JAVA Only Design, implement and test a Java class that processes a series of triangles. The triangle specification will be identical to that in Programming Assignment #2 (part A). As in that assignment, you will read in the three sides of the triangle, check the input for errors (and handle them if found), classify the triangle and compute its area. For this assignment, triangle data will be read from an input file and the program’s output will be written to another file. The output file will also include a summary of the data processed. You must use at least one dialog box in this program. The data for each triangle will be on a separate line of the input file, with the input data echoed and the results referred to by the line number (see example). Some things to note: All input data lines must be processed. A new line with triangle data always starts with the ‘#’ marker. If an error is encountered, it must be handled and the program will continue. Extraneous data must be discarded. This is data that appears after the first three triangle sides are found, but before a new triangle marker is found. If the end of file (EOF) is found, a message should be printed out There shall be no unhandled file related exceptions and an end-of-file exception must be avoided. On initialization, the program will prompt the user for both an input and an output file. If a non-existent input file is specified, the appropriate exception must be handled, resulting in an error message. For the exception case, re-prompt the user for the correct input file [Extra credit if you implement a JFileChooser type dialog box]. Once the I/O file is specified, the program will read in and process all the entries in the file. The output of the program will be written to the specified output file and echoed to the console. The program will evaluate each line and determine the validity of the triangle. If valid, the program will output summary line for the triangle calculation (see Programming Assignment #2A). If invalid, the program will output a description of the error found in the entry. Your program must be robust and handle situations where all the triangle inputs are not proper and/or present. Once all the entries are processed, a summary will be generated providing the following information: Pathname of the input file Number of lines processed Number of valid triangles Number of invalid triangles You should be able to reuse much of your Programming Assignment #2A code for this project. However, strongly recommend that you rewrite your code and put this functionality in separate method(s). Likewise, any residual problems from #2A should be fixed in this version. The main features you will be adding are: Multiple methods Exception handing File I/O Dialog box Sample file input: # 3 4 5 # 5 5 5 # 5 5 7 XXX # -3 10 10 15 # 0 0 0 # 3 4.1 5 # 3 x 4 # 5 4 3 6 YYY ZZZ # 1 1 10 # 15 16 22 # 3 4 Corresponding file output: 1: 3, 4, 5: The triangle is a right triangle, The area of this triangle is 6.00 2: 5, 5, 5: The triangle is equilateral, The area of this triangle is 10.83 3: 5, 5, 7: The triangle is isosceles, The area of this triangle is 12.50 Item discarded: XXX Error - At least one of your sides is an invalid integer value Item discarded: 15 Error - At least one of your sides is an invalid integer value Error - At least one of your sides is not an integer Error - At least one of your sides is not an integer Error - Sides not in ascending order Item discarded: 6 Item discarded: YYY Item discarded: ZZZ Error - Sides do not form a valid triangle 4: 15, 16, 22: This triangle is not distinctive, The area of this triangle is 120.00
JAVA Only
Design, implement and test a Java class that processes a series of triangles. The triangle specification will be identical to that in
The data for each triangle will be on a separate line of the input file, with the input data echoed and the results referred to by the line number (see example).
Some things to note:
- All input data lines must be processed.
- A new line with triangle data always starts with the ‘#’ marker.
- If an error is encountered, it must be handled and the program will continue.
- Extraneous data must be discarded. This is data that appears after the first three triangle sides are found, but before a new triangle marker is found.
- If the end of file (EOF) is found, a message should be printed out
- There shall be no unhandled file related exceptions and an end-of-file exception must be avoided.
On initialization, the program will prompt the user for both an input and an output file. If a non-existent input file is specified, the appropriate exception must be handled, resulting in an error message. For the exception case, re-prompt the user for the correct input file [Extra credit if you implement a JFileChooser type dialog box]. Once the I/O file is specified, the program will read in and process all the entries in the file. The output of the program will be written to the specified output file and echoed to the console.
The program will evaluate each line and determine the validity of the triangle. If valid, the program will output summary line for the triangle calculation (see Programming Assignment #2A). If invalid, the program will output a description of the error found in the entry. Your program must be robust and handle situations where all the triangle inputs are not proper and/or present.
Once all the entries are processed, a summary will be generated providing the following information:
- Pathname of the input file
- Number of lines processed
- Number of valid triangles
- Number of invalid triangles
You should be able to reuse much of your Programming Assignment #2A code for this project. However, strongly recommend that you rewrite your code and put this functionality in separate method(s). Likewise, any residual problems from #2A should be fixed in this version. The main features you will be adding are:
- Multiple methods
- Exception handing
- File I/O
- Dialog box
Sample file input:
# 3 4 5
# 5 5 5
# 5 5 7 XXX
# -3 10 10 15
# 0 0 0
# 3 4.1 5
# 3 x 4
# 5 4 3 6 YYY ZZZ
# 1 1 10
# 15 16 22
# 3 4
Corresponding file output:
1: 3, 4, 5: The triangle is a right triangle,
The area of this triangle is 6.00
2: 5, 5, 5: The triangle is equilateral,
The area of this triangle is 10.83
3: 5, 5, 7: The triangle is isosceles,
The area of this triangle is 12.50
Item discarded: XXX
Error - At least one of your sides is an invalid integer value
Item discarded: 15
Error - At least one of your sides is an invalid integer value
Error - At least one of your sides is not an integer
Error - At least one of your sides is not an integer
Error - Sides not in ascending order
Item discarded: 6
Item discarded: YYY
Item discarded: ZZZ
Error - Sides do not form a valid triangle
4: 15, 16, 22: This triangle is not distinctive,
The area of this triangle is 120.00
EOF found
File Path: E:\Java\Assign-05\pa5-test-01.txt
--------------- Summary Report ---------------
Total entries processed = 10
Number of valid entries = 4
Number of invalid entries = 6
Percentage of valid entries = 40.00%

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 8 images

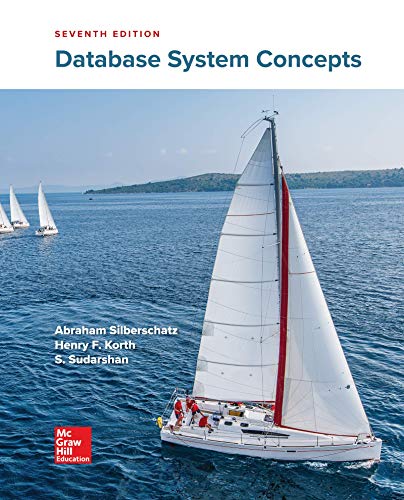
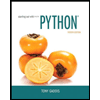
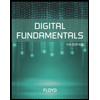
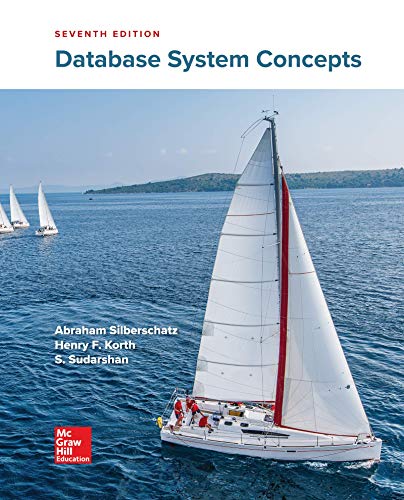
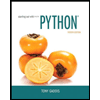
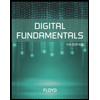
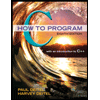
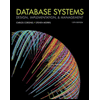
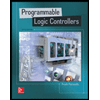