Write a function reverse which takes a string as an argument, reverses the string and returns a new string that is the reverse of the original. Note: you will need to malloc space for this new string! For example: Test char str[]="hello"; char *rts = reverse(str); printf("%s/%s", str,rts); Result hello/olleh
Write a function reverse which takes a string as an argument, reverses the string and returns a new string that is the reverse of the original. Note: you will need to malloc space for this new string! For example: Test char str[]="hello"; char *rts = reverse(str); printf("%s/%s", str,rts); Result hello/olleh
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
c
![Write a function reverse which takes a string as an argument, reverses the string and returns a new string that is the reverse of the original.
Note: you will need to malloc space for this new string!
For example:
Test
char str[]="hello";
char *rts reverse(str);
printf("%s/%s", str,rts);
Result
Answer: (penalty regime: 0 %)
1 #include <stdio.h>
2 #include <stdlib.h>
3 #include <string.h>
4
5
6
hello/olleh
char* reverse(char str[])
{
char *rts = (char*) malloc(strlen(str)* sizeof(char)); //allocating memory for pointer
7 char *start=rts; //starting address of pointer is stored in start
8 for(int i=strlen(str)-1;i>=0; i--) //traversing the str[] array from last to first
9-{
Check
10 *rts=str[i]; //storing the element of str[i] to rts
11
rts++; //incrementing pointer
12
}
13
return start; //rerturning starting address of the pointer
14
}
15
int main()
16 {
17 char str[100]="hello";
18 char *rts=reverse(str);
19 printf("%s/%s", str, rts);
20 return 0;
21}
Syntax Error(s)
_tester__.c:31:5: error: redefinition of 'main'
int main() {
ANNN
tester__.c:23:5: note: previous definition of 'main' was here
int main()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa1d592a5-ec96-4b1d-bddf-23e27014debe%2F55c7685d-86f6-4446-8b55-d1f4c145e51c%2Fkwbz4b3_processed.png&w=3840&q=75)
Transcribed Image Text:Write a function reverse which takes a string as an argument, reverses the string and returns a new string that is the reverse of the original.
Note: you will need to malloc space for this new string!
For example:
Test
char str[]="hello";
char *rts reverse(str);
printf("%s/%s", str,rts);
Result
Answer: (penalty regime: 0 %)
1 #include <stdio.h>
2 #include <stdlib.h>
3 #include <string.h>
4
5
6
hello/olleh
char* reverse(char str[])
{
char *rts = (char*) malloc(strlen(str)* sizeof(char)); //allocating memory for pointer
7 char *start=rts; //starting address of pointer is stored in start
8 for(int i=strlen(str)-1;i>=0; i--) //traversing the str[] array from last to first
9-{
Check
10 *rts=str[i]; //storing the element of str[i] to rts
11
rts++; //incrementing pointer
12
}
13
return start; //rerturning starting address of the pointer
14
}
15
int main()
16 {
17 char str[100]="hello";
18 char *rts=reverse(str);
19 printf("%s/%s", str, rts);
20 return 0;
21}
Syntax Error(s)
_tester__.c:31:5: error: redefinition of 'main'
int main() {
ANNN
tester__.c:23:5: note: previous definition of 'main' was here
int main()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
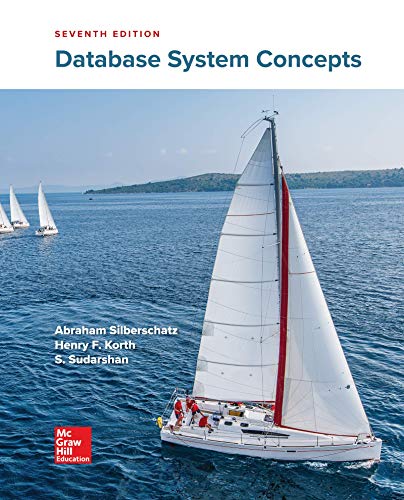
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
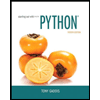
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
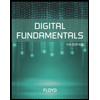
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
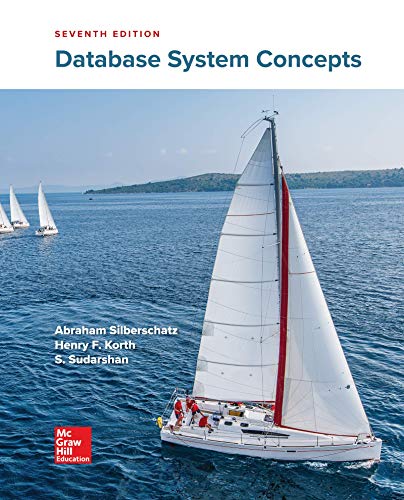
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
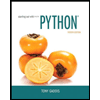
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
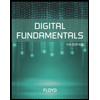
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
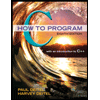
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
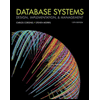
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
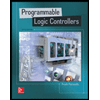
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education