Write a Program for insertion and deletion from an array without replacement of element from the arrays. Also, write a program to search the array for the presence of a given element from an array. Write the sub-functions in the provided test case in text form and upload the same. #include #include int cmpfunc (const void * a, const void * b) { return ( *(int*)a - *(int*)b ); } int insert_function(int *data, int n, int p, int x) { /* input data: array, n: size of array, p: position of insertion, x: element to insert*/ /*output:-1 indication error or 0 if successful /* write the function for inserting element into a position*/ } int delete_function(int *data, int n, int p, int x) { /* input data: array, n: size of array, p: position of deletion, x: element to deletion*/ /*output:-1 indication error or 0 if successful /* write the function for delete element into a position*/ } int linear_search_function(int *data, int n, int x) { /*write the function to search an element in the array*/ /* input data: array, n: size of array, x: element to search*/ /*output: index of element or -1 if element is not present*/ int index=-1; // holds the position of the element /*add rest of the content of the program*/ return index; } int binary_search_function(int *data, int n, int x) { /*write the function to search an element in the array*/ int index=-1; // holds the position of the element /*add rest of the content of the program*/ return index; } int main() { int *data, i, condn=0, n, p, x, flag; char flagstr='Y'; data=(int*)calloc(10, sizeof(int)); // 10 signifies the maximum size of the array printf("Enter the size of array:"); scanf("%d", &n); printf("Enter the data element:\n"); for(i=0;i
Write a Program for insertion and deletion from an array without replacement of element from the arrays. Also, write a program to search the array for the presence of a given element from an array. Write the sub-functions in the provided test case in text form and upload the same.
#include<stdio.h> #include<stdlib.h> int cmpfunc (const void * a, const void * b) { return ( *(int*)a - *(int*)b ); } int insert_function(int *data, int n, int p, int x) { /* input data: array, n: size of array, p: position of insertion, x: element to insert*/ /*output:-1 indication error or 0 if successful /* write the function for inserting element into a position*/ } int delete_function(int *data, int n, int p, int x) { /* input data: array, n: size of array, p: position of deletion, x: element to deletion*/ /*output:-1 indication error or 0 if successful /* write the function for delete element into a position*/ } int linear_search_function(int *data, int n, int x) { /*write the function to search an element in the array*/ /* input data: array, n: size of array, x: element to search*/ /*output: index of element or -1 if element is not present*/ int index=-1; // holds the position of the element /*add rest of the content of the program*/ return index; } int binary_search_function(int *data, int n, int x) { /*write the function to search an element in the array*/ int index=-1; // holds the position of the element /*add rest of the content of the program*/ return index; } int main() { int *data, i, condn=0, n, p, x, flag; char flagstr='Y'; data=(int*)calloc(10, sizeof(int)); // 10 signifies the maximum size of the array printf("Enter the size of array:"); scanf("%d", &n); printf("Enter the data element:\n"); for(i=0;i<n;i++) { scanf("%d", &data[i]); } while(flagstr=='Y') { printf("Enter the operation choice:\n 1. insertion\n 2. deletion \n 3. linear Searching\n 4. Binary Searching\n"); scanf("%d", &condn); switch(condn) { case 1: printf("Enter the element to be inserted\n"); scanf("%d",&x); printf("Enter the position of insertion\n"); scanf("%d", &p); flag=insert_function(data, n, p, x); n++; if(flag==-1) printf("%d\n", flag); else { for(i=0;i<n;i++) { printf("%d\n", data[i]); } } break; case 2: printf("Enter the element to be deleted\n"); scanf("%d",&x); printf("Enter the position of deletion\n"); scanf("%d", &p); flag=delete_function(data, n, p, x); n--; if(flag==-1) printf("%d", flag); else { for(i=0;i<n;i++) { printf("%d\n", data[i]); } } break; case 3: printf("Enter the element to be searched\n"); scanf("%d",&x); flag=linear_search_function(data, n, x); printf("%d", flag); break; case 4: printf("Enter the element to be searched\n"); scanf("%d",&x); qsort(data, n, sizeof(int), cmpfunc); flag=binary_search_function(data, n, x); printf("%d\n", flag); break; default: printf("\n operation not selected\n"); } printf("\nDo you want to perform other opration (Y/N):"); scanf("%c\n", &flagstr); } return 0; }

Step by step
Solved in 3 steps

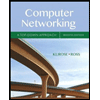
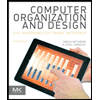
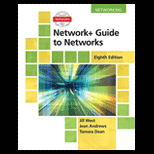
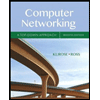
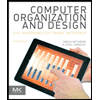
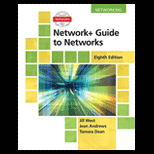
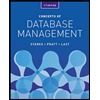
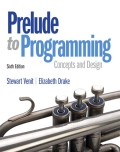
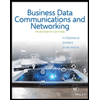