Microsoft Visual C# 7th edition. need help, please. Thanks In previous chapters, you created applications for Marshall’s Murals. Now, modify the version of the MarshallsRevenue program created in Chapter 5 so that after mural data entry is complete, the user is prompted for the appropriate number of customer names for both the interior and exterior murals and a code for each that indicates the mural style: L for landscape S for seascape A for abstract C for children’s O for other When a code is invalid, re-prompt the user for a valid code continuously. For example, if Y is input, output Y is not a valid code, and re-prompt the user until a valid code is entered. After data entry is complete, display a count of each type of mural. For example the output should be in the following format with the correct number next to each mural type: The interior murals scheduled are: Landscape 1 Seascape 2 Abstract 1 Children's 3 Other 9 The exterior murals scheduled are: Landscape 4 Seascape 0 Abstract 2 Children's 4 Other 0 Then, continuously prompt the user for a mural style code until the user enters a sentinel value (the uppercase character Z should be used as the sentinel value). With each code entry, display a list of all the customers with that code and whether their mural is interior or exterior. If the requested code is invalid, display an appropriate message and re-prompt the user. For example if L is input, the output might be: Customers ordering Landscape murals are: Katie Interior Jake Exterior If U is entered, the output should be U is not a valid code. In order to prepend the $ to currency values, the program will need to use the CultureInfo.GetCultureInfo method. In order to do this, include the statement using System.Globalization; at the top of your program and format the output statements as follows: WriteLine("This is an example: {0}", value.ToString("C", CultureInfo.GetCultureInfo("en-US"))); I need help finding customer names for each mural style, please. /*chapter 6*/ int x = 0; bool flag = false; string[,] interiorMuralCustomers = new string[2, numInterior]; string[,] exteriorMuralCustomers = new string[2, numExterior]; int LcountInterior = 0; int ScountInterior = 0; int AcountInterior = 0; int CcountInterior = 0; int OcountInterior = 0; for (x = 0; x < numInterior; ++x) { WriteLine("Enter name of customer {0} for interior mural:", (x + 1)); string name = ReadLine(); interiorMuralCustomers[0, x] = name; do { WriteLine("Enter Mural code. Valid codes are L, S, A, C, and O,"); string code = ReadLine(); if (code == "L" || code == "S" || code == "A" || code == "C" || code == "O") { interiorMuralCustomers[1, x] = code; flag = false; } else {WriteLine("{0} is not a valid code", code); WriteLine("Invalid code. Valid codes are L, S, A, C, and O,"); flag = true; } } while (flag); } for(x=0;x
Microsoft Visual C# 7th edition. need help, please. Thanks
In previous chapters, you created applications for Marshall’s Murals.
Now, modify the version of the MarshallsRevenue program created in Chapter 5 so that after mural data entry is complete, the user is prompted for the appropriate number of customer names for both the interior and exterior murals and a code for each that indicates the mural style:
- L for landscape
- S for seascape
- A for abstract
- C for children’s
- O for other
When a code is invalid, re-prompt the user for a valid code continuously. For example, if Y is input, output Y is not a valid code, and re-prompt the user until a valid code is entered.
After data entry is complete, display a count of each type of mural. For example the output should be in the following format with the correct number next to each mural type:
The interior murals scheduled are: Landscape 1 Seascape 2 Abstract 1 Children's 3 Other 9 The exterior murals scheduled are: Landscape 4 Seascape 0 Abstract 2 Children's 4 Other 0
Then, continuously prompt the user for a mural style code until the user enters a sentinel value (the uppercase character Z should be used as the sentinel value).
With each code entry, display a list of all the customers with that code and whether their mural is interior or exterior. If the requested code is invalid, display an appropriate message and re-prompt the user. For example if L is input, the output might be:
Customers ordering Landscape murals are: Katie Interior Jake Exterior
If U is entered, the output should be U is not a valid code.
In order to prepend the $ to currency values, the program will need to use the CultureInfo.GetCultureInfo method. In order to do this, include the statement using System.Globalization; at the top of your program and format the output statements as follows: WriteLine("This is an example: {0}", value.ToString("C", CultureInfo.GetCultureInfo("en-US")));
I need help finding customer names for each mural style, please.
/*chapter 6*/
int x = 0;
bool flag = false;
string[,] interiorMuralCustomers = new string[2, numInterior];
string[,] exteriorMuralCustomers = new string[2, numExterior];
int LcountInterior = 0;
int ScountInterior = 0;
int AcountInterior = 0;
int CcountInterior = 0;
int OcountInterior = 0;
for (x = 0; x < numInterior; ++x)
{
WriteLine("Enter name of customer {0} for interior mural:", (x + 1));
string name = ReadLine();
interiorMuralCustomers[0, x] = name;
do
{
WriteLine("Enter Mural code. Valid codes are L, S, A, C, and O,");
string code = ReadLine();
if (code == "L" || code == "S" || code == "A" || code == "C" || code == "O")
{
interiorMuralCustomers[1, x] = code;
flag = false;
}
else
{WriteLine("{0} is not a valid code", code);
WriteLine("Invalid code. Valid codes are L, S, A, C, and O,");
flag = true;
}
}
while (flag);
}
for(x=0;x<numInterior;++x)
{
switch(interiorMuralCustomers[1, x])
{
case "L":
LcountInterior++;
break;
case "S":
ScountInterior++;
break;
case "A":
AcountInterior++;
break;
case "C":
CcountInterior++;
break;
case "O":
OcountInterior++;
break;
}
WriteLine("The interior murals scheduled are:");
WriteLine("Landscape {0}", LcountInterior);
WriteLine("Seascape {0}", ScountInterior);
WriteLine("Abstract {0}", AcountInterior);
WriteLine("Children's {0}", CcountInterior);
WriteLine("Other {0}\n\n", OcountInterior);
}
for (x = 0; x < numExterior; ++x)
{
WriteLine("Enter name of customer {0} for exterior mural:", (x + 2));
string nameExterior = ReadLine();
exteriorMuralCustomers[0, x] = nameExterior;
do
{
WriteLine("Enter Mural code. Valid codes are L, S, A, C, and O,");
string code = ReadLine();
if (code == "L" || code == "S" || code == "A" || code == "C" || code == "O")
{
exteriorMuralCustomers[1, x] = code;
flag = false;
}
else
{WriteLine("{0} is not a valid code", code);
WriteLine("Invalid code. Valid codes are L, S, A, C, and O,");
flag = true;
}
}
while (flag);
}
int LcountExterior =0, ScountExterior =0, AcountExterior =0, CcountExterior =0, OcountExterior = 0;
for (x = 0; x < numExterior; ++x)
{
switch (exteriorMuralCustomers[1, x])
{
case "L":
LcountExterior++;
break;
case "S":
ScountExterior++;
break;
case "A":
AcountExterior++;
break;
case "C":
CcountExterior++;
break;
case "O":
OcountExterior++;
break;
}
WriteLine("The exterior murals scheduled are:");
WriteLine("Landscape {0}", LcountExterior);
WriteLine("Seascape {0}", ScountExterior);
WriteLine("Abstract {0}", AcountExterior);
WriteLine("Children's {0}", CcountExterior);
WriteLine("Other {0}\n\n", OcountExterior);
}
}



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

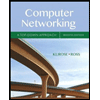
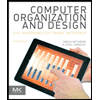
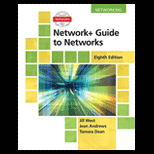
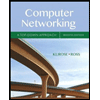
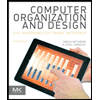
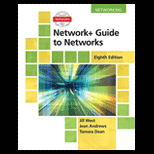
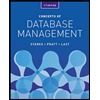
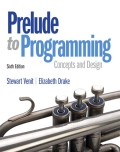
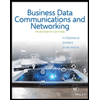