Write the definition of the class SwimmingPool, to implement the properties of a swimming pool. Your class should have the instance variables to atore the length(in feet), width (in feet), depth (in feet), the rate (in gallons per minute), at which the water is filling the pool, and the rate (in gallons per minute) at which the water is draining from the pool. And appropriate constructors to initialize the instance variables. Also, add member functions, to do the following: 1. Dtermine the amount of water needed to fill an empty pool or partially filled pool. 2. The time need to completely or partially fill the pool, or empty the pool; add water or drain for a specific amount of time. Here is my code that I have but not sure if it is correct. hank you:
Java User-Defined Classes
Write the definition of the class SwimmingPool, to implement the properties of a swimming pool. Your class should have the instance variables to atore the length(in feet), width (in feet), depth (in feet), the rate (in gallons per minute), at which the water is filling the pool, and the rate (in gallons per minute) at which the water is draining from the pool. And appropriate constructors to initialize the instance variables. Also, add member functions, to do the following:
1. Dtermine the amount of water needed to fill an empty pool or partially filled pool. 2. The time need to completely or partially fill the pool, or empty the pool; add water or drain for a specific amount of time.
Here is my code that I have but not sure if it is correct. hank you:
public static void main(String[] args) {
double poolLength;
double poolWidth;
double poolDepth;
double gallonsOfWater;
double shallowEnd;
double deepEnd;
// System.out.println("Please enter the dimension of the swimming pool: ");
// poolLength = console.nextDouble();
// poolWidth = console.nextDouble();
// shallowEnd = console.nextDouble();
//deepEnd = console.nextDouble();
// poolDepth = shallowEnd + deepEnd ;
//poolDepth = poolDepth / 2;
// gallonsOfWater = poolLength * poolWidth * poolDepth * GALLONS_PER_CUBIC_FOOT;
// System.out.println("The number of gallons of water in your pool is: " + gallonsOfWater);
SwimmingPool pool = new SwimmingPool(30, 15, 10);
pool.setRateToFillPool(20);
pool.setRateToDrainPool(19.5);
// System.out.println("The amount of water needed is " + pool.CalculateAmountOfWaterNeeded(20, 10, 4.5));
System.out.println("The amount of water needed is " + pool.getAmountOfWaterNeeded());
System.out.println("The time needed to fill your pool is " + pool.TimeToFillPool());
System.out.println("The time needed to drain your pool is " + pool.TimeToDrainPool());
}
}
//The SwimmingPool Class
public class SwimmingPool {
private final double GALLONS_PER_CUBIC_FOOT = 7.48;
private double lengthOfPool;
private double widthOfPool;
private double depthOfPool;
private double volumeOfPool;
private double capacityOfPool;
private double TimeToFillPool;
private double TimeToEmptyPool;
private double ratePerMinuteToFillPool;
private double ratePerMinuteToDrainPool;
SwimmingPool(){
lengthOfPool = 0.0;
widthOfPool = 0.0;
depthOfPool = 0.0;
}
SwimmingPool(double length, double width, double depth)
{
lengthOfPool = length;
widthOfPool = width;
depthOfPool = depth;
}
public void setLength(double length){
lengthOfPool = length;
}
public void setWidth(double width){
widthOfPool = width;}
public void setDepth(double depth){
depthOfPool = depth;
}
public void setPooldimensions(double length, double width, double depth){
lengthOfPool = length;
widthOfPool = width;
depthOfPool = depth;
}
public void setRateToFillPool(double rateToFill){
ratePerMinuteToFillPool = rateToFill;
}
public void setRateToDrainPool(double rateToDrain){
ratePerMinuteToFillPool = rateToDrain;
}
public double getRateToFillPool(){
return ratePerMinuteToFillPool;
}
public double getRateToDrainPool(){
return ratePerMinuteToFillPool;
}
//public void setPooldimensions(double length, double width, double depth1, double depth2){
// lengthOfPool = length;
// widthOfPool = width;
//depthOfPool1 = depth1;
//depthOfPool2 = depth2;
//}
private double CalculateAmountOfWaterNeeded(double length, double width, double depth)
{
volumeOfPool = (length * width * depth) * GALLONS_PER_CUBIC_FOOT;
return volumeOfPool;
}
public double getAmountOfWaterNeeded()
{ return CalculateAmountOfWaterNeeded(lengthOfPool, widthOfPool, depthOfPool);
//return volumeOfPool;--using this variable will return nothing because I
//didnot declare a wor,ing variable as a placeholder
}
// public double CalculateCapacityOfWateInCubicFoot(double length, double width, double depth1, depth2)
// {
// double totalDepth = (depth1 + depth2) / 2;
// volumeOfPool = (length * width * totalDepth) * 7.48;
//return volumeOfPool;
//}
public double TimeToFillPool(){
TimeToFillPool = volumeOfPool / (ratePerMinuteToFillPool * 60);
//System.out.println("the time to fill the pool is " + TimeToFillPool + "hours ");
return TimeToFillPool;
}
public double TimeToDrainPool(){
//setRateToDrainPool(ratePerMinuteToDrainPool);
TimeToEmptyPool = volumeOfPool / (ratePerMinuteToFillPool * 60);
return TimeToEmptyPool ;
}
public void addWater(){
}
public void drainWater(){
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

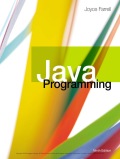
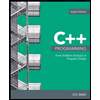
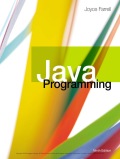
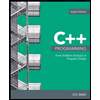
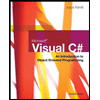