Write a program that calculates the area of some simple figures (i.e. a square and a rectangle). One function per figure. Check if the function arguments are greater than 0 – if they aren't, throw an exception. This program should use your own exceptions to communicate with a higher-level code. Add some attribute in your exception to pass a message to the user. Some boilerplate code is included below.
Write a program that calculates the area of some simple figures (i.e. a square and a rectangle). One function per figure. Check if the function arguments are greater than 0 – if they aren't, throw an exception. This program should use your own exceptions to communicate with a higher-level code. Add some attribute in your exception to pass a message to the user. Some boilerplate code is included below.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter14: Exception Handling
Section: Chapter Questions
Problem 4PE
Related questions
Question
pls help answer this question in c++

Transcribed Image Text:Lab 7.2.1 Exceptions: catch block
Objectives
Familiarize the student with:
• handling exceptions;
writing his/her own exception classes;
• passing additional information in exceptions;
Scenario
Write a program that calculates the area of some simple figures (i.e. a square and a rectangle). One function per figure. Check if the
function arguments are greater than 0 – if they aren't, throw an exception. This program should use your own exceptions to
communicate with a higher-level code. Add some attribute in your exception to pass a message to the user. Some boilerplate code is
included below.
#include <iostream>
using namespace std;
//add your own exception class here
//add functions code here
int main(void) {
float a, b, r;
cin >> a;
cin >> b;
try
{
square_area(a);
rectangle_area(a,b);
float rsquare
float rrectangle
cout <« rsquare << endl « rrectangle << endl;
}
//add a suitable catch block here
return 0;
Example input
2
1
Example output
4
Page 1 / 1
+
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
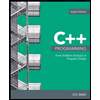
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
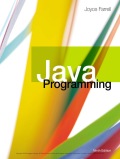
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
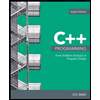
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
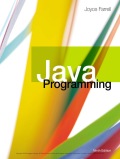
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT