Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator
class RetailPriceCalculator {
@staticmethod
def calculateRetail(wholesale_cost, markup_percentage):
if wholesale_cost < 0:
return 0.00
if markup_percentage < -100:
return 0.00
retail_price = wholesale_cost * (1 + markup_percentage / 100)
return retail_price
def main():
while True:
wholesale_cost = float(input("Please enter the wholesale cost or -1 to exit:\n"))
if wholesale_cost == -1:
break
while wholesale_cost < 0:
print("Wholesale cost cannot be a negative value.")
wholesale_cost = float(input("Please enter the wholesale cost again or -1 to exit:\n"))
markup_percentage = float(input("Please enter the markup percentage or -1 to exit:\n"))
if markup_percentage == -1:
break
while markup_percentage < -100:
print("Markup cannot be less than -100%.")
markup_percentage = float(input("Please enter the markup again or -1 to exit:\n"))
retail_price = RetailPriceCalculator.calculateRetail(wholesale_cost, markup_percentage)
print(f"The retail price is: {retail_price:.2f}")
if __name__ == "__main__":
main()
Test Case 1
10ENTER
Please enter the markup percentage or -1 exit:\n
-1ENTER
Test Case 2
100ENTER
Please enter the markup percentage or -1 exit:\n
100ENTER
The retail price is: 200.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 3
10ENTER
Please enter the markup percentage or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
100ENTER
The retail price is: 20.00\n
Please enter the wholesale cost or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-100ENTER
The retail price is: 0.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 4
-200ENTER
Wholesale cost cannot be a negative value.\n
Please enter the wholesale cost again or -1 exit:\n
10ENTER
Please enter the markup percentage or -1 exit:\n
-200ENTER
Markup cannot be less than -100%.\n
Please enter the markup again or -1 exit:\n
50ENTER
The retail price is: 15.00\n
Please enter the wholesale cost or -1 exit:\n
-1ENTER
Test Case 5
-1ENTER

Step by step
Solved in 4 steps with 2 images

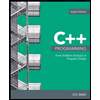
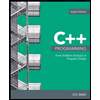