ava code Objectives •Use an abstract data type for a list •Use a method that contains an Object type as a formal parameter •Use methods in an existing class •Access data members within a class Required Files: •ListInterface.java •ListException.java •ListOutOfBoundsException.java •ListArrayBased.java Description A list is an abstract data type used to st
Write Java code
Objectives
•Use an abstract data type for a list
•Use a method that contains an Object type as a formal parameter
•Use methods in an existing class
•Access data members within a class
Required Files:
•ListInterface.java
•ListException.java
•ListOutOfBoundsException.java
•ListArrayBased.java
Description A list is an abstract data type used to store ordered data, and contains operations that fall into the following categories: (1) add data to a data collection, (2) remove data from a data collection, and (3) ask questions about data in a data collection. The operations which ask questions about the data in a data collection may include operations that determine if a data collection is empty, determine the size of a data collection, and retrieve the item at a given position in a data collection. A list should be able to contain any type of object. The methods for the List support any object as specified in the interface. In this assignment, the objects will be S trings.
class ListDriver
•The purpose of the ListDriver class is to perform ADT list operations on a list of objects of type String.
•The data that is to be used for the list is located in an array that is hard coded into the main method, which should include the name of each the grocery item from the example used in our discussion on the ADT: List. •An instance of ListArrayBased is passed to various methods within the ListDriver class along with other arguments needed for the method to perform its task. Appropriate comments are included for each method. •You will add code to the various methods in the ListDriver class so that each one performs its task as specified.
part 3 of the code in the pictures
* position pos. */
public void removeItem(ListArrayBased list, int pos){
TO DO: add code here
}
/* * removeAllItems *
* Precondition: a reference to a list *
* Postcondition: all items currently stored in the list * are removed */
public void removeAllItems(ListArrayBased list){
// TO DO: add code here
}
} // end of class ListArrayBasedDriver
![Postconditions:
* "List empty: B" where B is either TRUE or FALSE
* and "Size of list: n" where n is the size of
* the list is written to std out.
public void displaystatus(ListarrayBased list)
{
// TO DO: add code here
}
* displaylist
* Precondition: a reference to a list
*
* Postcondition: list is displayed on std out
*/
public void displaylist(ListarrayBased list)
{
// TO DO: add code here
* buildlist
* Precondition: a reference to a list and an string array
* of items to be address to the list
* Postcondition: items stored the string array are added
* to the list.
* /
public void buildlist(ListarrayBased list, string[] items)
{
// TO DO: add code here
}
* addItem
*
* Precondition: a reference to a list, a string
* representing a grocery item, and the integer
pos is the position in the list
*
* Postcondition: an item is added to the list at
* position pos.
* /
public void addItem(ListarrayBased list, string item, int pos)
{
// TO DO: add code here
}
то
* removeItem
*
* Precondition: a reference to a list and
* int pos;
* Postcondition: item is removed from the list by its](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff27854cd-5d9f-4553-a6c0-a306ccf4a4ef%2Fca54251e-7868-419c-a6e0-073dad35a691%2Fh1cbuag_processed.png&w=3840&q=75)
![//package PA02
/*
File: Listoriver.java
* ASsignment: Programming Assignment 2
Author:
Date:
Course:
Instructor:
Purpose: Test an implementation of an array-based list ADT.
To demonstrate the use of "ListArrayBased" the project driver
* class will populate the list, then invoke various operations
* of "ListarrayBased".
*/
import java.util.scanner;
public class Listoriver {
* main
*
* An array based list is populated with data that is stored
* in a string array. User input is retrieved from that std in.
* A text based menu is displayed that contains a number of options.
* The user is prompted to choose one of the available options:
* (1) Build List, (2) Add item, (3) Remove item, (4) Remove all items,
* or (5) done.
* appropriate method based on the option chosen by the user, and
* prompts the user for further input as required Program terminates
* if user chooses option (5). If the user chooses an option that is
* not in the menu, a message telling the user to choose an appropriate
* option is written to std out, followed by the options menu.
The switch statement manages calling the
*/
public static void main(string[] args)
{
String[] dataItems =
{"milk", "eggs", "butter", "apples", "bread", "chicken"};
// TO DO: add code here
} // end of main method
/*
* Displays the options menu, including the prompt
* for the user
*/
public void displayMenu()
{
// TODO: add code here
}
* displaystatus
* displays information about the state of
* the list
*
* Preconditions: a reference to a list](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff27854cd-5d9f-4553-a6c0-a306ccf4a4ef%2Fca54251e-7868-419c-a6e0-073dad35a691%2Ft3q4f3g_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

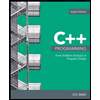
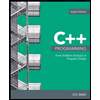