice Objects) Use the class Invoice provided in the lab assignment to create an array of Invoice objects.
Class Invoice includes four properties—a PartNumber (type int), a PartDescription (type string), a Quantity of the item being purchased (type int) and a Price (type decimal).Write a console application that performs the following queries on the array of Invoice objects and displays the results:
a) Use LINQ to sort the Invoice objects by PartDescription.
b) Use LINQ to sort the Invoice objects by Price.
c) Use LINQ to select the PartDescription and Quantity and sort the results by Quantity.
d) Use LINQ to select from each Invoice the PartDescription and the value of the Invoice
(i.e., Quantity * Price). Name the calculated column InvoiceTotal. Order the results by Invoice value. [Hint: Use let to store the result of Quantity * Price in a new range variable total.]
e) Using the results of the LINQ query in Part d, select the InvoiceTotals in the range
$200 to $500.
Sample Data
83 Electric sander 7 57.98
24 Power saw 18 99.99
7 Sledge hammer 11 21.50
77 Hammer 76 11.99
39 Lawn mower 3 79.50
68 Screwdriver 106 6.99
56 Jig saw 21 11.00
3 Wrench 34 7.50
Class Invoice
public class Invoice
{
// declare variables for Invoice object
private int quantityValue;
private decimal priceValue;
// auto-implemented property PartNumber
public int PartNumber { get; set; }
// auto-implemented property PartDescription
public string PartDescription { get; set; }
// four-argument constructor
public Invoice( int part, string description,
int count, decimal pricePerItem )
{
PartNumber = part;
PartDescription = description;
Quantity = count;
Price = pricePerItem;
} // end constructor
// property for quantityValue; ensures value is positive
public int Quantity
{
get
{
return quantityValue;
} // end get
set
{
if ( value > 0 ) // determine whether quantity is positive
quantityValue = value; // valid quantity assigned
} // end set
} // end property Quantity
// property for pricePerItemValue; ensures value is positive
public decimal Price
{
get
{
return priceValue;
} // end get
set
{
if ( value >= 0M ) // determine whether price is non-negative
priceValue = value; // valid price assigned
} // end set
} // end property Price
// return string containing the fields in the Invoice in a nice format
public override string ToString()
{
// left justify each field, and give large enough spaces so
// all the columns line up
return string.Format( "{0,-5} {1,-20} {2,-5} {3,6:C}",
PartNumber, PartDescription, Quantity, Price );
} // end method ToString
} // end class Invoice

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

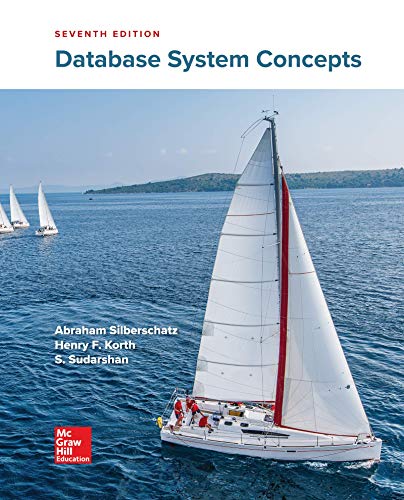
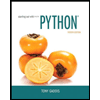
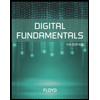
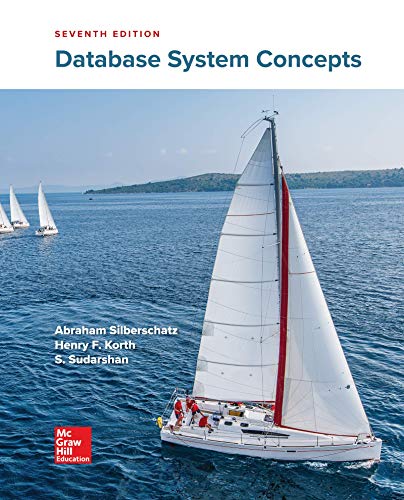
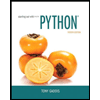
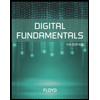
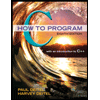
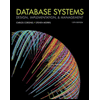
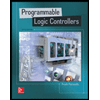