in C# i need to Create a BankAccount class with the following properties: Account Number (string) Account Holder Name (string) Balance (double) Account Type (enum: Savings, Current) Create the following methods in the BankAccount class: Deposit(double amount): Method to deposit money into the account Withdraw(double amount): Method to withdraw money from the account CheckBalance(): Method to check the current balance of the account Create a Bank class with the following properties: List of bank accounts (List) Create the following methods in the Bank class: AddAccount(BankAccount account): Method to add a new bank account to the list of accounts RemoveAccount(string accountNumber): Method to remove an existing bank account from the list of accounts SearchAccount(string accountNumber): Method to search for an existing bank account and return the account details Create a Program class with the Main method, and implement the following functionality: Create an instance of the Bank class Add at least 3 bank accounts to the list of accounts Search for an existing account and display the account details Deposit an amount into one of the accounts Withdraw an amount from one of the accounts Search for the account again and display the updated account details Remove one of the accounts Note: To test the functionality of the BankAccount and Bank classes you can create multiple instances of the classes and test the methods Add appropriate input validation (remember: an example of input validation would be typing a letter when the program is asking for a number, and instead of the program crashing, it simply disregards the letter and asks for a correct value) wherever necessary to make sure the methods are working correctly. Add some comments to the code to explain what each block of code is doing. Add some test cases to test the implemented functionality
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
in C# i need to
-
Create a BankAccount class with the following properties:
- Account Number (string)
- Account Holder Name (string)
- Balance (double)
- Account Type (enum: Savings, Current)
-
Create the following methods in the BankAccount class:
- Deposit(double amount): Method to deposit money into the account
- Withdraw(double amount): Method to withdraw money from the account
- CheckBalance(): Method to check the current balance of the account
-
Create a Bank class with the following properties:
- List of bank accounts (List<BankAccount>)
-
Create the following methods in the Bank class:
- AddAccount(BankAccount account): Method to add a new bank account to the list of accounts
- RemoveAccount(string accountNumber): Method to remove an existing bank account from the list of accounts
- SearchAccount(string accountNumber): Method to search for an existing bank account and return the account details
-
Create a Program class with the Main method, and implement the following functionality:
- Create an instance of the Bank class
- Add at least 3 bank accounts to the list of accounts
- Search for an existing account and display the account details
- Deposit an amount into one of the accounts
- Withdraw an amount from one of the accounts
- Search for the account again and display the updated account details
- Remove one of the accounts
Note: To test the functionality of the BankAccount and Bank classes you can create multiple instances of the classes and test the methods
-
Add appropriate input validation (remember: an example of input validation would be typing a letter when the program is asking for a number, and instead of the program crashing, it simply disregards the letter and asks for a correct value) wherever necessary to make sure the methods are working correctly.
-
Add some comments to the code to explain what each block of code is doing.
-
Add some test cases to test the implemented functionality

Below please find implementation of the BankAccount, Bank, and Program classes in C#:
Trending now
This is a popular solution!
Step by step
Solved in 5 steps

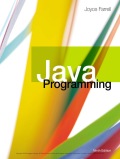
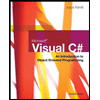
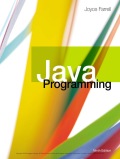
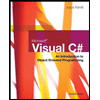