COMS W3134 - Spring 2024 - Theory 3 - Answer Key
.pdf
keyboard_arrow_up
School
Columbia University *
*We aren’t endorsed by this school
Course
3134
Subject
Computer Science
Date
May 8, 2024
Type
Pages
11
Uploaded by DeanRamMaster2400 on coursehero.com
COMS W3134, Theory Homework 3, Spring 2024
.Name & UNI: _______________________________
Date:
__________________
Point values are assigned for each question.
Points earned: ____ / 100, = ____ %
1.
Assume you have a binary search tree with no duplicate values. The method below,
findDifference,
will find the greatest difference between
any two nodes on the tree. You may
not add any additional lines to the functions, since everything you need is given. A
TreeNode
is
defined as follows:
class TreeNode {
public int
value
;
public TreeNode left, right;
}
Fill in the blanks for
findDifference
,
findMin
, and
findMax
to calculate the greatest
difference between nodes in a binary search tree. (12 points)
public static int findMin(TreeNode root) {
if (__A__) {
return root.value;
}
return __B__;
}
public static int findMax(TreeNode root) {
if (__C__) {
return root.value;
}
return __D__;
}
/**
* Finds the greatest difference between nodes in a
* binary search tree.
*/
public static int findDifference(TreeNode root) {
if (__E__) {
return 0;
}
return __F__;
}
A.
root.left == null
B.
findMin(root.left)
C.
root.right == null
D.
findMax(root.right)
E.
root == null
COMS W3134, Theory Homework 3, Spring 2024
F.
findMax(root) - findMin(root)
2.
Give an iterative algorithm in Java to visit all the nodes in a binary tree with a postorder traversal.
Same as above, nodes have
left
and
right
references as well as a
value
field. Each time a
Node is visited, your solution will print its value.
Write your solution below. (10 points)
public static void iterativePostorder(Node root) {
if (root == null) return;
MyStack<Node> stack = new MyArrayList<>();
Node current = root;
Node lastVisited = null;
while (current != null || !stack.__A__) {
if (current != null) {
stack.push(__B__);
current = current.left;
} else {
Node peekNode = stack.peek().right;
if (peekNode == null || peekNode == lastVisited) {
Node poppedNode = __C__;
System.out.println(__D__);
lastVisited = __E__;
} else {
current = peekNode;
}
}
}
}
A. isEmpty()or empty()
B. current
C. stack.pop()
D.
poppedNode.value
E. poppedNode
COMS W3134, Theory Homework 3, Spring 2024
full method:
public static void iterativePostorder(Node root) {
if (root == null) return;
MyStack<Node> stack = new MyArrayList<>();
Node current = root;
Node lastVisited = null;
while (current != null || !stack.isEmpty()) {
if (current != null) {
stack.push(current);
current = current.left;
} else {
Node peekNode = stack.peek().right;
if (peekNode == null || peekNode == lastVisited) {
Node poppedNode = stack.pop();
System.out.println(poppedNode.value);
lastVisited = poppedNode;
} else {
current = peekNode;
}
}
}
}
3.
You are given the preorder and inorder traversals of a binary tree of integers that contains no
duplicates. Using this information, reconstruct the binary tree and draw it. (10 points)
Inorder: [6, 2, 9, 4, 3, 8, 1, 5, 7, 0]
Preorder: [3, 9, 6, 2, 4, 1, 8, 7, 5, 0]
Solution
3
/ \
/
\
/
\
9
1
/ \
/ \
6
4
8
7
\
/ \
2
5
0
COMS W3134, Theory Homework 3, Spring 2024
4.
One of the properties for red-black trees is that for any given node, the path to its descendant leaves
contains the same amount of black nodes. This property is known as the black-heigh of the tree. The
recursive function below, checkBlackHeight, takes in a node in a red-black tree and true if the node
satisfies the black-height property, and false if it does not.
Fill in the blanks to the function given here. (10 points)
// Helper function that returns the black height for any node
public static int blackHeight(RBNode<K, V> node) {
if (node == null) {
return 0;
}
int leftHeight = blackHeight(node.left);
int rightHeight = blackHeight(node.right);
// If the current node is black, add 1 to the height
return ((node.color == RBNode.BLACK) ? 1 : 0) + Math.max(__A__);
}
// Function to check if a node satisfies the black-height property
public static boolean blackHeightEquality(RBNode<K, V> node) {
// Base case: a leaf node satisfies the black-height property
if (__B__) {
return true;
}
// Get the black height of the left and right subtrees
int leftHeight = blackHeight(node.left);
int rightHeight = blackHeight(node.right);
// Check if the black heights of left and right subtrees are equal
if (__C__) {
// Recursively check if both left and right subtrees satisfy
// the black-height property
return ___D___ && ___E___;
}
return false;
}
Solution
A.
leftHeight, rightHeight
B.
node == null
C.
leftHeight == rightHeight
D.
blackHeightEquality(node.right) OR blackHeightEquality(node.left)
E.
blackHeightEquality(node.right) OR blackHeightEquality(node.left)
(depending on which was called first)
5.
Using structural induction, prove that the number of leaves in a non-empty full binary tree is one
more than the number of internal nodes. (10 points)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
CODE :
def days_in_feb(user_year): is_leap_year = 29 not_leap_year = 28 if user_year % 4 ==0: return is_leap_year elif user_year % 4 ==0 and user_year % 100 == 0 and user_year % 400 == 0: return is_leap_year else: return(not_leap_year) if __name__ == '__main__': # Type your code here. Your code must call the function. user_year = int(input()) days = days_in_feb(user_year) print(user_year,'has', days, 'days in February.')
ERROR
OUTPUT IS NOT WORKING FOR YEAR 1900
Input
1712
Your output
1712 has 29 days in February.
Your output
days_in_feb(1913) correctly returned 28
days_in_feb(1600)
Your output
days_in_feb(1600) correctly returned 29
4: Unit testkeyboard_arrow_up
0 / 3
days_in_feb(1900)
Your output
days_in_feb(1900) incorrectly returned 29
arrow_forward
{
"name": "Renata Severson",
"dob": "2017-02-22",
"address": {
},
"street": "4228 Lucy Circle",
"town": "Ayr",
"postode" : "PL01 3MG"
"telephone": "+971-6808-178-469",
"score": 10,
"email": "brianna71@add.hn",
"verified": false,
"salary" : 50307
JSON Validation
Valid: Yes
If no, reason(s)
XX
arrow_forward
Some rows of the STUGRADE table of a school are shown below:
STU_CODE
CLS_CODE
GRADE
YEAR
291
111
3.1
2010
938
101B
2.3
2011
931
M101
3.3
2040
291
M101
3.4
2018
190
111
2.0
2021
931
321
3.9
2003
Suppose that STU_CODE is the student code (foreign key) and CLS_CODE is the class code (foreign key). Which of the following computes the average for each year of the student with code 931?
a.
SELECT AVG(GRADE) FROM STUGRADE WHERE STU_CODE = 931 GROUP BY YEAR
b.
SELECT AVG(GRADE) FROM STUGRADE WHERE STU_CODE = 931.
c.
SELECT AVG(GRADE) FROM STUGRADE GROUP BY STU_CODE = 931
d.
SELECT AVG(GRADE) FROM STUGRADE WHERE STU_CODE = 931 GROUP BY AVG(GRADE)
arrow_forward
Whoever must play, cannot play def subtract_square(queries):
Two players play a game of "Subtract a square", starting with a positive integer. On their turn to play, each player must subtract some square number (1, 4, 9, 16, 25, 36, 49, ...) from the current number so that the result does not become negative. Under the normal play convention of these games, the player who moves to zero wins, leaving his opponent stuck with no possible moves. (In the misère version of the game that has otherwise identical rules but where you win by losing the original game, these definitions would be adjusted accordingly.)
This and many similar combinatorial games can be modelled with recursive equations. This game is an example of an impartial game since the moves available to both players are exactly the same, as opposed to “partisan games” such as chess where each player can only move pieces of one colour. A game state is therefore determined by the remaining number alone, but not by which player has…
arrow_forward
"song1": {
"genre": "g1",
"album": "a1",
"rating": "r1",
"other": "some data1"
The songs.json
},
"song2": {
"genre": "g2",
"album":"a2",
"rating": "r2", "other": "some data2"
},
"song3": {
"genre": "g3",
"album": "a3",
"rating": "r3",
"other": "some data3"
},
"song4": {
"genre": "g4",
"album":"a4",
"rating": "r4",
"other": "some data4"
}
}
arrow_forward
Create an application that finds the greatest common divisor of two positive integers entered by the user.
Console
Greatest Common Divisor Finder
Enter first number: 12
Enter second number: 8
Greatest common divisor: 4
Continue? (y/n): y
Enter first number: 77
Enter second number: 33
Greatest common divisor: 11
Continue? (y/n): y
Enter first number: 441
Enter second number: 252
Greatest common divisor: 63
Continue? (y/n): n
Specifications
The formula for finding the greatest common divisor of two positive integers x and y must follow the Euclidean algorithm as follows:
Subtract x from y repeatedly until y < x.
Swap the values of x and y.
Repeat steps 1 and 2 until x = 0.
y is the greatest common divisor of the two numbers.
You can use one loop for step 1 of the algorithm nested within a second loop for step 3.
Assume that the user will enter valid integers for both numbers.
The application should continue only if the user enters 'y' or 'Y' to continue. Note that if you do…
arrow_forward
✓ Exercises
Exercise: Write a method _ and _ that computes the region representing the intersection of two regions.
Exercise: Write a method
_contains__, which checks whether a n-dimensional point belongs to the region. Remember, a point belongs to the
region if it belongs to one of the rectangles in the region.
✓ Membership of a point in a region
#@title Membership of a point in a region
def region_contains (self, p):
### YOUR SOLUTION HERE
Region._contains = region_contains
[ ] # Tests 10 points.
assert (2, 1) in Region (Rectangle((0, 2), (0, 3)), Rectangle((4, 6), (5,8)))
assert (2, 1) not in Region (Rectangle((0, 1), (0, 3)), Rectangle((4, 6), (5, 8)))
Exercise: Write a method _le_ for regions such that R <= S if the region R is contained in the region S. You can test this by checking that
the difference between R and S is empty.
arrow_forward
The constants are:
COLUMN_ID = 0COLUMN_NAME = 1COLUMN_HIGHWAY = 2COLUMN_LAT = 3COLUMN_LON = 4COLUMN_YEAR_BUILT = 5COLUMN_LAST_MAJOR_REHAB = 6COLUMN_LAST_MINOR_REHAB = 7COLUMN_NUM_SPANS = 8COLUMN_SPAN_DETAILS = 9COLUMN_DECK_LENGTH = 10COLUMN_LAST_INSPECTED = 11COLUMN_BCI = 12
INDEX_BCI_YEARS = 0INDEX_BCI_SCORES = 1MISSING_BCI = -1.0
EARTH_RADIUS = 6371
This is an example of one of the bridges which is a list.
def create_example_bridge_1() -> list:"""Return a bridge in our list-format to use for doctest examples.
This bridge is the same as the bridge from row three of the dataset."""
return [1, 'Highway 24 Underpass at Highway 403','403', 43.167233, -80.275567, '1965', '2014', '2009', 4,[12.0, 19.0, 21.0, 12.0], 65.0, '04/13/2012',[['2013', '2012', '2011', '2010', '2009', '2008', '2007','2006', '2005', '2004', '2003', '2002', '2001', '2000'],[MISSING_BCI, 72.3, MISSING_BCI, 69.5, MISSING_BCI, 70.0, MISSING_BCI,70.3, MISSING_BCI, 70.5, MISSING_BCI, 70.7, 72.9, MISSING_BCI]]]
def…
arrow_forward
Help code.
arrow_forward
Employee ( Eid, Ename,EAge, ECity, E_Street, E_House#, E_Basic_Salary, E_Bonus,E_Rank, E_Department)
Eid is Primary
E_Rank can be “Manager, Developer, Admin”
E_Department can be “HR, CS, IT, Labour”
Employee Age between 18 and
Default value for department is “CS”.
Project( p_id, pName, p_bonus,duration_in_months, Eid)
Pid and Eid combine makes Primary
NOTE: Eid in project is foreign key from Employee. Write SQL Queries for the following statements.
Display whole address of employee along with name, columns names must be “Emp Name” and “Address”.
Display 30% top records of
Display names of all those employees whose address is “house number 44, street no 25, Rwp”.
Display data of employees who lives in “isb or rwp or khi or lhr”. (do not use or keyword)
Display those employees whose age is more than 18 and less than 33. (do not use relational operators “ > or <”.
Display all those employees whose name starts with “S” and…
arrow_forward
JAVA Script
Create a form that has a last name, a first name, an email address, a drop-down listing of 10 cities in Massachusetts. The list is to be sorted (hint: think array), an email address, and a zip code field. Each field is to be process through a validation function. You may use one function for each but if you are creative you should be able to use just one function. The drop-down listing will not require validation because they will be choosing from a list that you are providing.
This input from must be styled and show an effect to the user as to which field that are currently providing input.
arrow_forward
Correct answer will be appreciated.else downvoted.
arrow_forward
Java Programming Project
You need to make an exam application in the project. Write the project using JavaFX. Do
not use a database. Accordingly, what is requested must be performed:
1. Adding questions to the question bank: different types of questions should be
added to the question bank. Question types include multiple choice, True/False, fill-in,
and classic type questions. It is mandatory to use inheritance and polymorphism in
writing classes related to questions and in using their objects.
For example, a multiple choice question should have the following information:
a) question text
b) answer options such as a, b, c, d
c) answer
d) points
e) degree of difficulty (such as easy, normal and difficult)
In the upper classes, taking into account the content of other types of questions and
subclasses must be determined which properties should be.
2. Removing a question from a question bank: finding a question that will be deleted
first when removing a question from a question bank it…
arrow_forward
Mary and Jane have a joint authorship for their book "Cooking 101". Mary passed
away before Jane. The term for their copyright will end when? *
Lifetime of Mary + 50 years
Lifetime of Jane + 50 years
Lifetime of Jane + 25 years
50 years from the date of the publication of their book
arrow_forward
Task 2
Use function to calculate Computer Skills. Both skills Yes: show "Sufficient", only one skill: show "Good", no skill: show "Poor"
Computer Skills
Student ID
521370
811298
780223
528112
427747
207676
158877
154194
480967
962948
701444
803700
268544
975048
204401
846716
127466
621984
285594
826758
972888
739477
857713
339297
249521
868320
872950
198271
702356
726662
365857
136850
492928
850557
987114
776815
666031
904552
161237
268716
Software Skill
Yes
Yes
Yes
No
Yes
Yes
No
Yes
No
No
Yes
No
No
Yes
Yes
No
No
No
Yes
Yes
Yes
Yes
Yes
Yes
Yes
Yes
No
Yes
No
Yes
No
Yes
No
Yes
No
Yes
No
Yes
Yes
Yes
Hardware Skill
No
Yes
Yes
No
Yes
Yes
No
No
Yes
No
Yes
No
No
No
No
Yes
No
No
No
No
Yes
Yes
Yes
Yes
No
No
Yes
No
Yes
No
Yes
Yes
| No
Yes
Yes
Yes
No
Yes
Yes
Yes
arrow_forward
in a shop ,there are 10 employee and 20 kinds of goods,goods id between 1-20
EMPLOYEE id first name last name gender
10001, 'Tom', 'Brown', 'F'10002, 'Elizabeth', 'Tremblay', 'F'10003, 'Gladys', 'Julie', 'F10004, 'John', 'Taylor', 'M10005, 'Amelia', 'Smith'10006, 'Logan', 'Katherine'10007, 'Leo', 'Brown'10007, 'Lem', 'Thompson'10009, 'Tom' 'Smith'10010, 'Emma', 'Campbell'
------------
and I want to add a name library in it ,like this
how could i create a HTML file ,with will randomly create customers with these employee .
there is a start button on the page .
press "start "bottom ,and it will It will randomly match 10 items, customers, and goods, display goods id ,customername and gender ,employer name , id and gender .
how to do such a page ?
arrow_forward
Fill the write here portion please
<!-- Testimonials -->
<section id="testimonials">// write here<li data-target="#carouselExampleIndicators" data-slide-to="0" class="active"></li>//write here<li data-target="#carouselExampleIndicators" data-slide-to="2"></li></ol>
arrow_forward
In PYTHON
Print values from the Goal column only
Print values only for columns: Team, Yellow Cards and Red Cards
Find and print rows for teams that scored more than 6 goals
Select the teams whose name start with a G
Print values only for columns Team and Shooting Accuracy , and only for rows in which the team is either of the following: England, Italy and Spain
Find and print rows for teams that scored more than 6 goals and at least 4000 passes
Team
Goals
Shots on target
Shots off target
Shooting Accuracy
Goals-to-shots (perc)
Total shots (inc. Blocked)
Hit Woodwork
Penalty goals
Penalties not scored
Headed goals
Passes
Passes completed
Passing Accuracy
Touches
Crosses
Dribbles
Corners Taken
Tackles
Clearances
Interceptions
Clearances off line
Clean Sheets
Blocks
Goals conceded
Saves made
Saves-to-shots ratio
Fouls Won
Fouls Conceded
Offsides
Yellow Cards
Red Cards
Subs on
Subs off
Players Used
Croatia
4
13
12
0.5
0.16
32
0
0
0
2
1076
828
0.769
1706
60…
arrow_forward
The parameter represents converted station data for a single station.
is_app_only
The function should return True if and only if the given station requires the use of an app
because it does NOT have a kiosk. Recall that a station requires the use of an app if and only
("Station") -> bool
if the string that the constant NO_KIOSK refers to is part of its station name.
arrow_forward
COURSE
CRS_CODE
DEPT_CODE
CRS_DESCRIPTION
CRS_CREDIT
CLASS
CLASS_CODE
CRS_CODE
CLASS_SECTION
CLASS_TIME
CLASS ROOM
PROF_NUM
00
ENROLL
CLASS_CODE
STU_NUM
ENROLL_GRADE
STUDENT
STU_NUM
STU_LNAME
STU_FNAME
STU_INIT
STU_DOB
STU_HRS
STU_CLASS
STU_GPA
STU_TRANSFER
DEPT_CODE
STU_PHONE
PROF_NUM
Review the relational diagram above and briefly describe the following in one sentence.
1. How would you extend this database to identify the Professor who taught a class?
2. How would you extend this database to identify the Department that had a course?
arrow_forward
def buy_sell_stock_prices(stock_prices): current_buy = stock_prices[0] global_sell = stock_prices[1] global_profit = global_sell - current_buy
for i in range(1, len(stock_prices)): current_profit = stock_prices[i] - current_buy
if current_profit > global_profit: global_profit = current_profit global_sell = stock_prices[i]
if current_buy > stock_prices[i]: current_buy = stock_prices[i]
return global_sell - global_profit, global_sell
stock_prices_1 = [10,9,16,17,19,23]buy_sell_stock_prices(stock_prices_1)# (9, 23)
stock_prices_2 = [8, 6, 5, 4, 3, 2, 1]buy_sell_stock_prices(stock_prices_2)# (6, 5).
arrow_forward
account.json
"1000001": { "accountType": "Chequing", "accountBalance": 0 }, "1000002": { "accountType": "Savings", "accountBalance": 0 }, "1000011": { "accountType": "Chequing", "accountBalance": 0 }, "1000022": { "accountType": "Savings", "accountBalance": 0 }, "1000031": { "accountType": "Chequing", "accountBalance": 0 }, "1000032": { "accountType": "Savings", "accountBalance": 0 }, "1000051": { "accountType": "Chequing", "accountBalance": 13.699999999999989 }, "1000052": { "accountType": "Savings", "accountBalance": 0 }, "1000071": { "accountType": "Chequing", "accountBalance": 0 }, "1000081": { "accountType": "Savings", "accountBalance": 0 }, "1000091": { "accountType": "Chequing", "accountBalance": 0 }, "lastID": "1000091"}
-------
var express =…
arrow_forward
mysql> DESC bookauthor;
mysql> DESC author;
mysql> DESC book;
| Field
| Туре
| Field
| Туре
| Field
Тур
| int(11
| varchai
author_order | int(11
royaltyshare | decima:
author_id
isbn
| author_id | int(1:
| ssn
lastname
| firstname | varch:
| phone
| address
| city
| state
| zip
| varch:
| varch:
isbn
var
I name
| type
pub_id
I price
| advance
I ytd_sales
I pubdate
var
var
| varch:
| varch:
| varch:
| varch:
| varch:
| int
dec
| dec
int
mysql> DESC bookeditor;
| dat
| Field
| Туре
mysql> DESC editor;
mysql> DESC publisher;
editor_id | int(11)
| varchar(1
| Field
isbn
| Field
| Туре
editor_id
ssn
pub_id | int(11)
| varchar(40)
varchar(40)
varchar(20)
varchar(2)
lastname
name
firstname
address
| phone
editor_position
salary
| city
state
arrow_forward
QUESTION 6
An
is a variable defined within a class to store values:
O parameter
O attribute
O argument
O None of the above
Click Save and Submit to save and submit. Click Save All Answers to save all
arrow_forward
Lab conditions:
This lab exercise to be completed by the end of the class. No late submission will be accepted
Work as group of two students.
Submit Word document file on D2L
Make sure your following naming format as listed below:
Last name, First Name:
Last name, First Name:
Questions:
1. Research, discuss Explain the purpose of different personal computer (PC) hardware components.
Make sure to address all the aspect of the topic.
Partial list of opcodes:
2. Desktop Computer DIY. Suppose you decide to build a desktop by yourself and your budget is
around $1000 (without OS). Discuss with your team members and list all the parts and tools you have
to purchase with price. List the technical Details and explain what your desktop will be used for, such
as listen to music, word document, 3D design, software development, watch movie and so on.
3. Download and run CPU-Z. Paste your screenshots (technical details) below.
4. Challenge Question
Consider the hypothetical machine:
Instruction…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
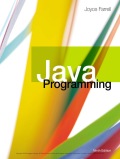
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
Related Questions
- CODE : def days_in_feb(user_year): is_leap_year = 29 not_leap_year = 28 if user_year % 4 ==0: return is_leap_year elif user_year % 4 ==0 and user_year % 100 == 0 and user_year % 400 == 0: return is_leap_year else: return(not_leap_year) if __name__ == '__main__': # Type your code here. Your code must call the function. user_year = int(input()) days = days_in_feb(user_year) print(user_year,'has', days, 'days in February.') ERROR OUTPUT IS NOT WORKING FOR YEAR 1900 Input 1712 Your output 1712 has 29 days in February. Your output days_in_feb(1913) correctly returned 28 days_in_feb(1600) Your output days_in_feb(1600) correctly returned 29 4: Unit testkeyboard_arrow_up 0 / 3 days_in_feb(1900) Your output days_in_feb(1900) incorrectly returned 29arrow_forward{ "name": "Renata Severson", "dob": "2017-02-22", "address": { }, "street": "4228 Lucy Circle", "town": "Ayr", "postode" : "PL01 3MG" "telephone": "+971-6808-178-469", "score": 10, "email": "brianna71@add.hn", "verified": false, "salary" : 50307 JSON Validation Valid: Yes If no, reason(s) XXarrow_forwardSome rows of the STUGRADE table of a school are shown below: STU_CODE CLS_CODE GRADE YEAR 291 111 3.1 2010 938 101B 2.3 2011 931 M101 3.3 2040 291 M101 3.4 2018 190 111 2.0 2021 931 321 3.9 2003 Suppose that STU_CODE is the student code (foreign key) and CLS_CODE is the class code (foreign key). Which of the following computes the average for each year of the student with code 931? a. SELECT AVG(GRADE) FROM STUGRADE WHERE STU_CODE = 931 GROUP BY YEAR b. SELECT AVG(GRADE) FROM STUGRADE WHERE STU_CODE = 931. c. SELECT AVG(GRADE) FROM STUGRADE GROUP BY STU_CODE = 931 d. SELECT AVG(GRADE) FROM STUGRADE WHERE STU_CODE = 931 GROUP BY AVG(GRADE)arrow_forward
- Whoever must play, cannot play def subtract_square(queries): Two players play a game of "Subtract a square", starting with a positive integer. On their turn to play, each player must subtract some square number (1, 4, 9, 16, 25, 36, 49, ...) from the current number so that the result does not become negative. Under the normal play convention of these games, the player who moves to zero wins, leaving his opponent stuck with no possible moves. (In the misère version of the game that has otherwise identical rules but where you win by losing the original game, these definitions would be adjusted accordingly.) This and many similar combinatorial games can be modelled with recursive equations. This game is an example of an impartial game since the moves available to both players are exactly the same, as opposed to “partisan games” such as chess where each player can only move pieces of one colour. A game state is therefore determined by the remaining number alone, but not by which player has…arrow_forward"song1": { "genre": "g1", "album": "a1", "rating": "r1", "other": "some data1" The songs.json }, "song2": { "genre": "g2", "album":"a2", "rating": "r2", "other": "some data2" }, "song3": { "genre": "g3", "album": "a3", "rating": "r3", "other": "some data3" }, "song4": { "genre": "g4", "album":"a4", "rating": "r4", "other": "some data4" } }arrow_forwardCreate an application that finds the greatest common divisor of two positive integers entered by the user. Console Greatest Common Divisor Finder Enter first number: 12 Enter second number: 8 Greatest common divisor: 4 Continue? (y/n): y Enter first number: 77 Enter second number: 33 Greatest common divisor: 11 Continue? (y/n): y Enter first number: 441 Enter second number: 252 Greatest common divisor: 63 Continue? (y/n): n Specifications The formula for finding the greatest common divisor of two positive integers x and y must follow the Euclidean algorithm as follows: Subtract x from y repeatedly until y < x. Swap the values of x and y. Repeat steps 1 and 2 until x = 0. y is the greatest common divisor of the two numbers. You can use one loop for step 1 of the algorithm nested within a second loop for step 3. Assume that the user will enter valid integers for both numbers. The application should continue only if the user enters 'y' or 'Y' to continue. Note that if you do…arrow_forward
- ✓ Exercises Exercise: Write a method _ and _ that computes the region representing the intersection of two regions. Exercise: Write a method _contains__, which checks whether a n-dimensional point belongs to the region. Remember, a point belongs to the region if it belongs to one of the rectangles in the region. ✓ Membership of a point in a region #@title Membership of a point in a region def region_contains (self, p): ### YOUR SOLUTION HERE Region._contains = region_contains [ ] # Tests 10 points. assert (2, 1) in Region (Rectangle((0, 2), (0, 3)), Rectangle((4, 6), (5,8))) assert (2, 1) not in Region (Rectangle((0, 1), (0, 3)), Rectangle((4, 6), (5, 8))) Exercise: Write a method _le_ for regions such that R <= S if the region R is contained in the region S. You can test this by checking that the difference between R and S is empty.arrow_forwardThe constants are: COLUMN_ID = 0COLUMN_NAME = 1COLUMN_HIGHWAY = 2COLUMN_LAT = 3COLUMN_LON = 4COLUMN_YEAR_BUILT = 5COLUMN_LAST_MAJOR_REHAB = 6COLUMN_LAST_MINOR_REHAB = 7COLUMN_NUM_SPANS = 8COLUMN_SPAN_DETAILS = 9COLUMN_DECK_LENGTH = 10COLUMN_LAST_INSPECTED = 11COLUMN_BCI = 12 INDEX_BCI_YEARS = 0INDEX_BCI_SCORES = 1MISSING_BCI = -1.0 EARTH_RADIUS = 6371 This is an example of one of the bridges which is a list. def create_example_bridge_1() -> list:"""Return a bridge in our list-format to use for doctest examples. This bridge is the same as the bridge from row three of the dataset.""" return [1, 'Highway 24 Underpass at Highway 403','403', 43.167233, -80.275567, '1965', '2014', '2009', 4,[12.0, 19.0, 21.0, 12.0], 65.0, '04/13/2012',[['2013', '2012', '2011', '2010', '2009', '2008', '2007','2006', '2005', '2004', '2003', '2002', '2001', '2000'],[MISSING_BCI, 72.3, MISSING_BCI, 69.5, MISSING_BCI, 70.0, MISSING_BCI,70.3, MISSING_BCI, 70.5, MISSING_BCI, 70.7, 72.9, MISSING_BCI]]] def…arrow_forwardHelp code.arrow_forward
- Employee ( Eid, Ename,EAge, ECity, E_Street, E_House#, E_Basic_Salary, E_Bonus,E_Rank, E_Department) Eid is Primary E_Rank can be “Manager, Developer, Admin” E_Department can be “HR, CS, IT, Labour” Employee Age between 18 and Default value for department is “CS”. Project( p_id, pName, p_bonus,duration_in_months, Eid) Pid and Eid combine makes Primary NOTE: Eid in project is foreign key from Employee. Write SQL Queries for the following statements. Display whole address of employee along with name, columns names must be “Emp Name” and “Address”. Display 30% top records of Display names of all those employees whose address is “house number 44, street no 25, Rwp”. Display data of employees who lives in “isb or rwp or khi or lhr”. (do not use or keyword) Display those employees whose age is more than 18 and less than 33. (do not use relational operators “ > or <”. Display all those employees whose name starts with “S” and…arrow_forwardJAVA Script Create a form that has a last name, a first name, an email address, a drop-down listing of 10 cities in Massachusetts. The list is to be sorted (hint: think array), an email address, and a zip code field. Each field is to be process through a validation function. You may use one function for each but if you are creative you should be able to use just one function. The drop-down listing will not require validation because they will be choosing from a list that you are providing. This input from must be styled and show an effect to the user as to which field that are currently providing input.arrow_forwardCorrect answer will be appreciated.else downvoted.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
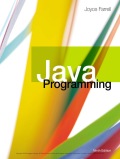
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage