Ch05 - Hw5-2
.docx
keyboard_arrow_up
School
California State University, Long Beach *
*We aren’t endorsed by this school
Course
340
Subject
Business
Date
May 10, 2024
Type
docx
Pages
3
Uploaded by DrFire6669 on coursehero.com
California State University, Long Beach
Information Systems College of Business Administration
IS 340 – Business Application Programming
Ch05 Functions
Professor: Jorge Echavarria
Week 05 Assignment
Chapter 05 (Functions)
Before you complete each of the following exercises, print this page
out and use it to keep notes about your progress. When you are done with each exercise, "check it off" of the list so you can keep track of your progress. Student Learning Outcomes:
1.
Students will implement different programs using each of the following: basic computation, simple I/O, conditional and iterative structures.
2.
Students will write programs that make use of function definitions, decomposition, parameter passing, and arrays.
3.
Students will write programs that implement programmer-defined types (classes or structures).
Submit the Assignment into “Assignment 5” Dropbox Folder. Attach it with the name LastnameFirstname_Assign5.docx (or .doc for Office 2003)
Don't forget to write your name and the chapter number and any relevant information on the top of
the document as well
The deadline
for all the assignments will be posted in Canvas
All Test Cases must be demonstrated in order to get full credit 1.
Write pseudocode/algorithm for a function that translates a telephone number with letters in it (such as 1-800-FLOWERS) into the actual phone number. Use the standard letters on a phone pad. Make sure your function has an input and a return result. Also, be specific on how the numbers are
translated in your algorithm (don’t use a “dictionary” or “map”. Use what we’ve learned: “if blocks”) 4 pts
IS 340: Ch05 Assignment
Page 1
California State University, Long Beach
Information Systems College of Business Administration
IS 340 – Business Application Programming
Ch05 Functions
Professor: Jorge Echavarria
2.
Write the following function and provide a program to test it (main and function in one .py) 5 pts
def repeat(string, n, delim)
that returns the string string
repeated n
times, separated by the string delim
. For example repeat(“ho”, 3, “,”) returns “ho, ho, ho”.
a)
Your code with comments
b)
A screenshot of the execution
3.
Write the following functions and provide a program to test them (main and all functions in one .py). 6 pts
A)
def allTheSame(x, y, z) (returning True if the arguments are all the same, otherwise False)
B)
def allDifferent(x, y, z) (returning True if the arguments are all different, otherwise False)
C)
def sorted(x, y, z) (returning True if the arguments are sorted in ascending, otherwise False)
Note
: Make sure your output has meaning (Don’t just print “True” or “False”)
a)
Your code with comments
b)
A screenshot of the execution
4.
Write the following function and provide a program to test it (main and function in one .py) 5 pts
Def countVowels(string)
that returns a count of all vowels in the string string
. Vowels are letters a, e, i, o, and u and their uppercase variants. Your main program should ask the user to input a string and then print the results.
Test Case:
Please enter a string: sentencE
The total number of vowels = 3
a)
Your code with comments
b)
A screenshot of the execution
5.
Write the following function and provide a program to test it (main and function in one .py) 5 pts
def readFloat(prompt)
that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Below is how you’re going to call the function:
salary = readFloat(“Please enter your salary:”)
percentageRaise = readFloat(“What percentage raise would you like?”)
print("The salary is", salary)
print("The raise percentage is", percentageRaise)
a)
Your code with comments
b)
A screenshot of the execution
IS 340: Ch05 Assignment
Page 2
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
You are alone at home and have to prepare a bread sandwich for yourself. The following preparation activities and time taken are given in the table below:
Task
Description
Predecessor
Time (Minutes)
A
Purchase of bread
-
20
B
Take cheese and apply on bread
A
5
C
Get onions from freezer
A
1
D
Fry onions with pepper
B,C
6
E
Purchase sauce for bread
-
15
F
Toast bread
B,C
4
G
Assemble bread and fried onions
F
2
H
Arrange in plat
G
1
Determine:
a. While purchasing sauce, you met a friend and spoke to him for 6 minutes. Did this cause any delay in the preparation?
arrow_forward
Student: Angelea Tingey
The following table details the tasks required for Indiana-based Frank Pianki Industries to manufacture a fully
portable industrial vacuum cleaner. The times in the table are in minutes. Demand forecasts indicate a need to
operate with a cycle time of 10 minutes.
Performance
Activity Activity Description Time (mins)
A Attach wheels to tub
B
Attach motor to lid
C
Attach battery pack
D
Attach safety cutoff
E
Attach filters
Attach lid to tub
(1) Yes
O No
F
G
H
I
Assemble
attachments
Function test
Final inspection
Packing
c) The goal is to have 100% efficiency. Is this possible? (1).
d) The theoretical minimum number of workstations =
number)
5.0
1.8
3.0
3.8
3.0
2.0
3.0
Instructor: Angela Johnson
Course: MGT-455-0500
4.0
2.0
2.0
Predecessors
B
с
B
A, E
a-b) One of the possible assignment of tasks to workstations is shown correctly in Fig. 2
The efficiency of the assembly line with 3 workstations =
decimal places)
D, F, G
H
I
% (enter your response rounded to two…
arrow_forward
Weighted-Average Method Arsenio Company manufactures a single product that goes throughtwo processes, mixing and cooking. The following data pertain to the mixing department for August:[LO 6-2, 6-5][LO 6-2, 6-3, 6-4]Work-in-Process Inventory, August 1Conversion: 80% complete 34,000 unitsWork-in-Process Inventory, August 31Conversion: 50% complete 30,000 unitsUnits started 66,000Units completed and transferred out ?CostsWork-in-Process Inventory, August 1Material X $ 64,800Material Y 88,100Conversion 119,880Costs added during AugustMaterial X 152,700Material Y 135,900Conversion 305,120Material X is added at the beginning of work in the mixing department. Material Y is also added in themixing department, but not until units are 60% complete with regard to conversion. Conversion costs areincurred uniformly during the process. The company uses the weighted-average cost method.Required1. Calculate the equivalent units of material X, material Y, and conversion for the mixing department.2.…
arrow_forward
8:22
A moodle.nct.edu.om
Question 2
Not yet answered
Marked out of 0.50
P Flag question
Human Resource Planning is a cyclical
process because
We plan only once and implement it
We plan multiple times based on
different objectives
the result of one plan becomes the basis
of the next plan
We do not plan for any future needs
Question 3
Not yet answered
Marked out of 0.50
P Flag question
II
arrow_forward
Jamison Day Consultants has been entrusted with thetask of evaluating a business plan that has been divided into foursections—marketing, finance, operations, and human resources.Chris, Steve, Juana, and Rebecca form the evaluation team. Eachof them has expertise in a certain field and tends to finish thatsection faster. The estimated times taken by each team memberfor each section have been outlined in the table below. Furtherinformation states that each of these individuals is paid $60/hour.a) Assign each member to a different section such that JamisonConsultants’s overall cost is minimized.b) What is the total cost of these assignments?Times Taken by Team Members for Different Sections (minutes)MARKETING FINANCE OPERATIONS HRChris 80 120 125 140Steve 20 115 145 160Juana 40 100 85 45Rebecca 65 35 25 75
arrow_forward
SCA-1.2 - SELF-EMPLOYMENT AND WAGE EMPLOYMENT
Activity time: 20 minutes Instruction to the student:
The students have to discuss and write the advantage of self-employment, comparing it with wage employment.
WAGE / SALARY EMPLOYMENT
SELF EMPLOYMENT/ OWN BUSINESS
arrow_forward
Can we identify a specific area of difficulty being tackled by the workflow management tool?
arrow_forward
Chris has been with Drake Manufacturing for five years and was recently promoted to the position of shift supervisor. He is still learning his new position, and it is important to him to be liked and respected. At the most recent operations review meeting, the director reported that the company’s financials are troublesome, and managers should be prepared for a major reorganization. Several jobs will be eliminated, but it had not yet determined which departments would be affected.
Questions
1.Should he give his employees “small doses” of the impending change?
arrow_forward
For tis week I will request from you to solve this mini case.
The CEO of the Baker Inc, wants to implement a new accounting system, to improve work flow through the company’s sales, cash receipts, accounts payable, and cash disbursement procedures. The CEO is, however, concerned about the potential disruption that the implementation will have on operations. In an announcement letter to Baker’s employees, the CEO stated that: “I have contracted Flybynight Consulting Group to do the needs analysis, system selection, and design work. The programming and implementation will be performed in-house using existing IT department staff. The development process will be unobtrusive to user departments because Flybynight knows what needs to be done. They will work independently, in the background, and will not disrupt departmental and internal audit work flow with time consuming interviews, surveys, and questionnaires. This promises to be an efficient process that will produce a system that will…
arrow_forward
Managed by Q case wage analysis: Cleaning operators and handymen are two of the most important jobs that Manged by Q employs since they are the face of the company. 2015 pay rates and bonuses are listed in the case for the markets of New York, San Francisco, and Chicago. Managed by Q, during this time, was assessing moving into Boston, Atlanta, and Washington DC.
Create a job description for each of these two roles (cleaning operators and handymen)
arrow_forward
Kiko Teddy Bear is a manufacturer of stuffed teddy bears. Kiko would like to be able to produce 40teddy bears per hour on its assembly line. The following information will assist in answering thequestions that follow:
Task Information for Kiko Teddy Bear
Work Element Task Description ImmediatePredecessor Task Time (inseconds)A Cut teddy bear pattern None 90B Sew teddy bear cloth A 75C Stuff teddy bear B 50D Glue on eyes C 20E Glue on nose C…
arrow_forward
Kiko Teddy Bear is a manufacturer of stuffed teddy bears. Kiko would like to be able to produce 40teddy bears per hour on its assembly line. The following information will assist in answering thequestions that follow:
Task Information for Kiko Teddy Bear
Work Element Task Description ImmediatePredecessor Task Time (inseconds)A Cut teddy bear pattern None 90B Sew teddy bear cloth A 75C Stuff teddy bear B 50D Glue on eyes C 20E Glue on nose C…
arrow_forward
Karina Nieto works for New Products Inc., and one of hermany tasks is assigning new workers to departments. Thecompany recently hired six new employees and would likeeach one to be assigned to a different department. The em-ployees have completed a two-month training session ineach of the six departments from which they received theevaluations shown below (higher numbers are better).Determine how the new employees should be assigned todepartments so that overall performance is maximized.
arrow_forward
Case: "What am I Doing Wrong?"
Susan Johnson, one of the supervisors in the business office, seemed always to be extra busy but never “caught up”. Frustrated with her never diminishing workload and tired of staying in hours of late nearly every day, she spent two evenings reading up on planning and priorities and decided to try to plan the next day's activities at the finish of each work day.
She came to the office the following Monday with her day planned out to the last minute. She had created a list of everything she needed to get done that day, including the following:
Complete an overdue report
Write two performance appraisals due that day
Develop the agenda for the following day’s staff meeting, and
Prepare for a new employee orientation.
It would be a tight fit for an 8-hour day, but she believes she could do it if she remained focused.
Susan got off to a good start by finishing the report earlier than she thought she would and turned her attention to the performance…
arrow_forward
Florida Southwestern State College has decided to implement a new registration system that will allow students to register online as well as in person. As an IT manager, you decide to set up a JAD session to help define the requirements for a new system. The FSW organization is fairly typical, with an administrative staff that includes a registrar, student support and service team, a business office, an IT group, and a number of academic departments. Using this information, you start work on a plan to carry out the JAD session. Who would you invite to the session, and why? What would be your agenda for the session, and what would take place at each stage of the session?
arrow_forward
Work measurement is concerned with determining the length of time it should take to complete the job. Job times are vital inputs for capacity planning, workforce planning, estimating labor costs, scheduling, budgeting, and designing incentive systems. Comment.
arrow_forward
An assembly task was broken down into four elements, and timed using the continuous timing method. The resulting data and performance ratings are provided below. Calculate the total standard time for this task.
Element
1
2
3
4
Watch Time
1.85
2.96
5.42
7.10
Observed Time
Rating
95
105
120
115
Normal Time
Total ST
Standard Time
arrow_forward
What is a workflow chart and how is it important for business planning purposes?
arrow_forward
Kiko Teddy Bear is a manufacturer of stuffed teddy bears. Kiko would like to be able to produce 40 teddy bears per hour on its assembly line. The following information will assist in answering the questions that follow:
Task Information for Kiko Teddy Bear
Work Element
Task Description
Immediate Predecessor
Task time (in seconds)
A
Cut teddy bear pattern
None
90
B
Sew teddy bear cloth
a
75
C
stuff teddy bear
b
50
D
glue on eyes
c
20
E
glue on nose
c
15
F
sew on mouth
c
35
G
attach manufacturer's label
b
15
H
Inspect and pack teddy bear
d,e,f,g
40
a. Draw a precedence diagram.
b. What cycle time would provide the desired output?
c. What is the maximum possible output of the line?
d. What is the theoretical minimum number of workstations using the cycle time computed in” b “ above?
e. Assign tasks to workstations using the cycle time in “b” above.
f. Calculate the efficiency and idle time percentage of the line.
arrow_forward
A company is setting up an assembly line to produce 90 units per hour. The table below identifies the work elements, times, and immediate predecessors.
Work Element
Time (Sec.)
Immediate Predecessor(s)
A
25
-
B
18
A
C
20
A
D
5
B, C
E
12
C
F
8
E
G
16
D, F
H
12
G
Use one of the heuristic decision rules described in Table below to balance the assembly line so that it will produce 90 units per hour. Clearly state the decision rule you use and complete the below table using the selected rule.
Decision Rule
Logic
Longest work element
Picking the candidate with the longest time to complete is an effort to fit in the most difficult elements first, leaving the ones with short times to “fill out” the station.
Shortest work element
This rule is the opposite of the longest work element rule because it gives preference in workstation assignments to those work elements that are quicker. It can be tried…
arrow_forward
Describe the idea behind the term "procedure." What makes a process different from a planned activity?
arrow_forward
Case Study Topic: The effective management of time is crucial for students success. What is the importance of effective time management to successful study? Explain your answers and reasoning.
arrow_forward
Topic: Managment Information system
Please read and answer the different parts
operational management in Customer Service department please answer accordingly
Insurance Ltd. accepts payments on various insurance policies from employees of businesses across the island. These businesses deduct payments from employees’ salaries and pay the insurance company a lump sum each month. This total amount deducted is shown on each employee’s payslip. Deductions are due at the end of each month and each participating business submits one payment for its employees to the insurance company via online payments through the bank. However, if payments were received after 11:00 a.m., the bank does not process them until the next business day. At the beginning of each month an administrative assistant at MGMT Insurance Ltd. downloads the deductions for each company and allocates payments to the various policies. These payments include the client’s unique number along with his/her relevant policy…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
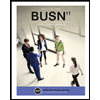
BUSN 11 Introduction to Business Student Edition
Business
ISBN:9781337407137
Author:Kelly
Publisher:Cengage Learning
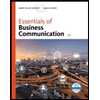
Essentials of Business Communication (MindTap Cou...
Business
ISBN:9781337386494
Author:Mary Ellen Guffey, Dana Loewy
Publisher:Cengage Learning
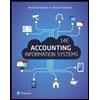
Accounting Information Systems (14th Edition)
Business
ISBN:9780134474021
Author:Marshall B. Romney, Paul J. Steinbart
Publisher:PEARSON
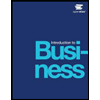
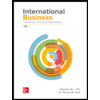
International Business: Competing in the Global M...
Business
ISBN:9781259929441
Author:Charles W. L. Hill Dr, G. Tomas M. Hult
Publisher:McGraw-Hill Education
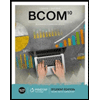
Related Questions
- You are alone at home and have to prepare a bread sandwich for yourself. The following preparation activities and time taken are given in the table below: Task Description Predecessor Time (Minutes) A Purchase of bread - 20 B Take cheese and apply on bread A 5 C Get onions from freezer A 1 D Fry onions with pepper B,C 6 E Purchase sauce for bread - 15 F Toast bread B,C 4 G Assemble bread and fried onions F 2 H Arrange in plat G 1 Determine: a. While purchasing sauce, you met a friend and spoke to him for 6 minutes. Did this cause any delay in the preparation?arrow_forwardStudent: Angelea Tingey The following table details the tasks required for Indiana-based Frank Pianki Industries to manufacture a fully portable industrial vacuum cleaner. The times in the table are in minutes. Demand forecasts indicate a need to operate with a cycle time of 10 minutes. Performance Activity Activity Description Time (mins) A Attach wheels to tub B Attach motor to lid C Attach battery pack D Attach safety cutoff E Attach filters Attach lid to tub (1) Yes O No F G H I Assemble attachments Function test Final inspection Packing c) The goal is to have 100% efficiency. Is this possible? (1). d) The theoretical minimum number of workstations = number) 5.0 1.8 3.0 3.8 3.0 2.0 3.0 Instructor: Angela Johnson Course: MGT-455-0500 4.0 2.0 2.0 Predecessors B с B A, E a-b) One of the possible assignment of tasks to workstations is shown correctly in Fig. 2 The efficiency of the assembly line with 3 workstations = decimal places) D, F, G H I % (enter your response rounded to two…arrow_forwardWeighted-Average Method Arsenio Company manufactures a single product that goes throughtwo processes, mixing and cooking. The following data pertain to the mixing department for August:[LO 6-2, 6-5][LO 6-2, 6-3, 6-4]Work-in-Process Inventory, August 1Conversion: 80% complete 34,000 unitsWork-in-Process Inventory, August 31Conversion: 50% complete 30,000 unitsUnits started 66,000Units completed and transferred out ?CostsWork-in-Process Inventory, August 1Material X $ 64,800Material Y 88,100Conversion 119,880Costs added during AugustMaterial X 152,700Material Y 135,900Conversion 305,120Material X is added at the beginning of work in the mixing department. Material Y is also added in themixing department, but not until units are 60% complete with regard to conversion. Conversion costs areincurred uniformly during the process. The company uses the weighted-average cost method.Required1. Calculate the equivalent units of material X, material Y, and conversion for the mixing department.2.…arrow_forward
- 8:22 A moodle.nct.edu.om Question 2 Not yet answered Marked out of 0.50 P Flag question Human Resource Planning is a cyclical process because We plan only once and implement it We plan multiple times based on different objectives the result of one plan becomes the basis of the next plan We do not plan for any future needs Question 3 Not yet answered Marked out of 0.50 P Flag question IIarrow_forwardJamison Day Consultants has been entrusted with thetask of evaluating a business plan that has been divided into foursections—marketing, finance, operations, and human resources.Chris, Steve, Juana, and Rebecca form the evaluation team. Eachof them has expertise in a certain field and tends to finish thatsection faster. The estimated times taken by each team memberfor each section have been outlined in the table below. Furtherinformation states that each of these individuals is paid $60/hour.a) Assign each member to a different section such that JamisonConsultants’s overall cost is minimized.b) What is the total cost of these assignments?Times Taken by Team Members for Different Sections (minutes)MARKETING FINANCE OPERATIONS HRChris 80 120 125 140Steve 20 115 145 160Juana 40 100 85 45Rebecca 65 35 25 75arrow_forwardSCA-1.2 - SELF-EMPLOYMENT AND WAGE EMPLOYMENT Activity time: 20 minutes Instruction to the student: The students have to discuss and write the advantage of self-employment, comparing it with wage employment. WAGE / SALARY EMPLOYMENT SELF EMPLOYMENT/ OWN BUSINESSarrow_forward
- Can we identify a specific area of difficulty being tackled by the workflow management tool?arrow_forwardChris has been with Drake Manufacturing for five years and was recently promoted to the position of shift supervisor. He is still learning his new position, and it is important to him to be liked and respected. At the most recent operations review meeting, the director reported that the company’s financials are troublesome, and managers should be prepared for a major reorganization. Several jobs will be eliminated, but it had not yet determined which departments would be affected. Questions 1.Should he give his employees “small doses” of the impending change?arrow_forwardFor tis week I will request from you to solve this mini case. The CEO of the Baker Inc, wants to implement a new accounting system, to improve work flow through the company’s sales, cash receipts, accounts payable, and cash disbursement procedures. The CEO is, however, concerned about the potential disruption that the implementation will have on operations. In an announcement letter to Baker’s employees, the CEO stated that: “I have contracted Flybynight Consulting Group to do the needs analysis, system selection, and design work. The programming and implementation will be performed in-house using existing IT department staff. The development process will be unobtrusive to user departments because Flybynight knows what needs to be done. They will work independently, in the background, and will not disrupt departmental and internal audit work flow with time consuming interviews, surveys, and questionnaires. This promises to be an efficient process that will produce a system that will…arrow_forward
- Managed by Q case wage analysis: Cleaning operators and handymen are two of the most important jobs that Manged by Q employs since they are the face of the company. 2015 pay rates and bonuses are listed in the case for the markets of New York, San Francisco, and Chicago. Managed by Q, during this time, was assessing moving into Boston, Atlanta, and Washington DC. Create a job description for each of these two roles (cleaning operators and handymen)arrow_forwardKiko Teddy Bear is a manufacturer of stuffed teddy bears. Kiko would like to be able to produce 40teddy bears per hour on its assembly line. The following information will assist in answering thequestions that follow: Task Information for Kiko Teddy Bear Work Element Task Description ImmediatePredecessor Task Time (inseconds)A Cut teddy bear pattern None 90B Sew teddy bear cloth A 75C Stuff teddy bear B 50D Glue on eyes C 20E Glue on nose C…arrow_forwardKiko Teddy Bear is a manufacturer of stuffed teddy bears. Kiko would like to be able to produce 40teddy bears per hour on its assembly line. The following information will assist in answering thequestions that follow: Task Information for Kiko Teddy Bear Work Element Task Description ImmediatePredecessor Task Time (inseconds)A Cut teddy bear pattern None 90B Sew teddy bear cloth A 75C Stuff teddy bear B 50D Glue on eyes C 20E Glue on nose C…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- BUSN 11 Introduction to Business Student EditionBusinessISBN:9781337407137Author:KellyPublisher:Cengage LearningEssentials of Business Communication (MindTap Cou...BusinessISBN:9781337386494Author:Mary Ellen Guffey, Dana LoewyPublisher:Cengage LearningAccounting Information Systems (14th Edition)BusinessISBN:9780134474021Author:Marshall B. Romney, Paul J. SteinbartPublisher:PEARSON
- International Business: Competing in the Global M...BusinessISBN:9781259929441Author:Charles W. L. Hill Dr, G. Tomas M. HultPublisher:McGraw-Hill Education
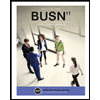
BUSN 11 Introduction to Business Student Edition
Business
ISBN:9781337407137
Author:Kelly
Publisher:Cengage Learning
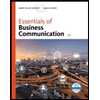
Essentials of Business Communication (MindTap Cou...
Business
ISBN:9781337386494
Author:Mary Ellen Guffey, Dana Loewy
Publisher:Cengage Learning
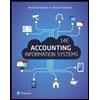
Accounting Information Systems (14th Edition)
Business
ISBN:9780134474021
Author:Marshall B. Romney, Paul J. Steinbart
Publisher:PEARSON
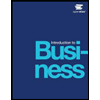
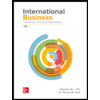
International Business: Competing in the Global M...
Business
ISBN:9781259929441
Author:Charles W. L. Hill Dr, G. Tomas M. Hult
Publisher:McGraw-Hill Education
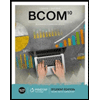