Write a C program to manage 50 bank accounts. The accounts are identified by numbers ranging from 100 to 149 as integer values. For instance, Account number 100 corresponds to the data stored in index 0, and account number 111 corresponds to the data stored in index 11. Therefore, when a user provides an account number, your program should include a function that returns the index where that account is stored. The program can handle various transactions and will be implemented as a menudriven program. You will use an array to store information for the 50 accounts. Initially, this array of accounts will be initialized to -1, where -1 signifies that the account is not yet open. The following table presents the questions that the program will ask the user. Each response to a transaction-type question should be a number. If the user provides an illegal number, the program should display an error message. All amounts are in dollars and can have up to 2 decimal places. User input Functions Transaction type?: 1 Account number?: account_number Initial deposit?: amount Open an account, giving the initial deposit. Allowed if less than 50 accounts now open and Amount should be greater or equal to 100 dollar. Prints the new account number. Transaction type?: 2 Account number?: account_number A Balance inquiry, prints the account number and the balance, only allowed if the account is open. Transaction type?: 3 Account number?: account_number Amount?: amount A Deposit, prints the account number and new balance, only allowed if account open. Transaction type?: 4 Account number?: account_number Amount?: amount A Withdrawal, only allowed if account open and sufficient funds available, prints account number and new balance. Transaction type?: 5 Account number?: account_number Close the account. Only allowed if account is open. Transaction type?: 6 Interest rate?: interest_rate Compute interest at given % rate. and apply to all accounts Use the interest formula below for your interest calculation balance += balance * interestRate / 100 Please utilize the provided code template as the official basis for your code and proceed to fill in the necessary code to complete the program. #include #define MAX_ACCOUNTS 50 // Function pointer for account operations void functionP(void (*p)(int, float), int* accountp, float *amountp){ p(*accountp, *amountp); } // arrays are decleared int accounts[MAX_ACCOUNTS]; float balances[MAX_ACCOUNTS]; int findAccount(int accountNumber) { for (int i = 0; i < MAX_ACCOUNTS; i++) { if (accounts[i] == accountNumber) { return i; } } return -1; } void openAccount(int accountNumber, float initialDeposit) { int index = findAccount(accountNumber); //Your Code Here } void balanceInquiry(int accountNumber, float amount) { int index = findAccount(accountNumber); //Your Code Here } void deposit(int accountNumber, float amount) { int index = findAccount(accountNumber); //Your Code Here } void withdrawal(int accountNumber, float amount) { int index = findAccount(accountNumber); //Your Code Here } void closeAccount(int accountNumber, float amount) { int index = findAccount(accountNumber); //Your Code Here } void computeInterest(float interestRate) { //Your Code Here } int main() { //Your Code Here to Initialize accounts and balances arrays int choice, accountNumber; float amount, interestRate; while (1) { printf("\nBank Account Management Menu:\n"); printf("1. Open an account\n"); printf("2. Balance inquiry\n"); printf("3. Deposit\n"); printf("4. Withdrawal\n"); printf("5. Close account\n"); printf("6. Compute interest\n"); printf("Enter your choice: "); scanf("%d", &choice); //Your Code Here } return 0;
Write a C program to manage 50 bank accounts. The accounts are identified by numbers
ranging from 100 to 149 as integer values. For instance, Account number 100
corresponds to the data stored in index 0, and account number 111 corresponds to the
data stored in index 11. Therefore, when a user provides an account number, your
program should include a function that returns the index where that account is stored.
The program can handle various transactions and will be implemented as a menudriven
program. You will use an array to store information for the 50 accounts. Initially, this
array of accounts will be initialized to -1, where -1 signifies that the account is not yet
open.
The following table presents the questions that the program will ask the user. Each
response to a transaction-type question should be a number. If the user provides an
illegal number, the program should display an error message. All amounts are in dollars
and can have up to 2 decimal places.
User input Functions
Transaction type?: 1
Account
number?: account_number
Initial deposit?: amount
Open an account, giving the initial deposit.
Allowed if less than 50 accounts now open and Amount
should be greater or equal to 100 dollar.
Prints the new account number.
Transaction type?: 2
Account
number?: account_number
A Balance inquiry, prints the account number
and the balance, only allowed if the account is open.
Transaction type?: 3
Account
number?: account_number
Amount?: amount
A Deposit, prints the account number
and new balance, only allowed if account open.
Transaction type?: 4
Account
number?: account_number
Amount?: amount
A Withdrawal, only allowed if account open
and sufficient funds available, prints
account number and new balance.
Transaction type?: 5
Account
number?: account_number
Close the account. Only allowed if account
is open.
Transaction type?: 6
Interest rate?: interest_rate
Compute interest at given % rate.
and apply to all accounts
Use the interest formula below for your interest calculation
balance += balance * interestRate / 100
Please utilize the provided code template as the official basis for your code and proceed to
fill in the necessary code to complete the program.
#include <stdio.h>
#define MAX_ACCOUNTS 50
// Function pointer for account operations
void functionP(void (*p)(int, float), int* accountp, float
*amountp){
p(*accountp, *amountp);
}
// arrays are decleared
int accounts[MAX_ACCOUNTS];
float balances[MAX_ACCOUNTS];
int findAccount(int accountNumber) {
for (int i = 0; i < MAX_ACCOUNTS; i++) {
if (accounts[i] == accountNumber) {
return i;
}
}
return -1;
}
void openAccount(int accountNumber, float initialDeposit) {
int index = findAccount(accountNumber);
//Your Code Here
}
void balanceInquiry(int accountNumber, float amount) {
int index = findAccount(accountNumber);
//Your Code Here
}
void deposit(int accountNumber, float amount) {
int index = findAccount(accountNumber);
//Your Code Here
}
void withdrawal(int accountNumber, float amount) {
int index = findAccount(accountNumber);
//Your Code Here
}
void closeAccount(int accountNumber, float amount) {
int index = findAccount(accountNumber);
//Your Code Here
}
void computeInterest(float interestRate) {
//Your Code Here
}
int main() {
//Your Code Here to Initialize accounts and balances arrays
int choice, accountNumber;
float amount, interestRate;
while (1) {
printf("\nBank Account Management Menu:\n");
printf("1. Open an account\n");
printf("2. Balance inquiry\n");
printf("3. Deposit\n");
printf("4. Withdrawal\n");
printf("5. Close account\n");
printf("6. Compute interest\n");
printf("Enter your choice: ");
scanf("%d", &choice);
//Your Code Here
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

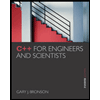
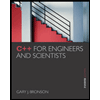