Write a complete program that sorts dword unsigned integer array in descending order. Assume that the user doesn’t enter more than 40 integers. You MUST use the template-1-2.asm Download template-1-2.asm and follow all the directions there. Note: you have to review 3 peer assignments. You can’t add any more procedures to the template. The procedures can’t use any global variables (variables that are inside .data segment). The caller of any procedures sends its argument through the stack. Inside any procedures, if you need to use a register, you have to preserve its original value. You can't use uses, pushad operators. The callee is in charge of cleaning the stack Sample run: Enter up to 40 unsigned dword integers. To end the array, enter 0. After each element press enter: 1 4 3 8 99 76 34 5 2 17 0 Initial array: 1 4 3 8 99 76 34 5 2 17 Array sorted in descending order: 99 76 34 17 8 5 4 3 2 1 template-1-2.asm Title Assignment 7 COMMENT ! ***************** date: ***************** ! include irvine32.inc ; =============================================== .data ; Fill your data here ;================================================= .code main proc ; FILL YOUR CODE HERE ; YOU NEED TO CALL ENTER_ELEM, SORT_ARR AND PRINT_ARR PROCEDURES ; exit main endp ; ================================================ ; int enter_elem(arr_addr) ; ; Input: ; ARR_ADDRESS THROUGH THE STACK ; Output: ; ARR_LENGTH THROUGH THE STACK ; Operation: ; Fill the array and count the number of elements ; enter_elem proc ; FILL YOUR CODE HERE enter_elem endp ; ================================================ ; void print_arr(arr_addr,arr_len) ; ; Input: ; ? ; Output: ; ? ; Operation: ; print out the array ; print_arr proc ; FILL YOUR CODE HERE print_arr endp ; ================================================ ; void sort_arr(arr_addr,arr_len) ; ; Input: ; ? ; Output: ; ? ; Operation: ; sort the array ; sort_arr proc ; FILL YOUR CODE HERE ; YOU NEED TO CALL COMPARE_AND_SWAP PROCEDURE sort_arr endp ; =============================================== ; void compare_and_swap(x_addr,y_addr) ; ; Input: ; ? ; Output: ; ? ; Operation: ; compare and call SWAP ONLY IF Y < X ; compare_and_swap proc ; FILL YOUR CODE HERE ; YOU NEED TO CALL SWAP PROCEDURE compare_and_swap endp ; ================================================= ; void swap(x_addr,y_addr) ; ; Input: ; ? ; Output: ; ? ; Operation: ; swap the two inputs ; swap proc ; FILL YOUR CODE HERE swap endp end main
Write a complete program that sorts dword unsigned integer array in descending order. Assume that the user doesn’t enter more than 40 integers. You MUST use the template-1-2.asm Download template-1-2.asm and follow all the directions there.
Note: you have to review 3 peer assignments.
- You can’t add any more procedures to the template.
- The procedures can’t use any global variables (variables that are inside .data segment).
- The caller of any procedures sends its argument through the stack.
- Inside any procedures, if you need to use a register, you have to preserve its original value. You can't use uses, pushad operators.
- The callee is in charge of cleaning the stack
-
Sample run:
Enter up to 40 unsigned dword integers. To end the array, enter 0.
After each element press enter:
1
4
3
8
99
76
34
5
2
17
0
Initial array:
1 4 3 8 99 76 34 5 2 17
Array sorted in descending order:
99 76 34 17 8 5 4 3 2 1
template-1-2.asm
Title Assignment 7
COMMENT !
*****************
date:
*****************
!
include irvine32.inc
; ===============================================
.data
; Fill your data here
;=================================================
.code
main proc
; FILL YOUR CODE HERE
; YOU NEED TO CALL ENTER_ELEM, SORT_ARR AND PRINT_ARR PROCEDURES
;
exit
main endp
; ================================================
; int enter_elem(arr_addr)
;
; Input:
; ARR_ADDRESS THROUGH THE STACK
; Output:
; ARR_LENGTH THROUGH THE STACK
; Operation:
; Fill the array and count the number of elements
;
enter_elem proc
; FILL YOUR CODE HERE
enter_elem endp
; ================================================
; void print_arr(arr_addr,arr_len)
;
; Input:
; ?
; Output:
; ?
; Operation:
; print out the array
;
print_arr proc
; FILL YOUR CODE HERE
print_arr endp
; ================================================
; void sort_arr(arr_addr,arr_len)
;
; Input:
; ?
; Output:
; ?
; Operation:
; sort the array
;
sort_arr proc
; FILL YOUR CODE HERE
; YOU NEED TO CALL COMPARE_AND_SWAP PROCEDURE
sort_arr endp
; ===============================================
; void compare_and_swap(x_addr,y_addr)
;
; Input:
; ?
; Output:
; ?
; Operation:
; compare and call SWAP ONLY IF Y < X
;
compare_and_swap proc
; FILL YOUR CODE HERE
; YOU NEED TO CALL SWAP PROCEDURE
compare_and_swap endp
; =================================================
; void swap(x_addr,y_addr)
;
; Input:
; ?
; Output:
; ?
; Operation:
; swap the two inputs
;
swap proc
; FILL YOUR CODE HERE
swap endp
end main

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

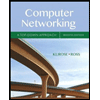
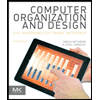
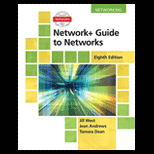
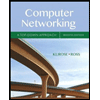
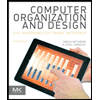
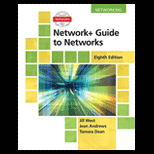
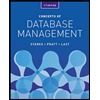
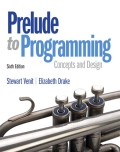
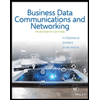