Write a function f which takes three pointers to int variables (a, b, c). Then it should: -Set the first variable to the sum of values of the variables (a + b + c). -Set the second variable to difference between maximum and minimum of values ( max(a,b,c) - min(a,b,c) ) -Set the third variable to median of a, b, c. For example: if a = 5, b = 10, c = 2 Then f(&a, &b, &c) should: Set a to 17 (that is the sum) Set b to 8 (that is max - min = 10 - 2) Set c to 5 (that is the median of 5, 10 and 2) NOTE: You are already provided a test main which checks your code for evaluation. DO NOT MODIFY IT or your answer will NOT be accepted. #include // Write your code here... ////////////////////////////////////////// // THIS IS THE TEST MAIN FOR YOUR PROGRAM // DO NOT MODIFY IT!! int main() { int a = 1; int b = 2; int c = 3; for (int i = 0; i < 2; i++) { f(&a, &b, &c); printf("%d\n", a); printf("%d\n", b); printf("%d\n", c); } return 0; } //////////////////////////////////////////
Write a function f which takes three pointers to int variables (a, b, c). Then it should:
-Set the first variable to the sum of values of the variables (a + b + c).
-Set the second variable to difference between maximum and minimum of values ( max(a,b,c) - min(a,b,c) )
-Set the third variable to median of a, b, c.
For example:
if a = 5, b = 10, c = 2
Then f(&a, &b, &c) should:
Set a to 17 (that is the sum)
Set b to 8 (that is max - min = 10 - 2)
Set c to 5 (that is the median of 5, 10 and 2)
NOTE: You are already provided a test main which checks your code for evaluation. DO NOT MODIFY IT or your answer will NOT be accepted.
#include<stdio.h>
// Write your code here...
//////////////////////////////////////////
// THIS IS THE TEST MAIN FOR YOUR
// DO NOT MODIFY IT!!
int main() {
int a = 1;
int b = 2;
int c = 3;
for (int i = 0; i < 2; i++) {
f(&a, &b, &c);
printf("%d\n", a);
printf("%d\n", b);
printf("%d\n", c);
}
return 0;
}
//////////////////////////////////////////
2)1) Define a struct to store fractions like a/b where a is numerator and b is denominator and a and b are integers.
Example: 1/2, 4/5, etc..
2) Write a function which takes two fractions as input parameters and returns the sum of these fractions which is another fraction.
For example:
If the input fractions are 1/2 and 2/5,
Then the function should return 9/10 as a fraction.
If the input fractions are 1/4 and 2/4,
Then the function should return 3/4 as a fraction.
In general:
a/b + c/d = (a + c) / b if b = d
a/b + c/d = (ad + bc) / (bd) otherwise
3) In main function:
- Take two fractions from the user. You need to get two integers from user for each fraction.
- Send two fractions to your function and get the result returned from the function.
- Print the result in a/b format.
#include<stdio.h>
// 1) Define a struct to store fractions like a/b
// where a is numerator and b is denominator
// and a and b are integers.
// Example: 1/2, 4/5, etc..
// ...
// 2) Write a function which takes two fractions
// and returns the sum of these fractions which
// is another fraction.
// For example:
// If the input fractions are 1/2 and 2/5,
// Then the function should return 9/10 as a fraction
// If the input fractions are 1/4 and 2/4,
// Then the function should return 3/4 as a fraction
// In general:
// a/b + c/d = (a + c) / b if b = d
// a/b + c/d = (ad + bc) / (bd) otherwise
// ...
// 3) In main function:
// - Take two fractions from user. You need to get
// two integers from user for each fraction
// - Send two fractions to your function and get
// the result returned from the function
// - Print the result in a/b format.
int main() {
// Complete main function
// ...
return 0;
}

Step by step
Solved in 2 steps

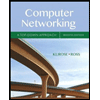
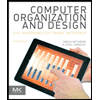
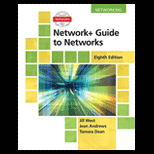
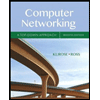
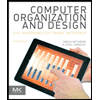
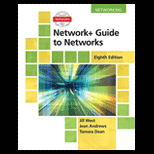
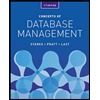
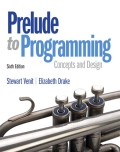
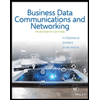