i, this is a java programming question with direction of what to do. I'm just gonna attach the question sheet. Implement the following class diagram (diagram in attached photo) setFirstName() method should set the firstName attribute of the class. setLastName() method should set the lastName attribute of the class. setSpecialization() method should set the specialization attribute of the Doctor class. setHealthnumber() method should set the healthNumber attribute of the Patient class. setIllness() method should set the illness attribute of the Patient class. If you are not comfortable with set methods, you are free to create your own constructors to set the attribute values.
Hi, this is a java programming question with direction of what to do. I'm just gonna attach the question sheet.
Implement the following class diagram
(diagram in attached photo)
setFirstName() method should set the firstName attribute of the class.
setLastName() method should set the lastName attribute of the class.
setSpecialization() method should set the specialization attribute of the Doctor class.
setHealthnumber() method should set the healthNumber attribute of the Patient class.
setIllness() method should set the illness attribute of the Patient class.
If you are not comfortable with set methods, you are free to create your own constructors to set the
attribute values.
Create a HospitalApplication class which contains the main method. Inside the main method the
following should be done in the order mentioned. (Any other ordering would not guarantee full marks).
Prompt for the following inputs for 5 Doctors. (Use for loops)
• First name
• Last name
• Specialization
Valid specializations are:
• General Physician
• Neurologist
• Nephrologist
• Cardiologist
The specializations are case insensitive (General physician, General Physician and GENERAL PHYSICIAN
should all be valid.)
If the user enters an invalid specialization
• Print “You entered an invalid specialization”
• Keep prompting the user to enter a valid specialization.
The getName() method should:
• Concatenate the first name and last name with a space between them
• The first letter of the first and last names should be in capitals
• Should return the formatted name
The getSpecialization() method should:
• Capitalize the first letter of specialization and return the specialization
For all the doctors, print the summary (using loops) in the following format:
Name followed by a tab (\t) and then the specialization. For example, if the first doctor entered was:
firstName: John
lastName: Doe
specialization: Cardiologist
The summary should print
Dr. John Doe Cardiologist
And so on for all the 5 doctors
Use an infinite loop to get the following patient information:
First name
Last name
PHN (Personal Health Number)
Illness
PHN should:
• Contain 10 digits to be valid
• Begin with 9 to be valid
If the user enters an invalid PHN:
• Print “You entered an invalid PHN”
• Keep prompting the user to enter a valid PHN.
The illnesses are case insensitive.
Use the following table to identify which doctor can cure the given illness for the patient.
(image in 2nd attchment)
When the user enters valid values:
• Create a patient object with the passed information
• print the summary as follows (using the patient object):
firstName: Stan
lastName: Smith
PHN: 9999999999
Illness: Heart issue
Stan Smith with PHN 9999999999 has Heart issue
You can be treated by Dr. John Doe
• If there are multiple doctors available for a particular illness, then print all their names
separated by commas (You can be treated by Dr. John Doe, Dr. John David).
• If there are no doctors available for a particular illness, then print “Sorry, no doctors available”



Step by step
Solved in 4 steps with 6 images

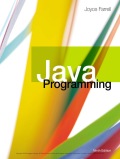
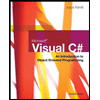
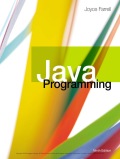
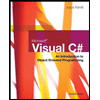