A text file called hours.txt has been provided. Copy the text file into the folder of the project. The lines of text in the text file contain the surname, list of hours spent on Facebook per day over a period of 7 days (one week) and job title of a number of employees. Example of the content of the text file: Simon 8234556 Manager Johnson 1020103 Clerk Peterson 6238126 Assistant Pule 4568752 Manager Gumede 4536776 Clerk Green 2310211 Cleaner Mokoena 7683587 Manager Fourie 4342742 Clerk *End of the text file *Note: Three lines of text contain the information of one employee. Declare an array of Employee structs called arrEmp that must be able to hold the information of up to 30 employees. An employee struct must consist of the following fields: surname, hours (integer) and manager (bool). Use the following declaration (this declaration is compulsory and must be used): struct employee { char surname [20]; int hours; bool manager; } ; Write a method called readFromFile() that will receive the array and number of elements as parameters. Read the data from the text file into the array. The Facebook hours must be read as an integer into the hours-field. If the job title is a manager, the Boolean value of true must be assigned to the managerfield. Otherwise the value of false must be assigned to the manager-field. Return the number of elements (employees) saved in the array. Note: The information of one employee is saved in three lines of text in the file. Therefore you need three read instructions per employee. Write a function called displayInfo() that will receive the array and the number of elements in the array. Display the list of employees as shown in the example. Example of output (displayinfo.png): Attached Image. Write a function called calcTotal() that will receive an integer value (e.g. 8234526) and calculate and return the total number of Facebook hours for the employee. NOTE: To calculate the total number of hours get each individual digit contained in the value received by the function and add up the individual digit-values. For this purpose use the % operator and / operator repetitively inside a for loop. Write a function called displayTotalHours() that will receive the array and the number of elements saved in the array as parameters. Inside a for loop, call the calcTotal() function to obtain the total hours for each employee and display a numbered list with the surnames and total number of hours spent on Facebook for each employee. Calculate and return the total number of hours spent on Facebook by all the employees. Example (totalhours.png): Attached File
A text file called hours.txt has been provided. Copy the text file into the folder of the project.
The lines of text in the text file contain the surname, list of hours spent on Facebook per day over a period of 7 days (one week) and job title of a number of employees.
Example of the content of the text file:
Simon
8234556
Manager
Johnson
1020103
Clerk
Peterson
6238126
Assistant
Pule
4568752
Manager
Gumede
4536776
Clerk
Green
2310211
Cleaner
Mokoena
7683587
Manager
Fourie
4342742
Clerk
*End of the text file
*Note: Three lines of text contain the information of one employee.
Declare an array of Employee structs called arrEmp that must be able to hold the information of up to 30 employees. An employee struct must consist of the following fields: surname, hours (integer) and manager (bool).
Use the following declaration (this declaration is compulsory and must be used):
struct employee
{
char surname [20];
int hours;
bool manager;
} ;
Write a method called readFromFile() that will receive the array and number of elements as parameters.
Read the data from the text file into the array. The Facebook hours must be read as an integer into the hours-field. If the job title is a manager, the Boolean value of true must be assigned to the managerfield.
Otherwise the value of false must be assigned to the manager-field. Return the number of elements (employees) saved in the array.
Note: The information of one employee is saved in three lines of text in the file. Therefore you need three read instructions per employee.
Write a function called displayInfo() that will receive the array and the number of elements in the array. Display the list of employees as shown in the example. Example of output (displayinfo.png): Attached Image.
Write a function called calcTotal() that will receive an integer value (e.g. 8234526) and calculate and return the total number of Facebook hours for the employee.
NOTE: To calculate the total number of hours get each individual digit contained in the value received by the function and add up the individual digit-values. For this purpose use the % operator and / operator repetitively inside a for loop.
Write a function called displayTotalHours() that will receive the array and the number of elements saved in the array as parameters. Inside a for loop, call the calcTotal() function to obtain the total hours for each employee and display a numbered list with the surnames and total number of hours spent on Facebook for each employee. Calculate and return the total number of hours spent on Facebook by all the employees.
Example (totalhours.png): Attached File



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

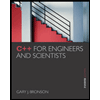
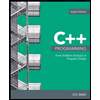
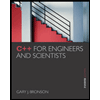
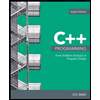