Edit only the class definition. DO NOT CHANGE the code given under 'main' please. Steps: Additionally implement any Python Magic/Dunder methods such that instances of the class minimally: 1. Support addition, subtraction, equality operations and the built-in abs function 2. Are Iterable i.e., support for loops and star arguments for unpacking into function calls 3. Support a string representation that displays the class name and coordinates stored by the instance: i.e. for an object initialized as: Vector(0, 3), the string representation should be ‘Vector(0, 3)’ The code given under main tests for each of the program requirements and subsequently uses the turtle module to plot randomly generated points rotated. A screenshot for a sample run of the program is attached. Template.py: from math import hypot, pi from random import uniform import turtle as t class Vector2D: ... if __name__ == '__main__': # Test Vector class a, b = Vector2D(0, 3), Vector2D(0, -3) tests = [(str(a), 'Vector2D(0, 3)'), (repr(a), 'Vector2D(0, 3)'), (a+b, Vector2D(0, 0)), (a-b, Vector2D(0, 6)), (abs(a), hypot(*a)), (a.dot(b), -9), (a.scale(3), Vector2D(0, 9)), (a.direction(), Vector2D(0.0, 1)), (a.distance(b), 6), (a.rotate(pi), b), ([x for x in a], list(a)), (tuple(a), (0, 3))] for i, test in enumerate(tests, 1): assert test[0] == test[1], f'Test {i}: FAIL {test[0]} != {test[1]}' # All tests passed !!! # Start drawing t.setworldcoordinates(-150, -150, 150, 150) t.title('We Spin') t.tracer(1000) t.pu() # generate 100 points on y-axis between -50 and 50 points = [Vector2D(uniform(-50, 50), 0) for i in range(100)] # generate 20000 new points by transforming initial points spin_points = [p.rotate(400/i*pi)+p.scale(2) for p in points for i in range(1, 201)] # plot spin points for p in spin_points: t.goto(*p) t.pencolor(uniform(0, 1), uniform(0, 1), uniform(0, 1)) t.dot(5) t.done()
Edit only the class definition. DO NOT CHANGE the code given under 'main' please.
Steps:
Additionally implement any Python Magic/Dunder methods such that instances of the class minimally:
1. Support addition, subtraction, equality operations and the built-in abs function
2. Are Iterable i.e., support for loops and star arguments for unpacking into function calls
3. Support a string representation that displays the class name and coordinates stored by the instance: i.e. for an object initialized as:
The code given under main tests for each of the program requirements and subsequently uses the turtle module to plot randomly generated points rotated. A screenshot for a sample run of the program is attached.
Template.py:


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

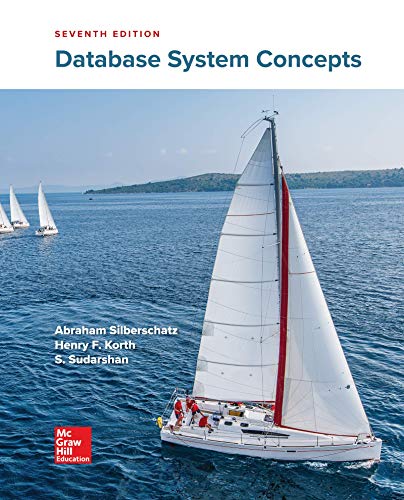
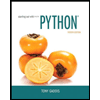
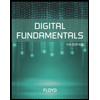
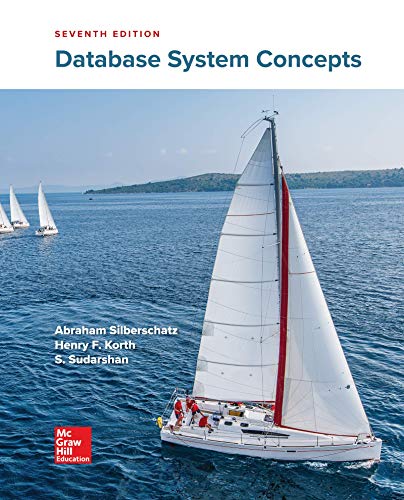
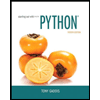
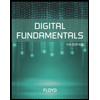
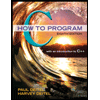
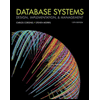
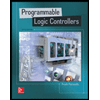