Description: In this project, you are required to make a java code to build a blockchain including multiple blocks. In each block, it contains a list of transactions, previous hash - the digital signature of the previous block, and the hash of the current block which is based on the list of transactions and the previous hash. If anyone would change anything with the previous block, the digital signature of the current block will change. When this changes, the digital signature of the next block will change. Detailed Requirements: 1. Define your block class which contains three private members and four public methods. a. Members: previousHash (int), transactions (String[]), and blockHash (int); b. Methods: i. Block( int previousHash, String[] transactions){} ii. getPreviousHash(){} iii. getTransaction(){} iv. getBlockHash(){} 2. Using ArrayList to design your Blockchain. 3. Your blockchain must contain genesis block and make it to be the first block. 4. You can add a block to the end of the blockchain. When a new block is added, it first must point to the last block in your blockchain, and can allow a user to add any number of transactions until the user click "done" 5. In each transaction, please use the following format "Santoshi (sender) pays x (number) BTC to Ivan (receiver)". Put each transaction in a string. 6. You can display the transactions, previous hash, and the current hash of any selected block. Please use 1, 2, 3,... to select a block. "1" indicates the genesis block. If a selected block does not exist, please display "the block does not exist in this Blockchain". 7. Edit the transactions in a selected block, but you must verify and show it will break the consistency with the "previousHash" in the block after this block. 8. Display the whole blockchain with the contents in each block. 1. Two screenshots to show the running results. 2. Read me with name "readme.txt" to show how to run/operate your code. 3. Java source code. Sample output: When you run the code, please display the following menu, 1. Add a block; 2. Display a block; 3. Edit a Block; 4. Display the whole blockchain If "1" is selected, display which block it is to be added to the end of the chain, Then display previous hash, current hash, and transactions. Each transaction must follow the following format. Satoshi pays 10 BTC to Ivan; Sam pas 20 BTC to Jeff; .... Display a submenu 1.1 Submit 1.2 refresh If 1.1 is selected, add the current block to the block chain. If 1.2 is selected, recalculate previous hash, current hash. If transactions are edited or added, it needs refresh first before submit it. If 2 is selected, please remind the user to input the block number, such as 4, then you code can print out Block 5. Block 5: Previous Hash: A3DEA0B0C148992ACEF3659 Current Hash: xxxxxxxxxxxxxx Transactions: Satoshi pays 5 BTC to Ivan; Sam pas 10000 BTC to Jeff; If 3 is selected, please ask the user to input a Block number, such as 14, then display Block 14 contents. Block 14: Previous Hash: 03DEA0B0C148992ACEF3659 (cannot be edited) Current Hash: xxxxxxxxxxxxxx (cannot be edited) Transactions: Satoshi pays 10 BTC to Ivan; Sam pas 20 BTC to Jeff; (for editing) Display a submit menu: 3.1 Submit. As long as you change any transaction information, the current hash must be changed. If you submit the changes, please check the previous hash of the block after this block to see if they are consistent. If 4 is selected, please print out the whole block chain from the first block to the last one as the following format. Block 1: Previous Hash: 03DEA0B0C148992ACEF3659 Current Hash: xxxxxxxxxxxxxx Transactions: Satoshi pays 10 BTC to Ivan; Sam pas 20 BTC to Jeff; Block 2: Previous Hash: A3DEA0B0C148992ACEF3659 Current Hash: xxxxxxxxxxxxxx Transactions: Satoshi pays 5 BTC to Ivan; Sam pas 10000 BTC to Jeff; Blcok 3: ....
Description: In this project, you are required to make a java code to build a blockchain including multiple blocks. In each block, it contains a list of transactions, previous hash - the digital signature of the previous block, and the hash of the current block which is based on the list of transactions and the previous hash. If anyone would change anything with the previous block, the digital signature of the current block will change. When this changes, the digital signature of the next block will change.
Detailed Requirements: 1. Define your block class which contains three private members and four public methods. a. Members: previousHash (int), transactions (String[]), and blockHash (int); b. Methods: i. Block( int previousHash, String[] transactions){} ii. getPreviousHash(){} iii. getTransaction(){} iv. getBlockHash(){}
2. Using ArrayList to design your Blockchain.
3. Your blockchain must contain genesis block and make it to be the first block.
4. You can add a block to the end of the blockchain. When a new block is added, it first must point to the last block in your blockchain, and can allow a user to add any number of transactions until the user click "done"
5. In each transaction, please use the following format "Santoshi (sender) pays x (number) BTC to Ivan (receiver)". Put each transaction in a string.
6. You can display the transactions, previous hash, and the current hash of any selected block. Please use 1, 2, 3,... to select a block. "1" indicates the genesis block. If a selected block does not exist, please display "the block does not exist in this Blockchain".
7. Edit the transactions in a selected block, but you must verify and show it will break the consistency with the "previousHash" in the block after this block.
8. Display the whole blockchain with the contents in each block.
1. Two screenshots to show the running results.
2. Read me with name "readme.txt" to show how to run/operate your code.
3. Java source code.
Sample output:
When you run the code, please display the following menu,
1. Add a block; 2. Display a block; 3. Edit a Block; 4. Display the whole blockchain
If "1" is selected, display which block it is to be added to the end of the chain,
Then display previous hash, current hash, and transactions. Each transaction must follow the following format.
Satoshi pays 10 BTC to Ivan; Sam pas 20 BTC to Jeff; ....
Display a submenu 1.1 Submit 1.2 refresh
If 1.1 is selected, add the current block to the block chain. If 1.2 is selected, recalculate previous hash, current hash. If transactions are edited or added, it needs refresh first before submit it.
If 2 is selected, please remind the user to input the block number, such as 4, then you code can print out Block 5.
Block 5:
Previous Hash: A3DEA0B0C148992ACEF3659
Current Hash: xxxxxxxxxxxxxx
Transactions: Satoshi pays 5 BTC to Ivan; Sam pas 10000 BTC to Jeff;
If 3 is selected, please ask the user to input a Block number, such as 14, then display Block 14 contents.
Block 14:
Previous Hash: 03DEA0B0C148992ACEF3659 (cannot be edited)
Current Hash: xxxxxxxxxxxxxx (cannot be edited)
Transactions: Satoshi pays 10 BTC to Ivan; Sam pas 20 BTC to Jeff; (for editing)
Display a submit menu: 3.1 Submit.
As long as you change any transaction information, the current hash must be changed. If you submit the changes, please check the previous hash of the block after this block to see if they are consistent.
If 4 is selected, please print out the whole block chain from the first block to the last one as the following format.
Block 1:
Previous Hash: 03DEA0B0C148992ACEF3659
Current Hash: xxxxxxxxxxxxxx
Transactions: Satoshi pays 10 BTC to Ivan; Sam pas 20 BTC to Jeff;
Block 2:
Previous Hash: A3DEA0B0C148992ACEF3659
Current Hash: xxxxxxxxxxxxxx
Transactions: Satoshi pays 5 BTC to Ivan; Sam pas 10000 BTC to Jeff;
Blcok 3:
....

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

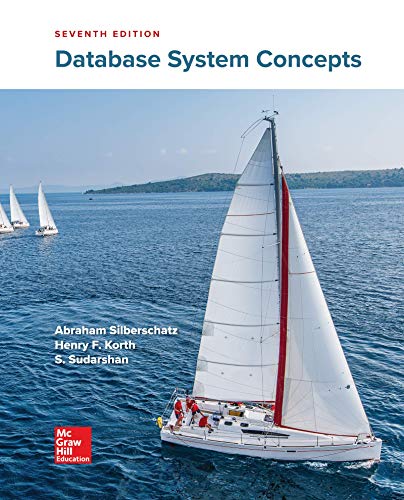
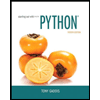
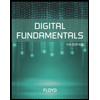
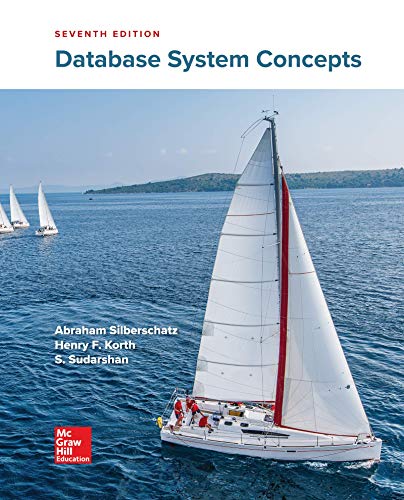
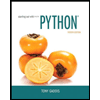
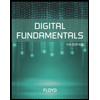
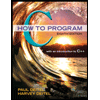
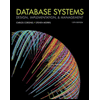
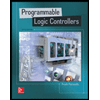